


C++ function rewriting in practice: a trick to implement subclass-specific functions
Function rewriting allows subclasses to redefine functions of the same name of the base class to implement functions specific to the subclass: based on name lookup and type matching, when a subclass defines a function of the same name, the base class function will be rewritten. This allows subclasses to provide different implementations, for example the Circle and Rectangle classes in the example override the area() function of the Shape class to calculate their own area.
C Function rewriting: Implementation of subclass-specific functions
Function rewriting is an important mechanism in C. It allows subclasses to redefine the behavior of functions of the same name in the base class. This is useful for defining specific functionality specific to a subclass.
Principle
Function rewriting is based on name lookup and type matching rules. When a function is called, the compiler searches the scope for a function with a matching name. If multiple overloaded functions are found, the best match principle is used for selection.
If the subclass defines a function with the same name as the base class, the function in the subclass will override the function in the base class. This allows subclasses to provide a different implementation than the base class.
Practical case
Consider a scenario where we have a Shape
base class that represents a general shape. Derived classes Circle
and Rectangle
represent circles and rectangles respectively. We need to calculate the area of these shapes.
Base class Shape
class Shape { public: virtual double area() const = 0; // 纯虚函数 };
Derived class Circle
class Circle : public Shape { public: Circle(double radius) : radius(radius) {} double area() const override { return M_PI * radius * radius; } private: double radius; };
Derived class Rectangle
class Rectangle : public Shape { public: Rectangle(double width, double height) : width(width), height(height) {} double area() const override { return width * height; } private: double width, height; };
Using
We can use these classes to calculate the area of different shapes:
int main() { Circle circle(5.0); Rectangle rectangle(3.0, 4.0); std::cout << "Area of circle: " << circle.area() << std::endl; std::cout << "Area of rectangle: " << rectangle.area() << std::endl; return 0; }
Output:
Area of circle: 78.5398 Area of rectangle: 12.0
In this example, The Circle
and Rectangle
classes override the area()
function defined in the Shape
class. This allows us to implement area calculation logic specific to each shape.
The above is the detailed content of C++ function rewriting in practice: a trick to implement subclass-specific functions. For more information, please follow other related articles on the PHP Chinese website!
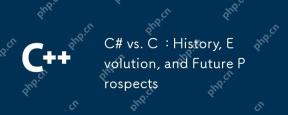
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
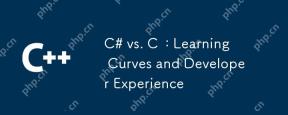
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
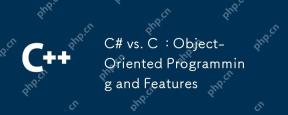
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
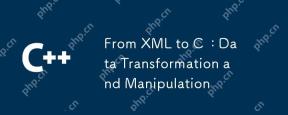
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
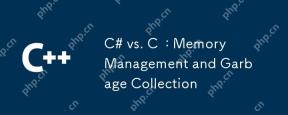
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
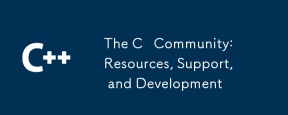
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
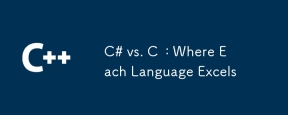
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
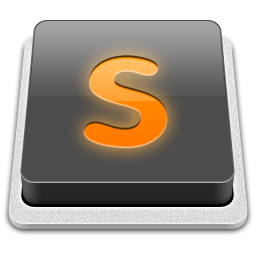
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.