How can blocking queues in Java avoid thread starvation issues?
The blocking queue in Java can avoid thread starvation problems by using the following methods: using fair locks (ReentrantLock) to ensure that threads have a fair chance to access resources. Use condition variables (Condition) to allow threads to wait until specific conditions are met.
How blocking queue in Java avoids thread starvation problem
The blocking queue is a thread-safe data structure that allows Threads retrieve or insert elements from the queue. However, when the queue is empty, threads trying to retrieve elements will be blocked, and when the queue is full, threads trying to insert elements will also be blocked.
In some cases, blocking queues may encounter thread starvation problems, that is, some threads are blocked for a long time and cannot obtain resources or perform tasks. This can cause system performance degradation or deadlocks.
Using fair locks
One way to solve the problem of thread starvation is to use fair locks. Fair locks guarantee that each thread will get a fair chance when accessing a resource. In Java, you can create fair locks using the ReentrantLock
class. The following code example demonstrates how to use fair locks to protect blocking queues:
import java.util.concurrent.BlockingQueue; import java.util.concurrent.LinkedBlockingQueue; import java.util.concurrent.locks.ReentrantLock; public class FairBlockingQueue<E> { private final BlockingQueue<E> queue; private final ReentrantLock lock; public FairBlockingQueue() { this.queue = new LinkedBlockingQueue<>(); this.lock = new ReentrantLock(true); // 使用公平锁 } public void put(E element) throws InterruptedException { lock.lock(); try { queue.put(element); } finally { lock.unlock(); } } public E take() throws InterruptedException { lock.lock(); try { return queue.take(); } finally { lock.unlock(); } } }
Using condition variables
Another way to solve the problem of thread starvation is to use condition variables. Condition variables allow a thread to wait until a specific condition is met. In Java, you can create condition variables using the Condition
class. The following code example demonstrates how to use condition variables to protect blocking queues:
import java.util.concurrent.BlockingQueue; import java.util.concurrent.LinkedBlockingQueue; import java.util.concurrent.locks.Condition; import java.util.concurrent.locks.ReentrantLock; public class ConditionBlockingQueue<E> { private final BlockingQueue<E> queue; private final ReentrantLock lock; private final Condition notEmpty; private final Condition notFull; public ConditionBlockingQueue(int capacity) { this.queue = new LinkedBlockingQueue<>(capacity); this.lock = new ReentrantLock(); this.notEmpty = lock.newCondition(); this.notFull = lock.newCondition(); } public void put(E element) throws InterruptedException { lock.lock(); try { while (queue.size() == queue.remainingCapacity()) { notFull.await(); } queue.put(element); notEmpty.signal(); } finally { lock.unlock(); } } public E take() throws InterruptedException { lock.lock(); try { while (queue.isEmpty()) { notEmpty.await(); } E element = queue.take(); notFull.signal(); return element; } finally { lock.unlock(); } } }
By using fair locks or condition variables, we can ensure that each thread has a fair chance to access the blocking queue, thereby avoiding thread starvation problems.
The above is the detailed content of How can blocking queues in Java avoid thread starvation issues?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
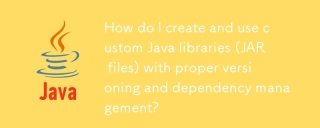
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
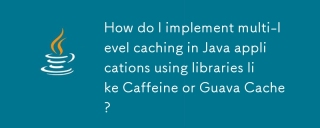
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
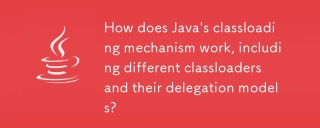
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
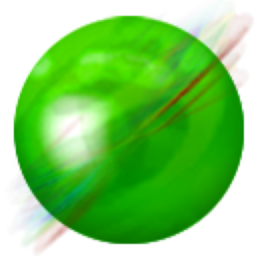
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
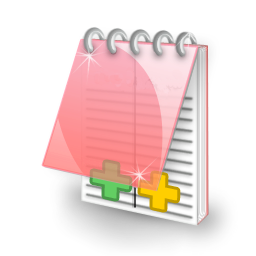
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
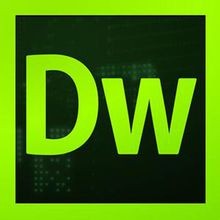
Dreamweaver CS6
Visual web development tools