


Recursion is a programming technique that solves problems through function self-tuning. In C, it can be achieved by calling itself and passing different parameters. Optimization techniques include tail recursive optimization, memoization, and pruning. Recursive code is generally less efficient than iterative code, but may still be a better choice when it provides a cleaner and cleaner solution.
In-depth analysis of C recursion: principles, implementation and optimization techniques
Principles
Recursion is a programming technique that solves a problem by calling itself inside a function. When a function calls itself, a new instance of the function is created, passing different arguments. When the new instance executes, it calls the original instance, and so on, until the recursion stopping condition is reached.
Implementation
In C, the implementation of the recursive function is as follows:
void recursive_function(int n) { if (n <= 0) { // 递归停止条件 return; } // 执行某些操作 recursive_function(n - 1); // 递归调用 }
Optimization technology
To improve the efficiency of recursive code, the following optimization techniques can be used:
- Tail recursion optimization: If the recursive call is the last step in the function, the compiler can convert the recursion into Iterate, thereby eliminating the overhead of function calls.
- Memo: Improve efficiency by storing the results of previous calculations to avoid double calculations.
- Pruning: Reduce the number of recursive calls by avoiding them for certain situations.
Practical case
The following is an example of a recursive C function that calculates factorial:
int factorial(int n) { if (n <= 1) { // 递归停止条件 return 1; } return n * factorial(n - 1); // 递归调用 }
Performance considerations
Recursive code is generally less efficient than iterative code because recursion creates new function instances and stores intermediate results. Therefore, the performance of recursion is limited both in terms of space and time.
In practice, the decision whether to use recursion should be based on the specific problem. If the problem can be solved by a more efficient iterative method, the iterative method should be used in preference. However, if recursion provides a clearer and concise solution, it may still be the better choice.
The above is the detailed content of An in-depth analysis of C++ recursion: principles, implementation and optimization techniques. For more information, please follow other related articles on the PHP Chinese website!
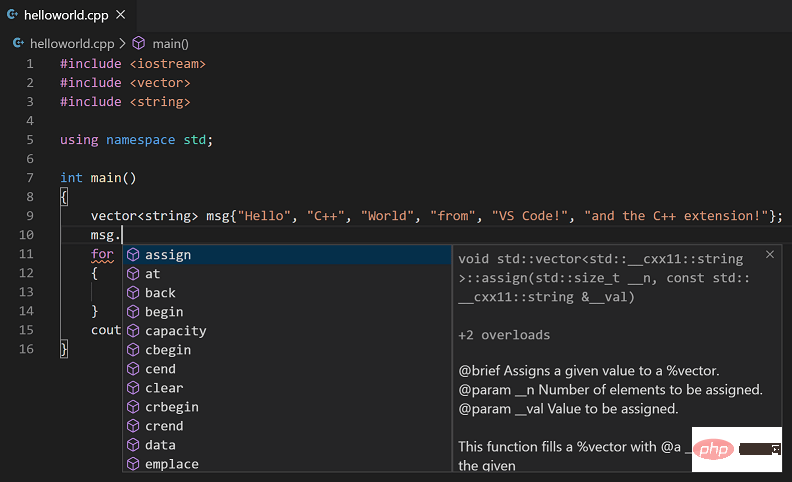
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
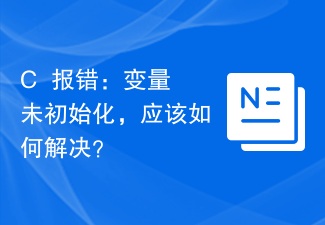
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
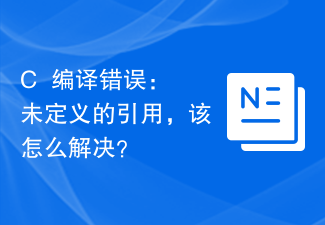
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
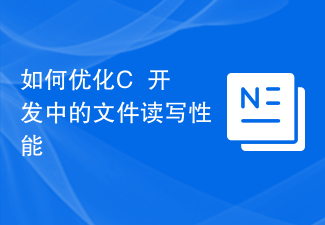
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
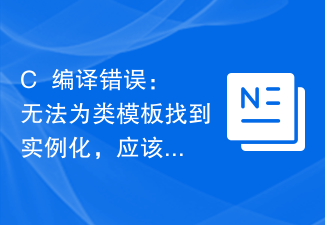
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
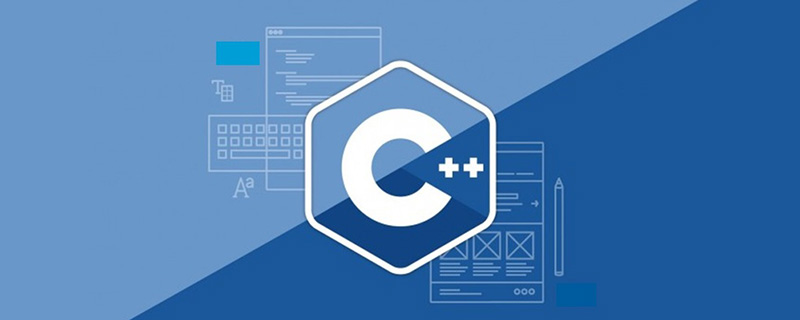
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
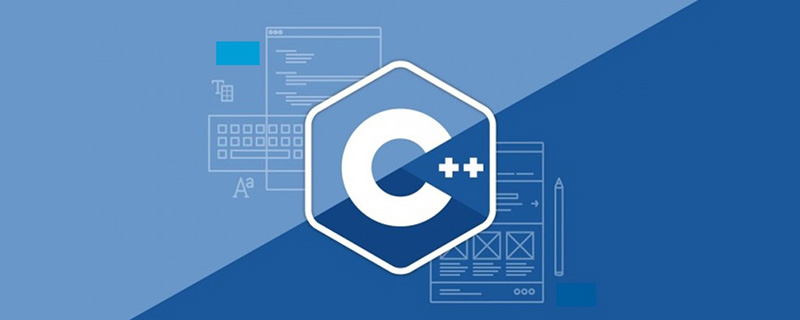
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
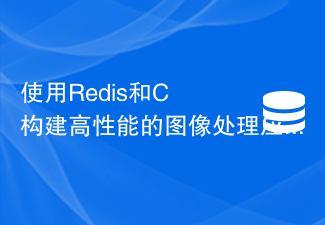
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
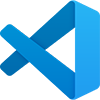
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
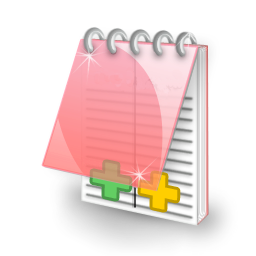
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment
