Special use cases and tricks for generics in Go Use empty type interfaces for dynamic type checking to check runtime types. Use generic type parameters in collections to create containers of diverse types. Implement generic methods to perform common operations for different types of parameters. Use type constraints to implement type-specific generics and customize operations for specified types.
Special use cases and techniques of generics in Go
Generics introduce novel features that make writing flexible and efficient Code becomes possible. This article will explore special use cases and techniques for generics in Go.
1. Use empty type interface for dynamic type checking
any
type can represent any type. This allows us to perform dynamic type checking based on types determined at runtime.
func isString(v any) bool { _, ok := v.(string) return ok } func main() { x := "hello" y := 10 fmt.Println(isString(x)) // true fmt.Println(isString(y)) // false }
2. Using generic types on collections
Generic type parameters can be used in collection types to create a container of diverse types.
type Stack[T any] []T func (s *Stack[T]) Push(v T) { *s = append(*s, v) } func (s *Stack[T]) Pop() T { if s.IsEmpty() { panic("stack is empty") } v := (*s)[len(*s)-1] *s = (*s)[:len(*s)-1] return v } func main() { s := new(Stack[int]) s.Push(10) s.Push(20) fmt.Println(s.Pop()) // 20 fmt.Println(s.Pop()) // 10 }
3. Implement generic methods
Generic methods allow us to implement common operations for different types of parameters.
type Num[T numeric] struct { V T } func (n *Num[T]) Add(other *Num[T]) { n.V += other.V } func main() { n1 := Num[int]{V: 10} n2 := Num[int]{V: 20} n1.Add(&n2) fmt.Println(n1.V) // 30 // 可以使用其他数字类型 n3 := Num[float64]{V: 3.14} n4 := Num[float64]{V: 2.71} n3.Add(&n4) fmt.Println(n3.V) // 5.85 }
4. Use type constraints to implement type-specific generics
Type constraints limit the scope of generic types. It allows us to implement custom operations for specific types.
type Comparer[T comparable] interface { CompareTo(T) int } type IntComparer struct { V int } func (c *IntComparer) CompareTo(other IntComparer) int { return c.V - other.V } // IntSlice 实现 Comparer[IntComparer] 接口 type IntSlice []IntComparer func (s IntSlice) Len() int { return len(s) } func (s IntSlice) Less(i, j int) bool { return s[i].CompareTo(s[j]) < 0 } func (s IntSlice) Swap(i, j int) { s[i], s[j] = s[j], s[i] } func main() { s := IntSlice{{10}, {20}, {5}} sort.Sort(s) fmt.Println(s) // [{5} {10} {20}] }
These special use cases and techniques demonstrate the power of generics in Go, allowing the creation of more general, flexible, and efficient code.
The above is the detailed content of Special use cases and techniques of generics in golang. For more information, please follow other related articles on the PHP Chinese website!
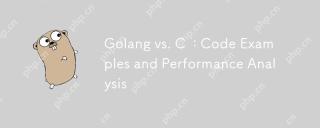
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
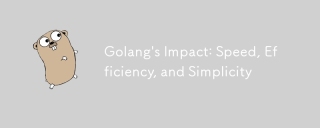
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
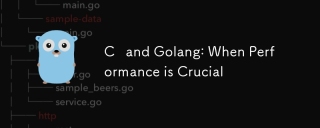
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
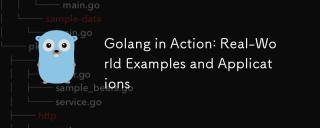
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
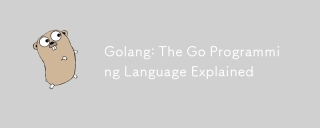
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
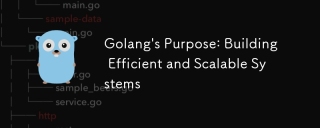
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
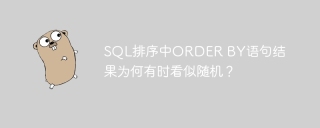
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
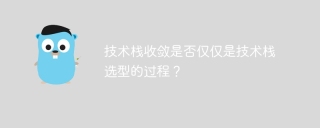
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
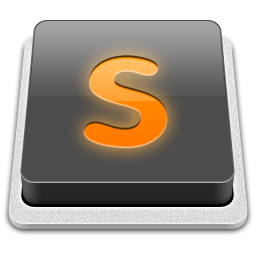
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
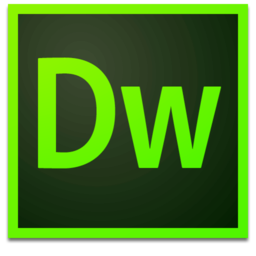
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool