


Performance optimization tips and experience summary of golang anonymous functions and closures
Although anonymous functions and closures are anonymous in Go, improper use will affect performance. To optimize closures, you can avoid unnecessary copies, reduce the number of captured variables, use the peephole optimizer and inlining, and finally benchmark the effectiveness.
Performance optimization tips and practical cases of Golang anonymous functions and closures
In Golang, anonymous functions and closures are Anonymous functions without explicit names. They can be used to create reusable, transitive blocks of code. However, if used incorrectly, they can also have a negative impact on program performance. The following are some tips and practical examples for optimizing the performance of anonymous functions and closures:
1. Avoid unnecessary copies
When a closure captures a value, it Create a copy of the value. This can incur significant overhead if the value is a large structure or slice. Consider using pointers or references to avoid unnecessary copies.
Case:
// 错误示范:拷贝切片 func badCopy(arr []int) func() []int { return func() []int { return arr // 返回切片副本 } } // 正确示范:使用指针 func goodCopy(arr []int) func() []int { return func() []int { return arr[:len(arr):len(arr)] // 返回切片指针 } }
2. Reduce the number of captured variables
The more variables a closure captures, the performance overhead The bigger it gets. The number of captured variables should be minimized and only the necessary variables should be captured.
Case:
// 错误示范:捕获过多变量 func badCapture(a, b, c, d int) func() int { return func() int { return a + b + c + d } } // 正确示范:仅捕获必要变量 func goodCapture(a, b, c int) func() int { d := 0 // 定义局部变量 return func() int { return a + b + c + d } }
3. Using peephole optimizer
peephole optimizer is a compiler optimization technology. Small code sequences can be identified and optimized. It can automatically optimize anonymous functions and closures that are unnecessary in certain situations.
Case:
The optimizer may optimize the following code:
func f(a int) { func() { _ = a }() // 使用匿名函数捕获 a }
The optimized code may become:
func f(a int) { _ = a // 直接使用 a }
4. Consider using inlining
Inlining is a compiler optimization technique that inserts function code directly into the location where it is called, thus eliminating the overhead of function calls. It can improve the performance of anonymous functions and closures, especially when they are small and called frequently.
Case:
inliner may optimize the following code:
func f() int { return 1 + 2 } func g() { for i := 0; i < 1000; i++ { _ = f() } }
After optimization, the code may become:
func g() { for i := 0; i < 1000; i++ { _ = 1 + 2 } }
5. Use benchmark testing
Benchmark testing is the best way to measure the performance of your code. By running your code under different circumstances and comparing the results, you can determine the effectiveness of a specific optimization technique.
Case:
func BenchmarkAnonFunc(b *testing.B) { for i := 0; i < b.N; i++ { f := func(a, b int) int { return a + b } _ = f(1, 2) } } func BenchmarkInlinedFunc(b *testing.B) { for i := 0; i < b.N; i++ { _ = func(a, b int) int { return a + b }(1, 2) } }
By comparing the results of these two benchmarks, you can determine whether it is worth changing the anonymous function to an inline function.
The above is the detailed content of Performance optimization tips and experience summary of golang anonymous functions and closures. For more information, please follow other related articles on the PHP Chinese website!
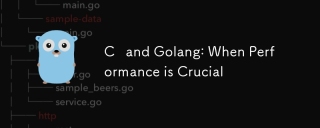
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
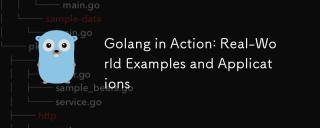
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
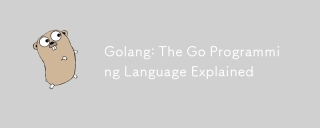
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
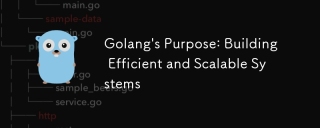
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
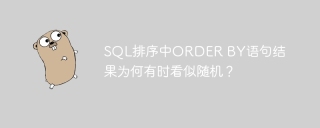
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
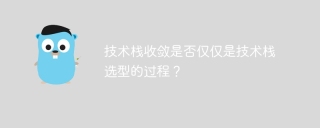
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
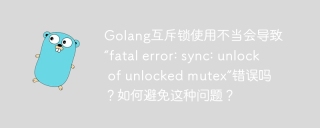
Golang ...
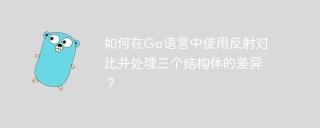
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
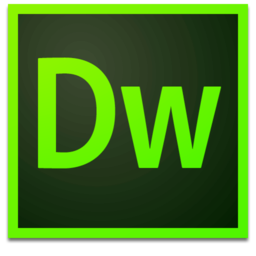
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.