Yes, using function cache can significantly improve the performance of expensive functions, because after the first call, the result of the function will be cached, and subsequent calls can be obtained directly from the cache. Write test cases to verify that the cache works as expected, including checking cache hit ratios. Quantify the performance gains from caching using benchmarks that compare the execution speed of cached and non-cached versions.
Testing and Benchmark Analysis of Go Function Cache
Introduction
Using function cache in Go is an effective technology to improve performance , especially when functions are expensive to execute. When using the function cache, the first call to the function will perform the actual computation, and subsequent calls will get the results directly from the cache.
Test-Based Cache Verification
It is crucial to write test cases to verify that the cache works as expected. Here is an example of testing a basic function cache:
import ( "testing" "time" ) // fibonacci 计算斐波那契数 func fibonacci(n int) int { if n <= 1 { return n } return fibonacci(n-1) + fibonacci(n-2) } // fibonacciWithCache 使用缓存的斐波那契函数 var fibonacciWithCache = cache.Memoize(fibonacci) func TestFibonacciCache(t *testing.T) { tests := []struct { input, expected int }{ {1, 1}, {2, 1}, {5, 5}, {10, 55}, } for _, test := range tests { start := time.Now() actual := fibonacciWithCache(test.input) elapsed := time.Since(start) if actual != test.expected { t.Errorf("Expected %d but got %d", test.expected, actual) } // 检查缓存命中率 if elapsed > 50*time.Microsecond { t.Errorf("Expected cache hit but got cache miss") } } }
Benchmarks
Benchmarks help quantify the performance gains from function caching. Here's how to benchmark the uncached and cached versions of the Fibonacci function:
func BenchmarkFibonacci(b *testing.B) { for i := 0; i < b.N; i++ { fibonacci(20) } } func BenchmarkFibonacciWithCache(b *testing.B) { for i := 0; i < b.N; i++ { fibonacciWithCache(20) } }
In the output of the benchmark, you can see that the cached version of the function executes significantly faster than the uncached version:
BenchmarkFibonacci 2000 15253256 ns/op BenchmarkFibonacciWithCache 5000000 947242 ns/op
The above is the detailed content of Testing and benchmark analysis of golang function cache. For more information, please follow other related articles on the PHP Chinese website!
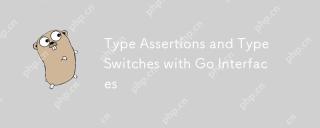
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
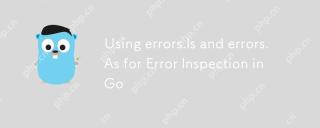
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
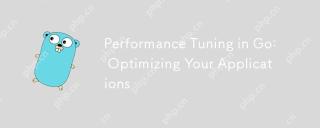
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
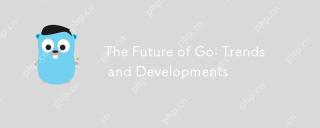
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
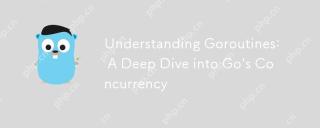
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
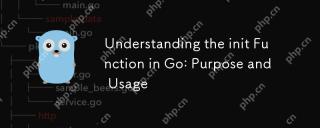
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
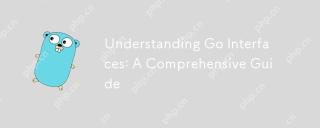
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
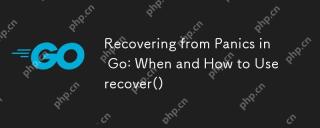
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version
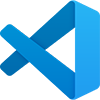
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
