What's the best way to optimize Java functions for error handling?
There are the following methods for function error handling in Java: use Function interface and try-catch block to catch exceptions and customize error handling logic. Handle null values in an elegant way using Java 10's Optional class to represent values that may or may not exist. Use functional programming libraries (such as Lombok) to simplify error handling and automatically catch exceptions through annotations.
Optimize the way Java functions handle errors
Use the Function interface of Java 8
Function
The interface provides a simple way to define a function that maps one parameter to another result. It also provides elegant error handling mechanism.
import java.util.function.Function; public class ErrorHandlingWithFunction { public static void main(String[] args) { Function<String, Integer> parseIntFunction = s -> Integer.parseInt(s); // 捕获 `parseInt` 抛出的 `NumberFormatException` Function<String, Integer> parseIntWithTryCatch = s -> { try { return parseIntFunction.apply(s); } catch (NumberFormatException e) { // 处理错误并返回默认值 return -1; } }; System.out.println(parseIntWithTryCatch.apply("123")); // 输出:123 System.out.println(parseIntWithTryCatch.apply("abc")); // 输出:-1 } }
Using Java 10’s java.util.Optional
Optional
class can represent an object that may or may not exist value. It provides an elegant way to handle null
values.
import java.util.Optional; public class ErrorHandlingWithOptional { public static void main(String[] args) { String input = "123"; Optional<Integer> integerOptional = Optional.ofNullable(input) .map(Integer::parseInt); // `map` 函数将字符串映射到整数 // 检查是否存在值 if (integerOptional.isPresent()) { System.out.println(integerOptional.get()); // 输出:123 } else { System.out.println("输入不是数字"); } } }
Use a functional programming library
You can also use a functional programming library like Lombok to simplify error handling.
import lombok.SneakyThrows; public class ErrorHandlingWithLombok { @SneakyThrows // 自动处理 `NumberFormatException` public static int parseInt(String s) { return Integer.parseInt(s); } public static void main(String[] args) { System.out.println(parseInt("123")); // 输出:123 } }
The above is the detailed content of What's the best way to optimize Java functions for error handling?. For more information, please follow other related articles on the PHP Chinese website!
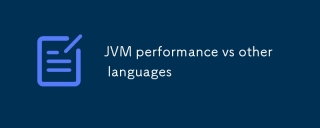
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
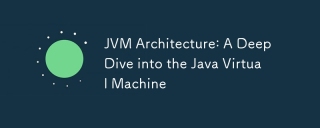
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
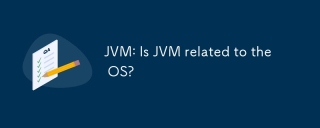
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
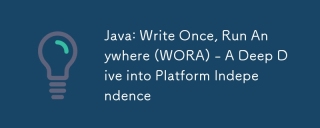
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
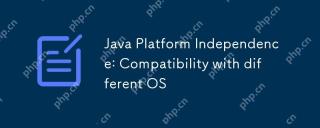
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
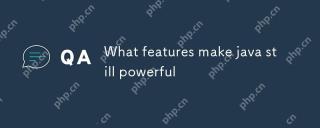
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
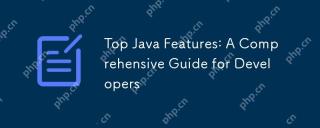
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
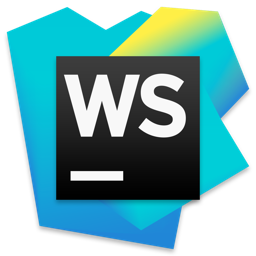
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
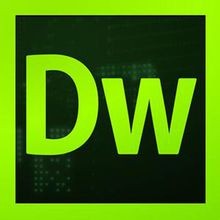
Dreamweaver CS6
Visual web development tools
