Generics in Go enable code reuse by using type parameters to create reusable functions and algorithms, thereby reducing redundancy and improving code maintainability. Generic functions allow comparing the maximum value of different types (such as int and string) and verifying whether an element is present in a slice using reusable algorithms, providing a powerful tool for code reuse and flexibility.
Using generics in Go to achieve code reuse
Introduction
Generics allow the creation of reusable code across a variety of types, thereby reducing redundancy and improving code maintainability. Go 1.18 introduces generics, providing powerful new tools for code reuse.
Use generics to create reusable functions
In order to create a generic function, you need to use square brackets [] to specify the type parameters:
func Max[T comparable](x, y T) T { if x > y { return x } return y }
This function Use the type parameter T
as the element type for comparison. Due to the comparable
constraint, functions can only be used on types that can be compared to each other.
Practical case
Compare the maximum values of different types
We can use the generic functionMax
To compare the maximum value of different types:
var x int = 10 var y string = "five" fmt.Println(Max(x, y)) // 编译器错误,类型不匹配
To fix this error, we need to explicitly cast to the matching type:
yInt, _ := strconv.Atoi(y) fmt.Println(Max(x, yInt)) // 输出:10
Verify that the element is in the slice
Generics can also be used to write reusable algorithms, such as verifying whether an element exists in a slice:
func Contains[T comparable](slice []T, element T) bool { for _, v := range slice { if v == element { return true } } return false }
Practical case
Searching for elements in an integer slice
We can use the Contains
function to search for elements in an integer slice:
slice := []int{1, 2, 3} fmt.Println(Contains(slice, 2)) // 输出:true
Conclusion
By using generics in Go, we can create reusable code, reduce redundancy, and improve code maintainability. Generics are particularly useful for working with various data types and writing algorithms that can be applied to multiple types.
The above is the detailed content of Tips for implementing code reuse using generics in golang. For more information, please follow other related articles on the PHP Chinese website!
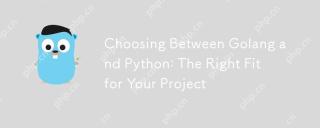
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
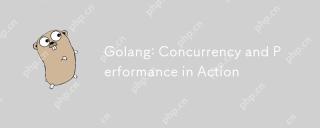
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
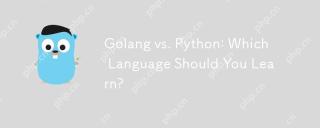
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
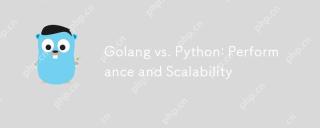
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
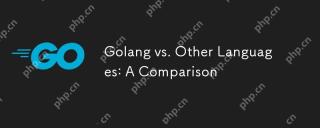
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
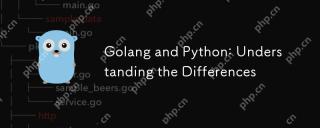
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
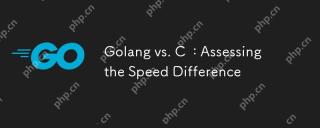
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
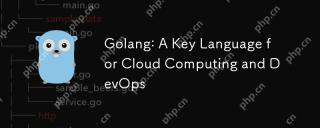
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
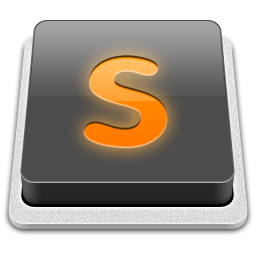
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.