Golang function communication pipeline timeout processing strategy
When using pipelines for communication, in order to prevent the pipeline receiver from being blocked all the time, Golang provides two timeout processing strategies: use Context to set time limits or use select to listen to multiple pipelines. When the pipeline receiver does not receive data, these two All strategies will time out.
Golang function communication pipeline timeout processing strategy
Pipelines are a common way of inter-process communication in Golang. However, when the receiving end of the pipeline cannot receive data, it will block forever. To prevent this blocking, we can use a piped receive operation with a timeout.
Timeout processing strategy
There are two main timeout processing strategies:
- Use Context:Use context .Context type, we can set a time limit. If no data is received within this time limit, the operation will time out.
- Use select: Using the select statement, we can listen to multiple pipes at the same time. When the pipeline receiver does not receive data, select will time out.
Practical case
The following is an example of a pipeline receiving operation using the Context timeout processing strategy:
package main import ( "context" "fmt" "log" "sync/atomic" "time" ) func main() { // 创建一个管道 ch := make(chan int) ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() // 并发地将数据发送到管道 go func() { for i := 0; i < 10; i++ { ch <- i } }() // 使用 Context 超时接收数据 go func() { var total uint64 for { select { case <-ctx.Done(): fmt.Println("Timeout reached!") return case value := <-ch: total += uint64(value) } } }() log.Printf("Total: %d", total) }
Using the select timeout processing strategy Pipe receive operation example:
package main import ( "fmt" "log" "sync/atomic" "time" ) func main() { // 创建一个管道 ch := make(chan int) // 创建一个 select 语句来监听管道和超时 var total uint64 go func() { for { select { case value := <-ch: total += uint64(value) case <-time.After(5 * time.Second): fmt.Println("Timeout reached!") return } } }() // 并发地将数据发送到管道 go func() { for i := 0; i < 10; i++ { ch <- i } }() log.Printf("Total: %d", total) }
The above is the detailed content of Golang function communication pipeline timeout processing strategy. For more information, please follow other related articles on the PHP Chinese website!
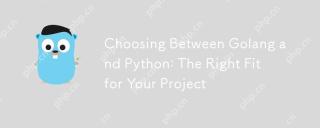
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
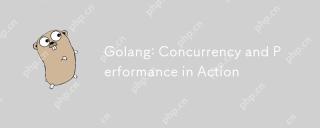
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
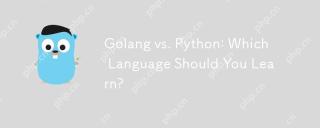
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
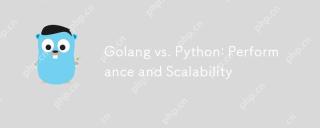
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
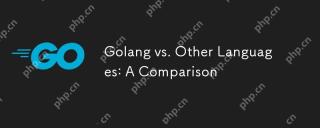
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
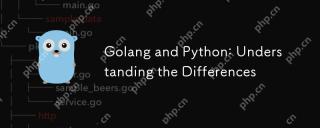
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
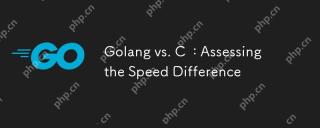
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
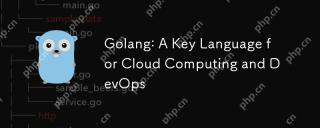
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
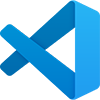
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
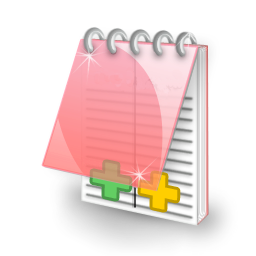
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use