


Overview of function inheritance: Function inheritance in C is implemented through the override keyword, allowing subclasses to override parent class functions, making polymorphism possible, that is, objects show different behaviors at runtime, even if they belong to the same parent class.
C Detailed explanation of function inheritance: The essence of polymorphism
In object-oriented programming, inheritance is an important mechanism, which allows subclasses to inherit from parent classes Data members and member functions. Function inheritance refers to the inheritance of member functions from parent class to child class.
Introduction to Polymorphism
Polymorphism is a key concept in object-oriented programming that allows objects to behave differently at runtime, even if they belong to the same parent class. Function inheritance is a way to achieve polymorphism.
Function inheritance
In C, function inheritance is implemented through the override
keyword. When a child class defines a function with the same name and signature as the parent class, the function is marked override
. This tells the compiler that the subclass is overriding the parent class's functions.
class Base { public: virtual void print() { std::cout << "Base class" << std::endl; } }; class Derived : public Base { public: virtual void print() override { std::cout << "Derived class" << std::endl; } };
In this example, the Base
class defines a print()
function, and the Derived
class passes override
keyword overrides this function. Therefore, when the print()
function of a Derived
class object is called, it will print "Derived class", not "Base class".
Virtual function
override
The function must be a virtual function. Virtual functions are declared with the virtual
keyword, which allows subclasses to override parent class functions. In the above example, the print()
function is virtual.
Practical case
The following is a code example that demonstrates function inheritance and polymorphism:
#include <iostream> class Shape { public: virtual double getArea() = 0; }; class Rectangle : public Shape { public: double width, height; Rectangle(double w, double h) : width(w), height(h) {} double getArea() override { return width * height; } }; class Circle : public Shape { public: double radius; Circle(double r) : radius(r) {} double getArea() override { return 3.14 * radius * radius; } }; int main() { Shape* shapes[] = {new Rectangle(2, 3), new Circle(4)}; for (int i = 0; i < 2; i++) { std::cout << "Area of " << (i == 0 ? "Rectangle" : "Circle") << ": " << shapes[i]->getArea() << std::endl; } return 0; }
In this case, Shape
is a shape class Base class, which defines a pure virtual function getArea()
