Time complexity measures the efficiency of an algorithm and represents the asymptotic behavior of the time required for algorithm execution. Big O notation is used in Java to represent time complexity. Common ones are: O(1), O(n), O(n^2), O(log n). The steps for calculating the time complexity of an algorithm include: determining basic operations, calculating the number of basic operations, summarizing basic operation times, and simplifying expressions. For example, a linear search algorithm that traverses n elements has a time complexity of O(n), and the search time increases linearly as the size of the list grows.
Time complexity calculation method in Java
What is time complexity?
Time complexity is a measure of algorithm efficiency, which describes the time required for an algorithm to execute when the amount of input data is different.
How to calculate time complexity in Java?
Time complexity in Java is usually expressed in big O notation, which represents the asymptotic behavior of a function as the number of inputs approaches infinity. Here are some common time complexity representations:
- O(1): Constant time, the time complexity is constant regardless of the input size.
- O(n): Linear time, the time complexity grows proportionally to the input size n.
- O(n^2): Square time, the time complexity grows proportionally to the square of the input size n.
- O(log n): Logarithmic time, time complexity grows logarithmically with input size n.
How to calculate the time complexity of a specific algorithm?
The steps to calculate the time complexity of a specific algorithm are as follows:
- Identify the basic operations: Identify the basic operations that are performed most frequently in the algorithm.
- Calculate the number of basic operations: Determine the number of times each basic operation is performed for a given input size.
- Summarize basic operation times: Multiply the time complexity of each basic operation by the number of times it is executed, and add them together.
- Simplify the expression: Eliminate the constant factors and retain the highest order term related to the input size.
Example:
Consider the following linear search algorithm for finding elements in a list:
public int linearSearch(List<Integer> list, int target) { for (int i = 0; i < list.size(); i++) { if (list.get(i) == target) { return i; } } return -1; }
- Basic operations: Traverse each element in the list.
- Number of basic operations: n, where n is the size of the list.
- Summary basic operation time: n * 1 = n
- Simplified expression: The time complexity is O(n).
Therefore, the time complexity of this linear search algorithm is O(n), which means that as the list size grows, the time required for searching will increase linearly.
The above is the detailed content of How to calculate time complexity in java. For more information, please follow other related articles on the PHP Chinese website!
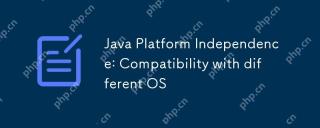
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
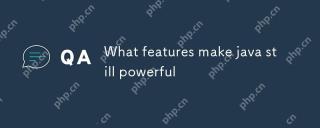
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
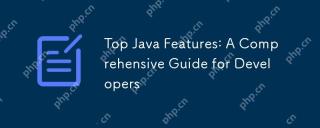
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
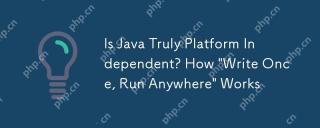
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
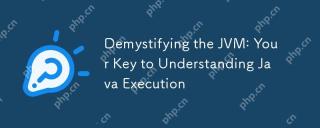
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
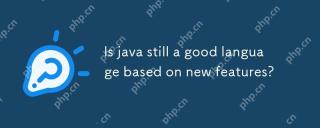
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
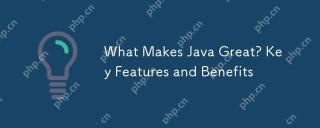
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
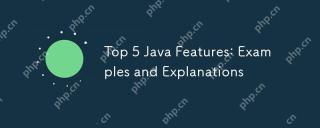
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
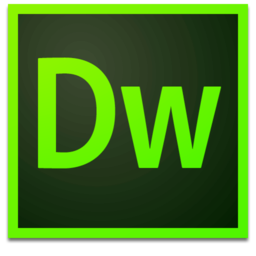
Dreamweaver Mac version
Visual web development tools
