Both closures and lambda expressions can capture local variables in Java: closures can capture free variables, while lambda expressions can only capture final or effectively final variables. A closure's free variables are accessible throughout its lifetime, whereas a lambda expression's captured variables are only accessible within the Lambda. A closure's free variables are stored on the heap, while a lambda expression's captured variables are stored on the stack. Lambda expressions create closures, and closures can contain Lambda expressions.
The difference and connection between closures and Lambda expressions in Java
Preface
Closures and Lambda expressions are both very useful techniques in Java programming. Both of them can capture local variables and store them outside the scope. However, there are some key differences between them.
Closures
A closure is a feature that captures variables declared in the environment in which it is executed, even after leaving that environment. These captured variables are called the "free variables" of the closure.
Lambda expression
Lambda expression is a simplified anonymous function. They can accept parameters and return a value. A lambda expression can also capture variables declared in its execution environment, but only if those variables are final(final) or effectively final(effectively final).
Differences
Although closures and Lambda expressions can both capture local variables, there are some key differences between them:
- Free variables: Closures can capture free variables, while Lambda expressions can only capture final or effectively final variables.
- Scope: Free variables of a closure can be accessed throughout their lifetime, even after leaving the scope in which they are defined. In contrast, a lambda expression's capture variables can only be accessed within the lambda expression.
- Memory management: Free variables of closures are stored in the heap, while captured variables of lambda expressions are stored in the stack.
Connection
Despite these differences, closures and lambda expressions are closely related:
- Lambda expressions can create closures: When a Lambda expression captures a free variable, it creates a closure.
- Closures can contain Lambda expressions: Closures can store Lambda expressions as free variables.
Practical case
Closure case:
class OuterClass { private int x = 10; public void printX() { Runnable runnable = () -> System.out.println(x); // 闭包捕获自由变量 x runnable.run(); } }
Lambda expression case:
class OuterClass { private final int x = 10; public void printX() { System.out.println(x); // Lambda 表达式可以使用 final 变量 } }
Conclusion
Closures and Lambda expressions are both useful techniques in Java. Understanding the differences and connections between them is important to writing efficient, maintainable code.
The above is the detailed content of The difference and connection between Java closures and lambda expressions. For more information, please follow other related articles on the PHP Chinese website!
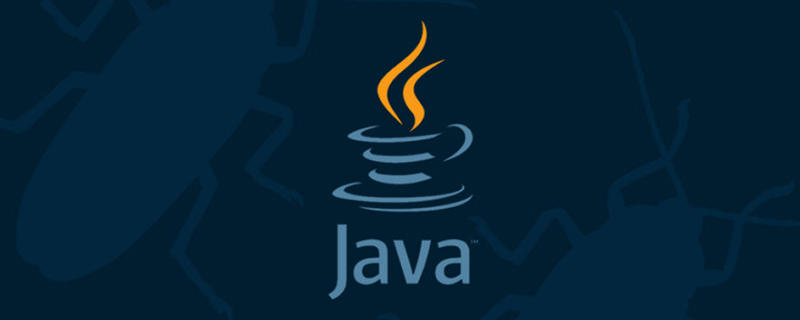
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
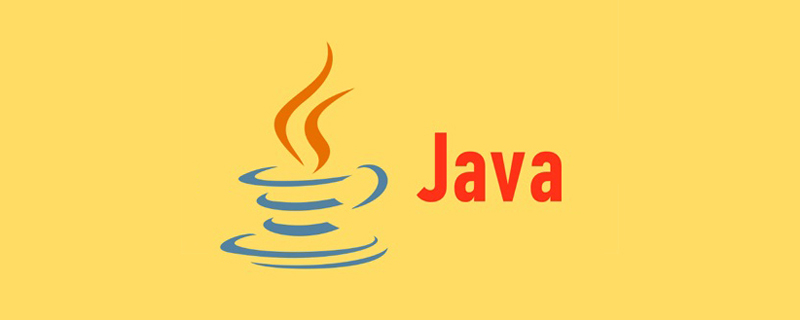
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
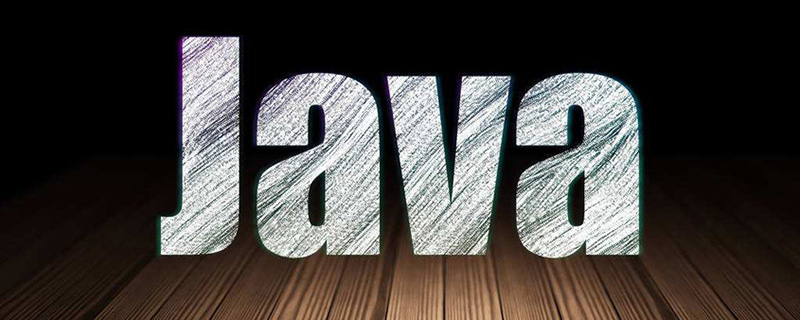
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
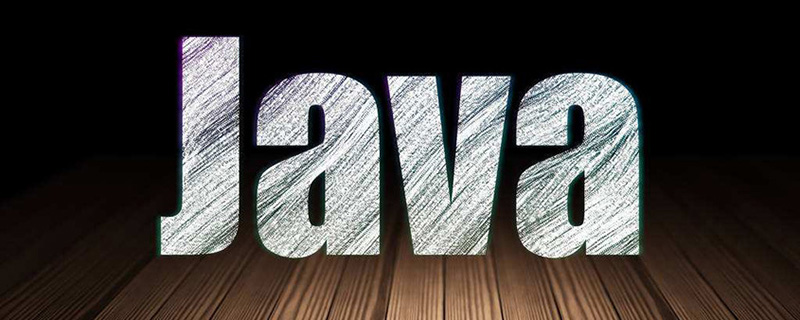
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
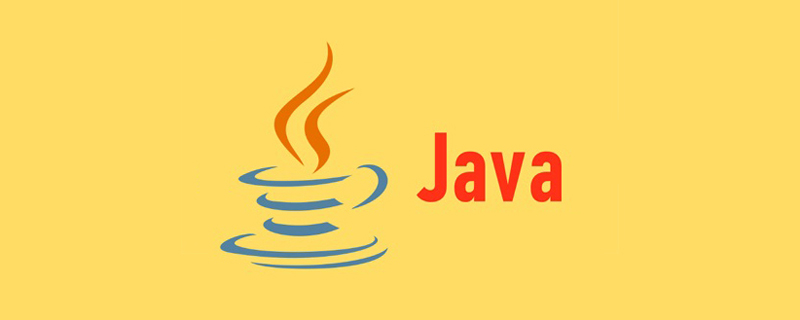
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
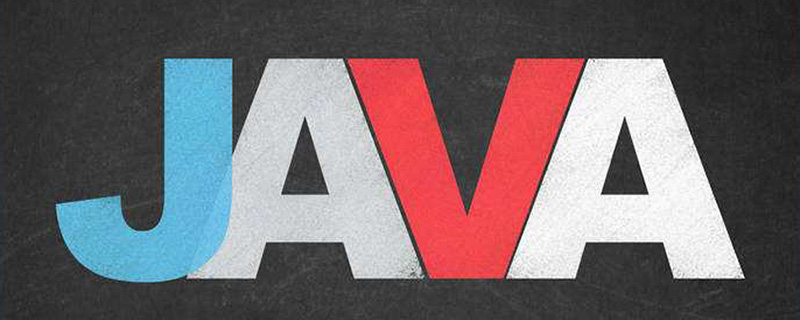
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
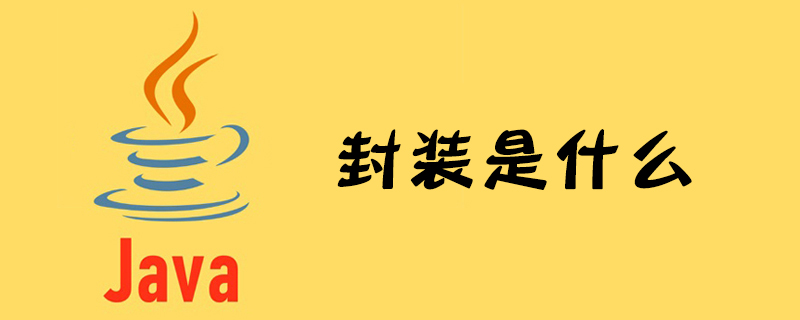
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
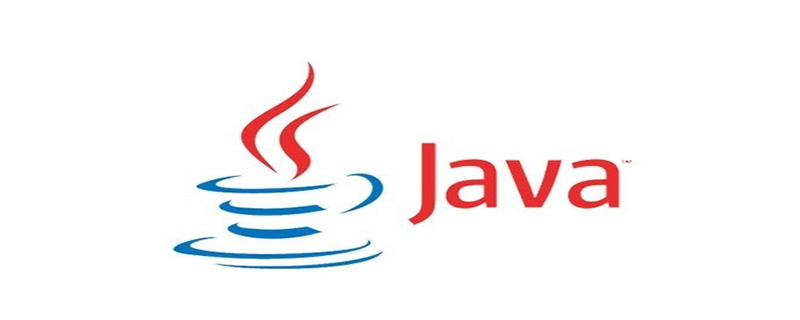
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
