Common errors and solutions to generics in golang
Improper use of generics in Go can lead to errors: pointers to type parameters cannot be dereferenced, concrete type pointers should be used. Generic types cannot be compared with non-generic values and should be compared using reflection. Misuse of empty interfaces can cause runtime errors and more specific type parameters should be used.
Common errors and solutions to generics in Go
Go is a widely used programming language. Generics were introduced in version 1.18. Although generics are a powerful tool, using them incorrectly can lead to puzzling errors. This article will explore some common mistakes with generics in Go and how to fix them.
Error 1: Pointer to type parameter
func Print[T any](ptr *T) { fmt.Println(*ptr) // 编译错误: 无效的指针解引用 }
In this code, the Print
function receives a T
type pointer. However, trying to dereference this pointer will result in a compilation error because T
is a type parameter, not a concrete type.
Solution:
Use a concrete type pointer:
func Print[T any](ptr *int) { fmt.Println(*ptr) // 成功打印 }
Error 2: Comparing a generic type to a non-generic value
func Equals[T comparable](a, b T) bool { return a == b // 编译错误: 无效的类型比较 }
Equals
Function is designed to compare elements of two generic types. However, type comparisons in Go are limited to concrete types.
Solution:
Use reflection to compare:
func Equals[T comparable](a, b T) bool { return reflect.DeepEqual(a, b) // 成功比较 }
Error 3: Abuse of empty interface
type MyMap[K comparable, V any] map[K]V func Merge[K comparable, V any](m MyMap[K, V], n MyMap[K, V]) MyMap[K, V] { for k, v := range n { m[k] = v // 运行时错误: 无效的类型断言 } return m }
Merge
The function attempts to merge two generic type maps. However, using the empty interface any
will result in a runtime error because the key-value pairs in the map cannot be correctly asserted as concrete types.
Solution:
Use more specific type parameters:
func Merge[K comparable, V int](m MyMap[K, V], n MyMap[K, V]) MyMap[K, V] { for k, v := range n { m[k] = v // 成功合并 } return m }
Practical case
Assumption We have a list that needs to store elements of different types:
type List[T any] []T func main() { list := List[int]{1, 2, 3} fmt.Println(len(list)) // 成功打印元素数量 }
In this example, we define a generic list type List
. By passing the element type as a type parameter, we can easily create a list storing ints and calculate its length.
Avoiding these common mistakes will help you write more robust and maintainable Go code. By using generics carefully and following best practices, we can take full advantage of this powerful feature.
The above is the detailed content of Common errors and solutions to generics in golang. For more information, please follow other related articles on the PHP Chinese website!
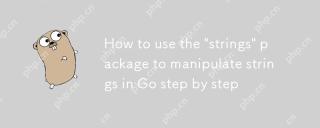
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
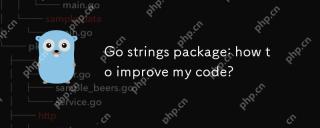
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
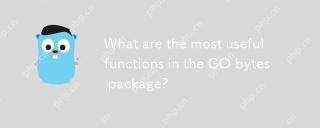
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
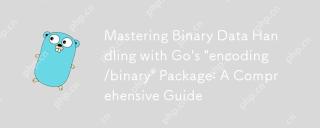
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
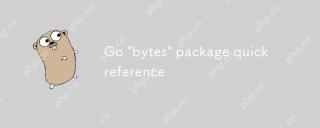
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
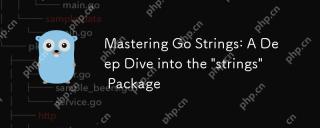
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
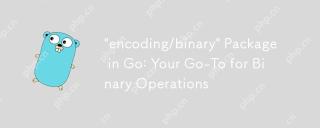
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
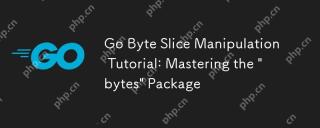
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
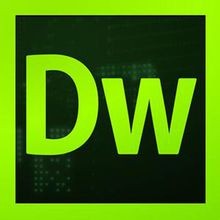
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
