


Function rewriting and template programming are powerful techniques in C for enabling code extension and generalization. Function overriding enables extension by overriding base class methods in derived classes; template programming enables generalization by creating generic code that can be used in various types. A practical example demonstrates the use of function rewriting and template programming to calculate the area of a shape, showing the use of both techniques in extending and generalizing code.
Function rewriting and template programming: revealing the wonderful use of code expansion and code generalization
Function rewriting and template programming are powerful techniques in C programming , allowing developers to create scalable and versatile code.
Function overriding
Function overriding enables code extension by allowing alternative implementations of base class methods to be provided in derived classes. The syntax is as follows:
class Derived : public Base { public: // 重写基类方法 override double calculate() { // 自定义实现 ... } };
Template Programming
Template programming allows the creation of generic code that can be used for various types. The syntax is as follows:
template<typename T> class MyClass { T data; ... };
Practical case
Consider a program for calculating the areas of different shapes:
// 使用基类和函数重写 class Shape { public: virtual double calculateArea() = 0; }; class Square : public Shape { // 使用函数重写扩展基类 public: double side; Square(double side) : side(side) {} double calculateArea() override { return side * side; } }; class Circle : public Shape { // 再次使用函数重写扩展基类 public: double radius; Circle(double radius) : radius(radius) {} double calculateArea() override { return 3.14 * radius * radius; } }; int main() { Square s(5); Circle c(10); cout << "Square area: " << s.calculateArea() << endl; cout << "Circle area: " << c.calculateArea() << endl; }
// 使用模板编程 template<typename T> class Shape { T side; public: Shape(T side) : side(side) {} T calculateArea() { return side * side; } // 通用实现 }; // 使用模板实例化创建特定形状 class Square : public Shape<double> {}; class Circle : public Shape<double> {}; int main() { Square s(5); Circle c(10); cout << "Square area: " << s.calculateArea() << endl; cout << "Circle area: " << c.calculateArea() << endl; }
The above is the detailed content of Function Rewriting and Template Programming: Revealing the Magical Uses of Code Expansion and Code Generalization. For more information, please follow other related articles on the PHP Chinese website!
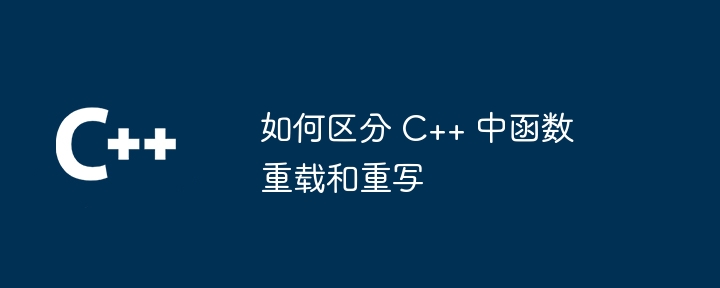
函数重载允许一个类中具有同名但签名不同的函数,而函数重写发生在派生类中,当它覆盖基类中具有相同签名的函数,提供不同的行为。
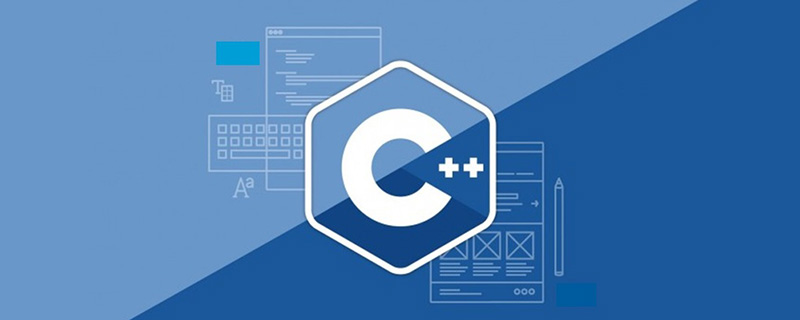
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
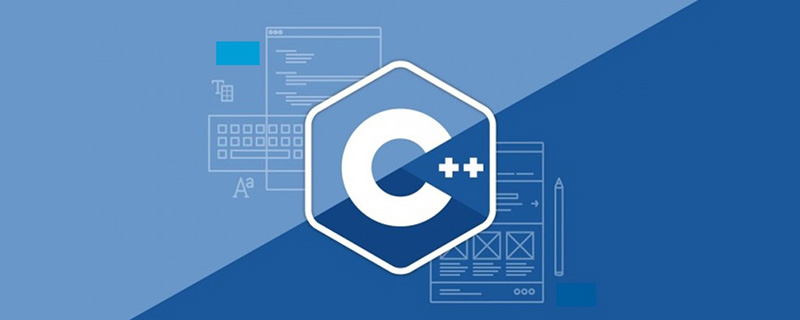
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
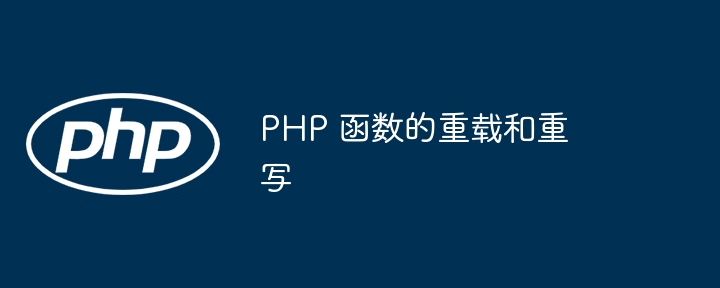
PHP中支持函数重载和重写,可创建灵活可重用的代码。函数重载:允许创建同名函数,但参数不同,根据参数匹配情况调用最合适的函数。函数重写:允许子类定义同名函数,覆盖父类方法,子类方法调用时将覆盖父类方法。
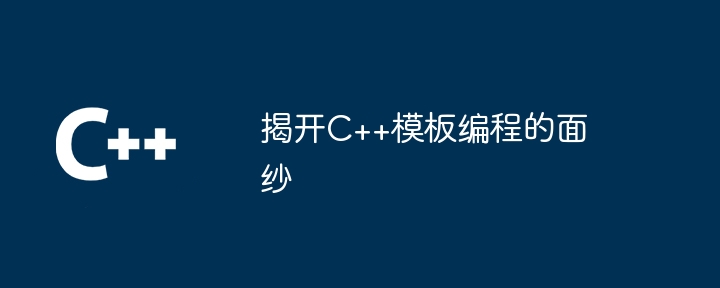
C++模板编程是一种使用参数化类或函数创建通用代码的技术,允许处理各种数据类型,提高代码可维护性和可扩展性。定义模板:使用template关键字指定模板参数,创建一个可通过不同类型实例化的类或函数。使用模板:在模板名前加上template关键字并指定参数类型,创建模板的具体实例。实战案例:使用模板进行二分查找,通过为模板参数传递不同的类型,可以在不同类型的排序数组上使用该函数。
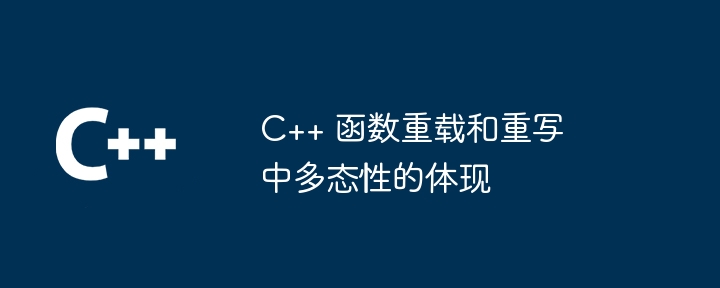
C++中的多态性:函数重载允许具有相同名称但不同参数列表的多个函数,根据调用时的参数类型选择执行的函数。函数重写允许派生类重新定义基类中已存在的方法,从而实现不同类型的行为,具体取决于对象的类型。
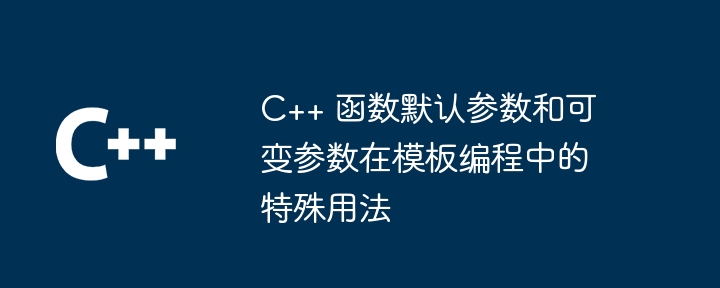
C++中针对默认参数和可变参数在模板编程中的特殊用法:默认参数允许函数在没有指定参数时使用默认值,从而实现函数重载的泛型化。可变参数允许函数接收任意数量的参数,实现了代码的通用性,可以用于处理任意数量的参数的函数或泛型化容器。实战案例:实现了一个通用的小数格式化函数,使用默认参数为不同类型的小数指定不同的精度。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
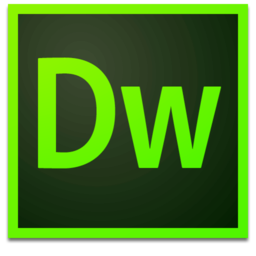
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
