Answer: You can customize the logging strategy and format of Java functions by extending the logging mechanism. Extended step: Create a custom logging configurator. Set logging level. Create a custom logging formatter.
Extending the logging mechanism in Java functions to meet custom needs
When building Java functions, the default logging mechanism may not meet specific needs . This article will guide you on how to extend the logging mechanism of Java functions to customize the logging strategy and format.
Logging Framework
Java functions use the Java Logging Framework for logging by default. The framework provides several logging levels, including INFO, WARN, ERROR, and FATAL.
Extended logging mechanism
To extend the logging mechanism, you need to create a custom logging configurator:
import java.util.logging.Logger; // 创建自定义日志记录配置器 Logger logger = Logger.getLogger("my-custom-logger"); // 设置日志记录级别 logger.setLevel(Level.FINE); // 自定义日志记录格式 logger.addHandler(new SimpleFormatterHandler());
Custom logging formatter
SimpleFormatterHandler
Class is responsible for formatting log messages. You can create a custom formatter by implementing the Formatter
interface:
import java.text.SimpleDateFormat; import java.util.Date; import java.util.logging.Formatter; import java.util.logging.LogRecord; public class MyCustomFormatter extends Formatter { @Override public String format(LogRecord record) { // 使用自定义格式化字符串 return String.format("[%s] %s - %s\n", new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()), record.getLoggerName(), record.getMessage()); } }
Practical case
Assume there is a Java function that handles incoming requests and records logs. Here are the steps to extend its logging mechanism:
- Import the necessary logging packages in the function code.
- Create a custom logging configurator and set the desired logging level.
- Create a custom logging formatter and add it to the logging configurator.
