Best practices for functions in Go include: Using meaningful function names Limiting the number of arguments Writing documentation comments using named return types Extracting common logic into separate functions Using closures to encapsulate state Provide interfaces Writing unit tests, coverage Different scenarios and using mocks and stub
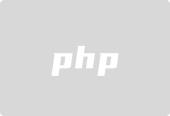
Function best practices in Go language object-oriented programming
In Go language object-oriented programming (OOP) , the function plays a crucial role. It is crucial to follow best practices to ensure code maintainability, scalability, and reusability.
Good Function Design
-
Choose meaningful names: Function names should clearly express their purpose and make it easier for other developers to understand.
-
Limit the number of parameters: Too many parameters will reduce the readability and maintainability of the function. Try not to exceed 4-5 parameters.
-
Use named return types: Explicitly specifying the type returned by a function helps improve code readability and prevent errors.
-
Write documentation comments: Add documentation comments before the function definition to explain the function, parameters and return values.
Reuse and scalability
-
Extract common logic to separate functions: Avoid writing the same code repeatedly, extract shared logic to Reusable functions.
-
Use closures to encapsulate state: Closures can be used to create functions with private state, helping to keep data encapsulated and modular.
-
Provide interfaces: Defining interfaces allows functions to accept different types of data and enhances the flexibility of the code.
Unit Testing
-
Writing Unit Tests: Write unit tests for each function to ensure it works as expected.
-
Cover different scenarios: Test various input and output scenarios to maximize coverage.
-
Use mocks and stubs: Use mocks and stubs to simulate dependencies and isolate functions for testing.
Practical case
Function to calculate the area of a circle:
import "math"
// CircleArea 计算给定半径的圆的面积。
func CircleArea(radius float64) float64 {
return math.Pi * radius * radius
}
Calculating the area of a polygon using reusable functions:
import "math"
// PolygonArea 计算多边形面积。
func PolygonArea(vertices [][]float64) float64 {
area := 0.0
for i := 0; i < len(vertices); i++ {
curX, curY := vertices[i][0], vertices[i][1]
nextX, nextY := vertices[(i+1)%len(vertices)][0], vertices[(i+1)%len(vertices)][1]
area += (curX * nextY - curY * nextX)
}
return math.Abs(area) / 2.0
}
The above is the detailed content of Golang functions best practices in object-oriented programming. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn