How to avoid side effects in golang functional programming?
It is crucial to avoid side effects in functional programming to ensure the purity of the program. In Go language, avoid side effects through the following tips: Use immutable data types Use functions to pass data as parameters Use concurrency-safe data structures Use error handling instead of panic or fatal Avoid printing to the console
Tips to avoid side effects in Go functional programming
In functional programming, avoiding side effects is crucial, which ensures the purity of the program and makes it easy to reason about and test. The Go language supports functional programming, but its concurrency nature can also lead to side effects. This article will introduce how to avoid side effects in Go functional programming and provide practical examples.
What are side effects?
Side effects refer to the behavior of a function that changes its external environment, such as changing global variables, writing to a file, or printing to the console. Side effects can make a program difficult to reason about because it introduces implicit dependencies beyond the scope of the function.
Sources of side effects in functional programming in Go
Potential sources of side effects in functional programming in Go include:
- Manipulating global variables
- Modify mutable containers such as slices, maps or channels
- Panic or fatal
- Print to the console
Tips to avoid side effects
To avoid side effects, follow these tips:
1. Use immutable data types
Use immutable data types, such as Strings and integers can be protected against accidental modification.
2. Use functions as parameters to pass data
Using functions as parameters to pass data can avoid changing global variables or external states.
3. Use concurrency-safe data structures
Use concurrency-safe data structures, such as sync.Map or atomic operations, to safely access shared data.
4. Use error handling instead of panic or fatal
Use error handling mechanism instead of panic or fatal, you can control the error handling process without affecting the program state.
5. Avoid printing to the console
Avoid printing to the console in a function, which will introduce side effects unrelated to the function return.
Practical Case
The following example demonstrates how to avoid side effects in Go functional programming:
// 不受副作用影响的函数 func Sum(nums []int) int { sum := 0 for _, num := range nums { sum += num } return sum } // 产生副作用的函数 func AppendToList(list []int, num int) { list = append(list, num) } func main() { // 使用不可变数据类型和函数作为参数 fmt.Println(Sum([]int{1, 2, 3})) // 输出:6 // 使用并发安全数据结构 m := sync.Map{} m.Store("key", 42) fmt.Println(m.Load("key")) // 输出:42 // 使用错误处理代替 fatal err := DoSomething() if err != nil { fmt.Println(err) } }
In the above example, the Sum function uses Immutable data types and function parameters avoid side effects. The AppendToList function produces side effects by explicitly modifying the list.
The above is the detailed content of How to avoid side effects in golang functional programming?. For more information, please follow other related articles on the PHP Chinese website!
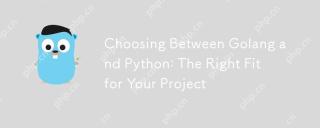
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
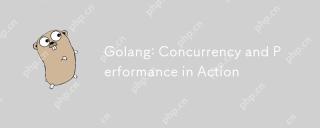
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
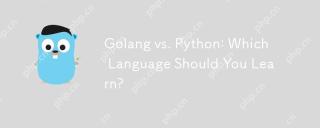
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
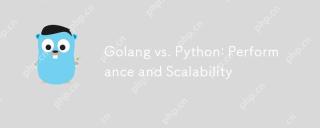
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
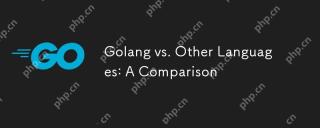
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
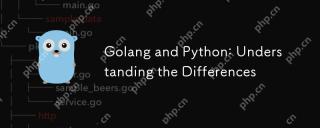
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
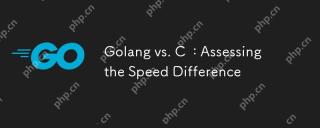
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
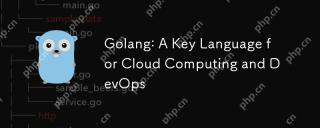
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
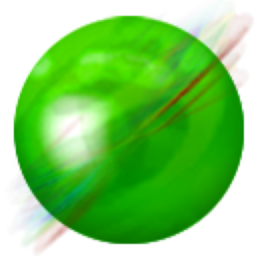
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
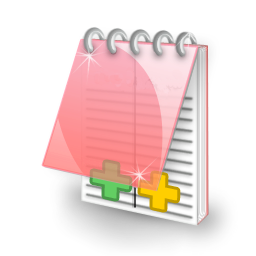
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
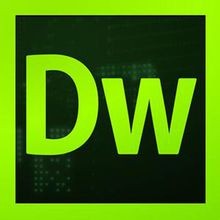
Dreamweaver CS6
Visual web development tools