Master the secrets of C++ recursion: Concept and example analysis
Answer: C Recursion is a powerful technique for a function to call itself. Detailed description: Recursive functions break the problem into smaller sub-problems. The function calls itself recursively to solve the subproblem until the subproblem is small enough. Common recursive practical cases include: factorial calculation, Fibonacci sequence, Hanoi's Tower, quick sort and depth-first search. The advantage of recursion is that it is simple and easy to understand and can solve complex problems efficiently. The disadvantages are that the call stack can overflow, large inputs can cause performance degradation, and debugging can be challenging.
Master the secrets of C recursion: Concept and example analysis
Introduction
Recursion is a powerful technique in computer science that allows a function to solve a problem by calling itself. In C, recursion can provide concise and efficient solutions in many situations. This article will delve into the concept of C recursion and provide practical examples to help you master this valuable skill.
The concept of recursion
The basic idea of the recursive function is to decompose the problem into smaller and simpler sub-problems than the original problem. The function then calls itself recursively to solve each subproblem until the subproblem is simple enough to be solved in other ways.
In C, you can call a function recursively using the keyword return
:
int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } }
This function computes the factorial of a specified integer.
Practical cases
The following are some common recursive practical cases:
- Factorial calculation:Calculate the given Factorial of an integer.
- Fibonacci Sequence: Generate the sequence in the Fibonacci Sequence.
- Tower of Hanau: Move the discs on the tower to follow specific rules.
- Quick sort: Use the recursive quick sort algorithm to sort the array.
- Depth-first search: Use recursion to traverse a tree or graph.
Pros and Cons
Pros:
- Concise and easy to understand solution
- Can effectively decompose complex problems
- Provides optimal efficiency for certain algorithms (such as quick sort)
Disadvantages:
- The call stack may overflow, causing runtime errors
- Larger input sizes may cause performance degradation
- Debugging recursive functions can be challenging
conclusión
Recursion is a powerful tool in C that can help solve complex problems. By understanding the concept of recursion and studying practical examples, you can master this technique and improve your programming skills.
The above is the detailed content of Master the secrets of C++ recursion: Concept and example analysis. For more information, please follow other related articles on the PHP Chinese website!
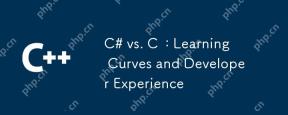
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
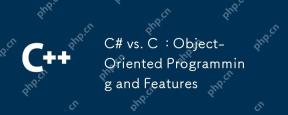
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
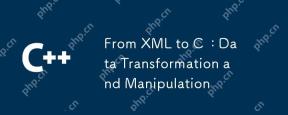
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
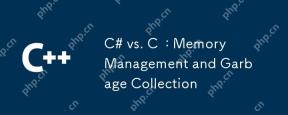
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
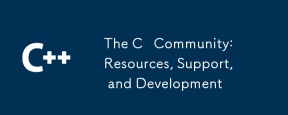
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
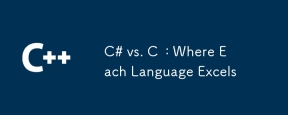
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
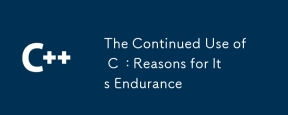
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
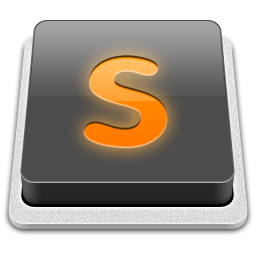
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use