. The Rectangle
and Circle
classes inherit from the Shape
class and override the getArea()
function to calculate their respective areas.
In the main()
function, using polymorphism, a base class array is used to store Rectangle
and Circle
objects. Since the getArea()
function is overridden, the correct area is printed based on the object type.
Through function inheritance and polymorphism, we can build flexible and extensible programs with different behaviors.
The above is the detailed content of Detailed explanation of C++ function inheritance: What is the essence of polymorphism?. For more information, please follow other related articles on the PHP Chinese website!
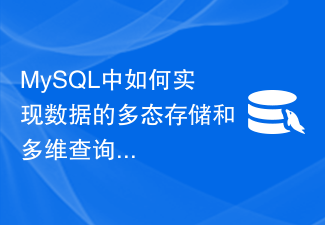
MySQL中如何实现数据的多态存储和多维查询?在实际应用开发中,数据的多态存储和多维查询是一个非常常见的需求。MySQL作为常用的关系型数据库管理系统,提供了多种实现多态存储和多维查询的方式。本文将介绍使用MySQL实现数据的多态存储和多维查询的方法,并提供相应的代码示例,帮助读者快速了解和使用。一、多态存储多态存储是指将不同类型的数据存储在同一个字段中的技
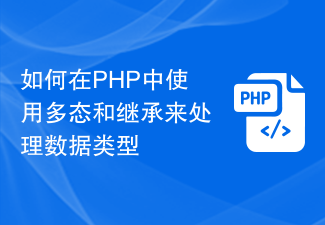
如何在PHP中使用多态和继承来处理数据类型引言:在PHP中,多态和继承是两个重要的面向对象编程(OOP)概念。通过使用多态和继承,我们可以更加灵活地处理不同的数据类型。本文将介绍如何在PHP中使用多态和继承来处理数据类型,并通过代码示例展示它们的实际应用。一、继承的基本概念继承是面向对象编程中的一种重要概念,它允许我们创建一个类,该类可以继承父类的属性和方法
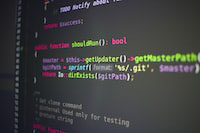
什么是面向对象编程?面向对象编程(OOP)是一种编程范式,它将现实世界中的实体抽象为类,并使用对象来表示这些实体。类定义了对象的属性和行为,而对象则实例化了类。OOP的主要优点在于它可以使代码更易于理解、维护和重用。OOP的基本概念OOP的主要概念包括类、对象、属性和方法。类是对象的蓝图,它定义了对象的属性和行为。对象是类的实例,它具有类的所有属性和行为。属性是对象的特征,它可以存储数据。方法是对象的函数,它可以对对象的数据进行操作。OOP的优点OOP的主要优点包括:可重用性:OOP可以使代码更
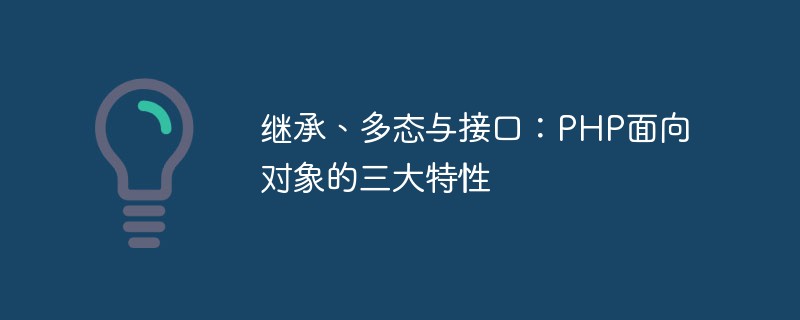
PHP是一种服务器端编程语言,自PHP5之后开始支持面向对象编程(OOP)。OOP的核心思想是将数据和行为封装在对象中,以提高程序的可维护性和可扩展性。在PHP中,面向对象编程具有三大特性:继承、多态与接口。一、继承继承是指一个类可以从另一个类中继承属性和方法。被继承的类称为父类或基类,继承的类称为子类或派生类。子类可以通过继承获得父类中的属性和方法,并且可
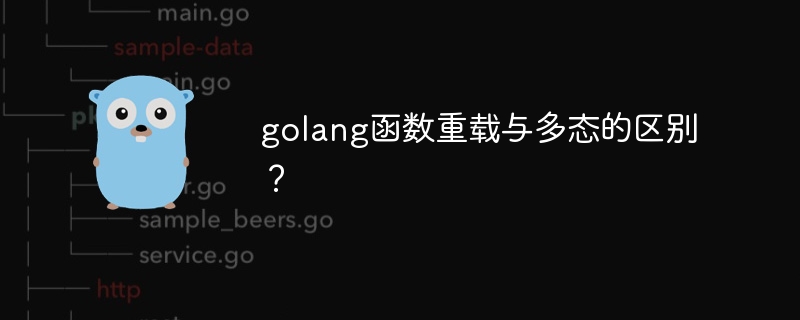
Go语言中不支持函数重载,因为它采用鸭子类型,根据实际类型确定值类型。而多态则通过接口类型和方法调用实现,不同类别的对象可以以相同方式响应。具体来说,Go语言中通过定义接口并实现这些方法,可以使不同类型的对象拥有相似行为,从而支持多态。
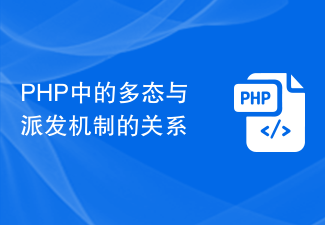
PHP中的多态与派发机制的关系在面向对象编程中,多态是一种强大的概念,它允许不同的对象对同一消息做出不同的响应。PHP作为一门强大的开发语言,也支持多态性,并且与之紧密相关的是派发机制。本文将通过代码示例来探讨PHP中的多态与派发机制的关系。首先,我们来了解一下什么是多态。多态是指对象能够根据自己的实际类型来调用相应的方法。通过使用多态,程序可以根据具体对象
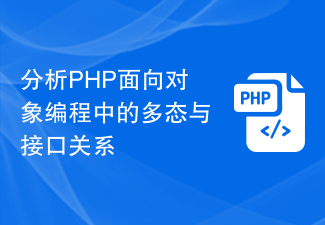
PHP面向对象编程中的多态与接口关系在PHP面向对象编程中,多态(Polymorphism)是一种重要的概念,它使得不同类的对象可以以一种统一的方式被使用。多态通过接口(Interface)的实现来实现,本文将通过代码示例来分析PHP面向对象编程中的多态与接口关系。在PHP中,接口是一种定义了一组方法的抽象结构,类通过实现接口来表达自己具有某些行为能力。接口
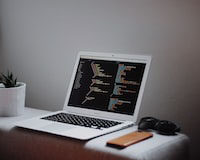
在python中,继承和多态是面向对象编程(OOP)中强大的概念,它们使代码更具可扩展性、可重用性和可维护性。本文将深入探讨Python中的继承和多态,揭开它们的神秘面纱并展示它们的强大功能。继承继承允许一个类(子类)从另一个类(父类)继承属性和方法。通过继承,子类可以重用父类中已经定义的代码,从而减少重复和提高代码可维护性。语法:classSubclass(Superclass):#子类独有的属性和方法演示代码:classAnimal:def__init__(self,name):self.n


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
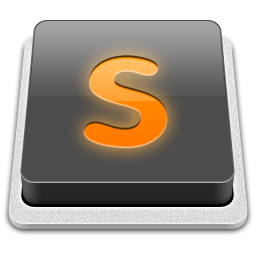
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
