Scalability design of golang function concurrent cache
Function concurrency cache can optimize performance in high concurrency scenarios by storing function calculation results in memory. It stores results using a concurrent-safe map and implements cache invalidation strategies as needed. For example, an example of concurrent caching for calculating the Fibonacci sequence demonstrates its advantages in avoiding repeated calculations and increasing execution speed.
Scalability design of function concurrent cache in Go language
Introduction
In high-concurrency scenarios, function calls often become performance issues bottleneck, especially when functions are expensive to process. To address this problem, we can adopt the function concurrent cache strategy to avoid repeated calculations and improve performance by storing function calculation results in memory.
Implementation Principle
1. Concurrent execution:
import "sync" type concurrentCache struct { sync.Mutex cache map[interface{}]interface{} } func (c *concurrentCache) Get(key interface{}) (interface{}, bool) { c.Lock() defer c.Unlock() val, ok := c.cache[key] return val, ok } func (c *concurrentCache) Set(key, val interface{}) { c.Lock() defer c.Unlock() c.cache[key] = val }
concurrentCache
maintains a concurrent and safe mapping for storage function calculation result. The Get
method gets the result from the map, while the Set
method stores the new result.
2. Cache invalidation:
In order to maintain the effectiveness of the cache, we need to consider the cache invalidation strategy according to specific scenarios. For example, we can set an expiration time or use the LRU (Least Recently Used) algorithm to cull less frequently used cache entries.
Practical example
The following is a simple function concurrent cache example based on concurrentCache
, used to calculate the Fibonacci sequence:
package main import "fmt" import "sync" var cache = &concurrentCache{cache: make(map[int]int)} func fibonacci(n int) int { if n <= 1 { return 1 } if val, ok := cache.Get(n); ok { return val.(int) } result := fibonacci(n-1) + fibonacci(n-2) cache.Set(n, result) return result } func main() { wg := sync.WaitGroup{} jobs := []int{10, 20, 30, 40, 50, 60} for _, n := range jobs { wg.Add(1) go func(n int) { defer wg.Done() result := fibonacci(n) fmt.Printf("Fibonacci(%d) = %d\n", n, result) }(n) } wg.Wait() }
In In this example, we cache the Fibonacci calculation function concurrently to avoid repeated calculations. By running this program, we can observe that concurrent calls are significantly faster than sequential execution.
Conclusion
Function concurrent caching is an effective method to improve performance in high concurrency scenarios. By adopting concurrency-safe data structures such as concurrentCache
and taking into account cache invalidation strategies, we can design a scalable and efficient function concurrent cache.
The above is the detailed content of Scalability design of golang function concurrent cache. For more information, please follow other related articles on the PHP Chinese website!
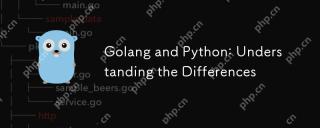
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
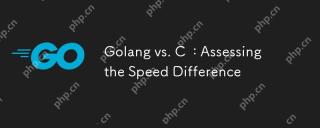
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
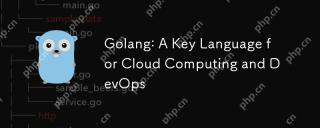
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
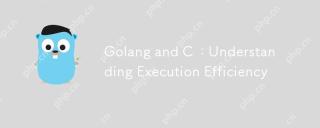
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
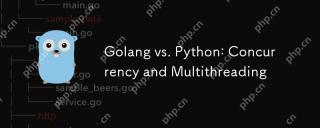
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
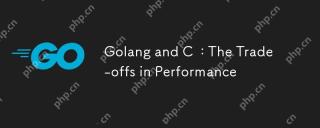
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
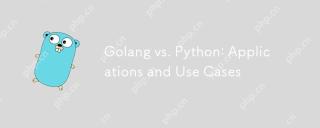
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
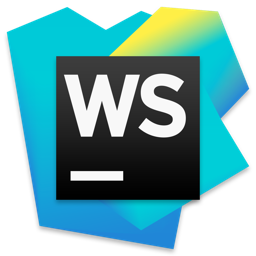
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor