


Best practices for PHP functions: integrating with external services?
Best practices for secure and efficient integration with external services include: 1) using HTTP client libraries; 2) handling errors; 3) caching responses; 4) using asynchronous calls; 5) enabling authentication and security. These practices optimize integration with external services in PHP by simplifying interactions, improving performance, and ensuring security. In a practical case, Guzzle is used to integrate with GitHub API to demonstrate the application of these best practices.
Best Practices for PHP Functions: Integrating with External Services
Integrating with external services is a common task in PHP, but Ensuring that the integration is secure and efficient is critical. Here are some best practices:
1. Use an HTTP client library
Using a specialized HTTP client library, such as Guzzle or PHP HTTP/Client, can simplify Interaction with external services. These libraries provide built-in support for common HTTP tasks, such as making requests, handling responses, and managing cookies.
Code sample:
use GuzzleHttp\Client; $client = new Client(); $response = $client->get('https://example.com/api/v1/users');
2. Handling errors
Various errors may occur when communicating with external services, handle These mistakes are critical. Use try-catch blocks or the exception handling mechanism provided by Guzzle to catch and handle errors.
Code example:
try { $response = $client->get('https://example.com/api/v1/users'); } catch (Exception $e) { // 处理错误 }
3. Caching responses
For frequently accessed endpoints, caching responses can improve performance. Guzzle provides a CachePool adapter that can be integrated with PSR-6 compliant caching systems such as Redis.
Code example:
use GuzzleHttp\Psr7\Cache; $cacheAdapter = new \Doctrine\Common\Cache\FilesystemCache(); $cachePool = new Cache($cacheAdapter); $client->getConfig('handler')->push(GuzzleHttp\Middleware::cache($cachePool));
4. Use asynchronous calls
If communication with external services is time-sensitive, use asynchronous calls Can improve application responsiveness. Guzzle provides a coroutine support package for performing non-blocking HTTP requests using coroutines.
Code Example:
use GuzzleHttp\Client; use GuzzleHttp\Psr7\Response; use Amp\Promise; $client = new Client(); Promise\async(function() { $response = yield $client->requestAsync('GET', 'https://example.com/api/v1/users'); // 处理响应... });
5. Authentication and Security
Use appropriate An authentication mechanism (such as Basic Authentication, OAuth, or JWT) authenticates the request. Consider using HTTP headers or sending authentication information as parameters.
Code Example:
$client = new Client([ 'auth' => ['user', 'password'], 'base_uri' => 'https://example.com/api/v1', ]);
Practice Example
Use Guzzle to integrate with the GitHub API to retrieve information about a specific repository :
Code example:
use GuzzleHttp\Client; $client = new Client([ 'base_uri' => 'https://api.github.com', 'headers' => [ 'Accept' => 'application/vnd.github.v3+json', 'Authorization' => 'Bearer ' . $_ENV['GITHUB_TOKEN'], ], ]); $response = $client->get('/repos/guzzle/guzzle'); $repo = json_decode($response->getBody()); echo $repo->name . ' has ' . $repo->subscribers_count . ' subscribers.';
The above is the detailed content of Best practices for PHP functions: integrating with external services?. For more information, please follow other related articles on the PHP Chinese website!
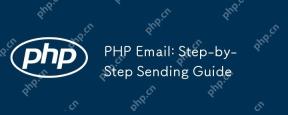
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
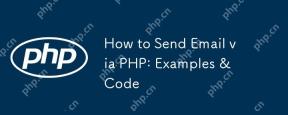
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
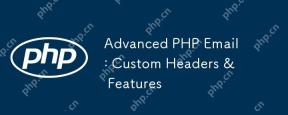
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
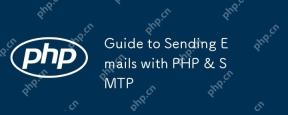
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
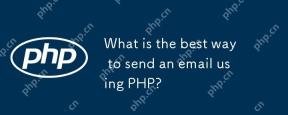
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
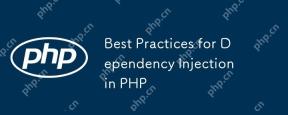
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
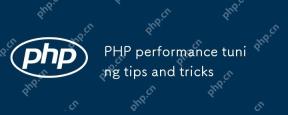
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
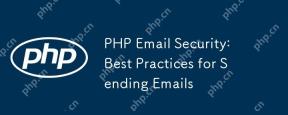
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
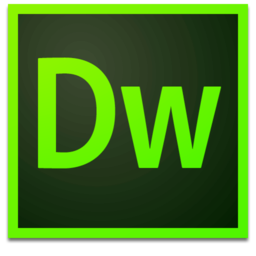
Dreamweaver Mac version
Visual web development tools