Code Example:
import com.google.cloud.functions.HttpFunction; import com.google.cloud.functions.HttpRequest; import com.google.cloud.functions.HttpResponse; import java.util.logging.Level; import java.util.logging.Logger; public class MyCustomizedLoggingFunction implements HttpFunction { private static final Logger logger = Logger.getLogger("my-custom-logger"); static { // 初始化日志记录 logger.setLevel(Level.FINE); logger.addHandler(new SimpleFormatterHandler()); } @Override public void service(HttpRequest request, HttpResponse response) throws IOException { // 省略其他代码... // 记录请求信息 logger.info("Received request: " + request.getUri()); } }
By following these steps, you can easily extend the logging mechanism of a Java function to meet custom needs.
The above is the detailed content of How can I extend the logging mechanism in Java functions to meet custom needs?. For more information, please follow other related articles on the PHP Chinese website!
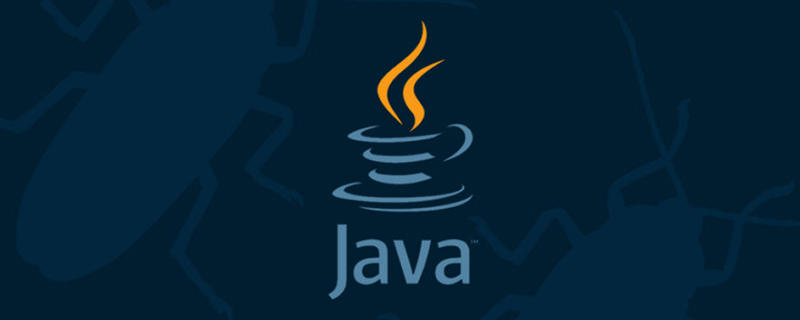
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
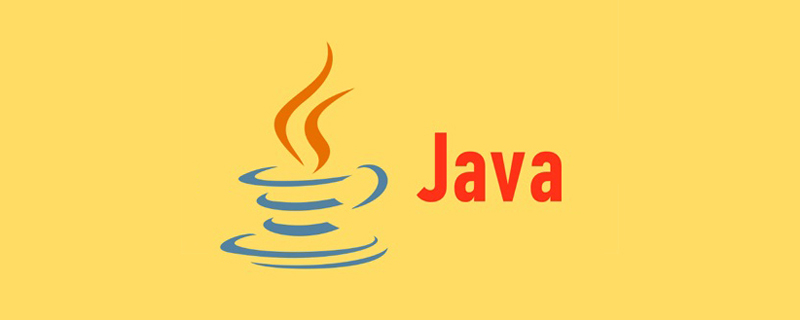
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
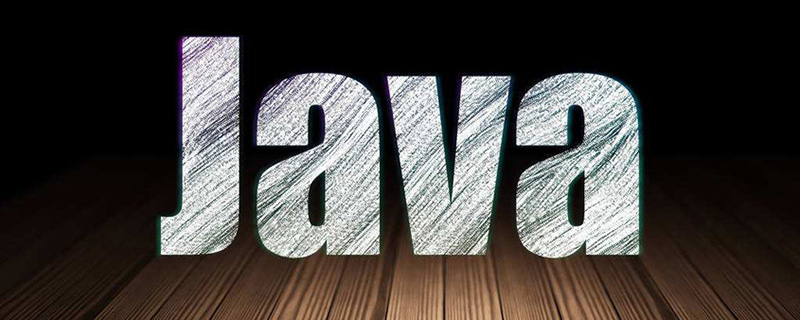
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
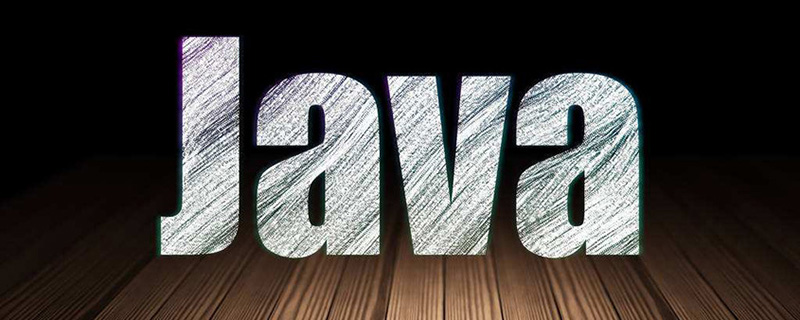
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
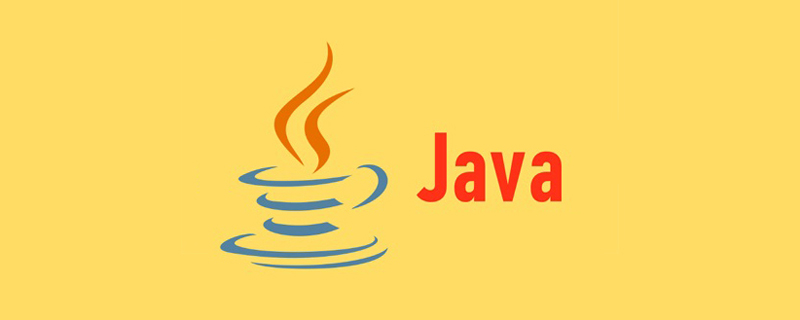
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
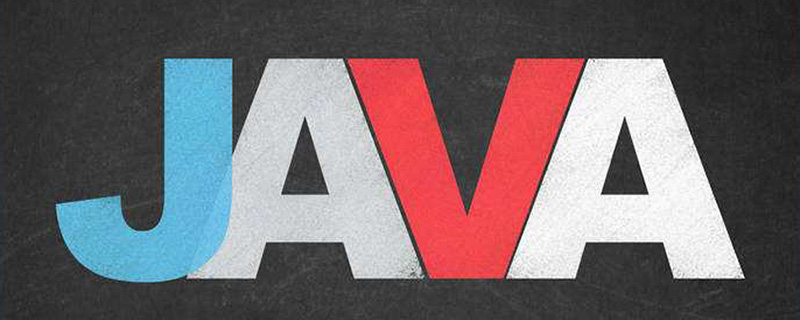
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
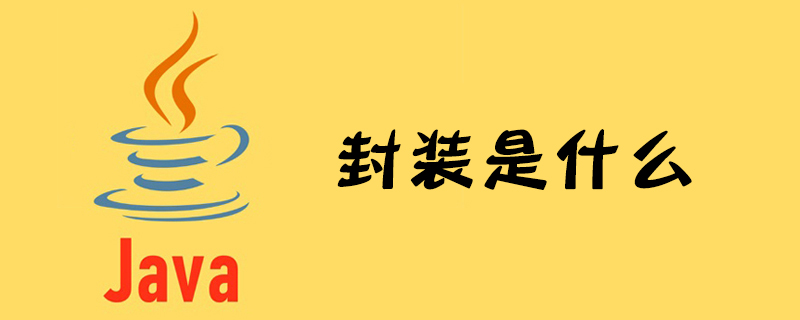
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
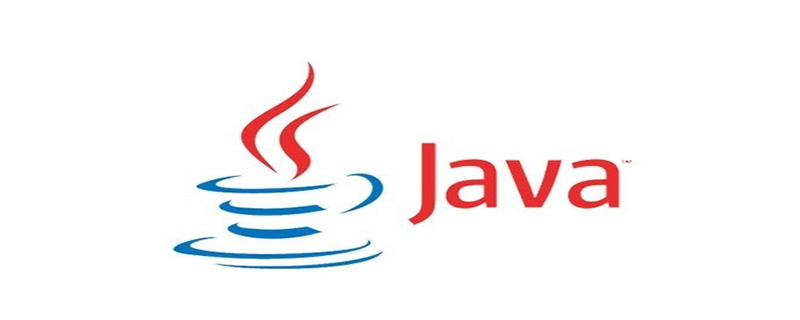
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
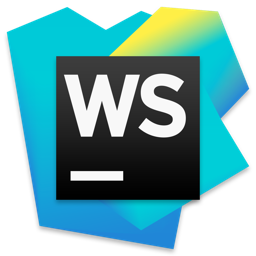
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
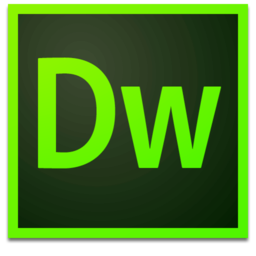
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
