C Function naming best practices: Use verb-noun convention (for example: InitilizeAccount()) Avoid using negative words (for example: DisableNegation()) Keep names concise (for example: PerformAction()) Optional: Use the Hungarian notation convention (For example: nCount, cBuffer)
Best practices for naming C functions in object-oriented design
Function naming in C-oriented design Very important in object design. Clear and understandable function names can enhance the readability and maintainability of your code. Here are a few best practices:
Use verb-noun convention
Use verb-noun order, where the verb describes what the function does and the noun represents what the function operates on . For example, InitilizeAccount()
and DeleteUser()
.
void InitilizeAccount(Account& account); void DeleteUser(const User& user);
Avoid using negative words
Negative words can make function names difficult to understand. For example, DontUseNegation()
is harder to understand than DisableNegation()
.
Keep names simple
Use concise, descriptive names. Avoid lengthy or vague names.
// 冗长 bool PerformActionOnData(const Data& data) { ... } // 简洁 bool PerformAction(const Data& data) { ... }
Use the Hungarian notation convention (optional)
The Hungarian notation convention uses prefixes in variable and parameter names to indicate type or purpose. While this is not required, it can provide additional clarity.
int nCount; // 整数计数器 char cBuffer[10]; // 字符缓冲区
Practical case
Consider a banking system that manages user accounts. Function naming can look like this:
// 初始化账户 void InitializeAccount(Account& account); // 删除账户 void DeleteAccount(const Account& account); // 添加用户 void AddUser(const User& user, Account& account); // 更新用户 void UpdateUser(const User& user); // 登录用户 bool LoginUser(const string& username, const string& password); // 登出用户 void LogoutUser(const User& user);
By following these best practices, you can write C function names that are readable, maintainable, and easy to understand, thereby improving overall code quality.
The above is the detailed content of C++ function naming best practices in object-oriented design. For more information, please follow other related articles on the PHP Chinese website!
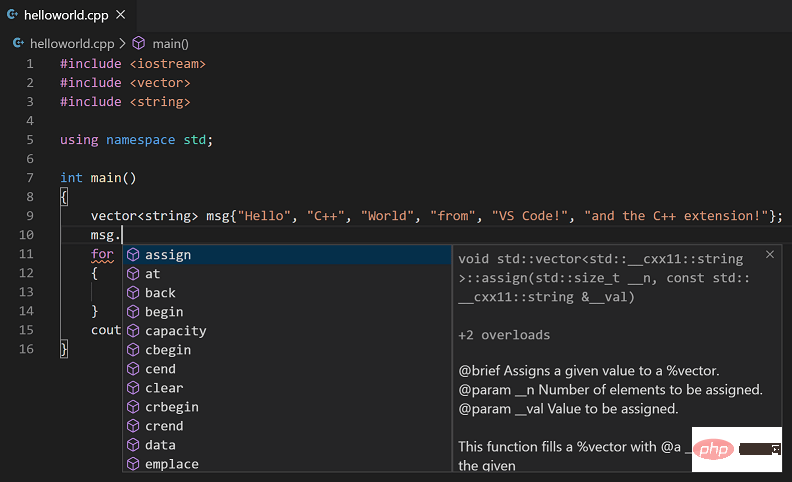
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
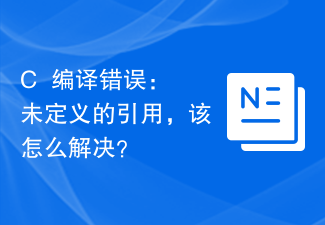
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
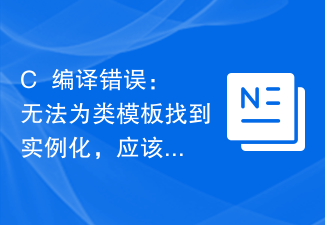
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
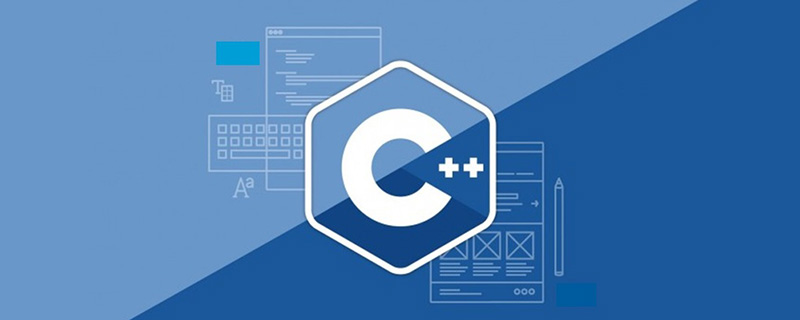
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
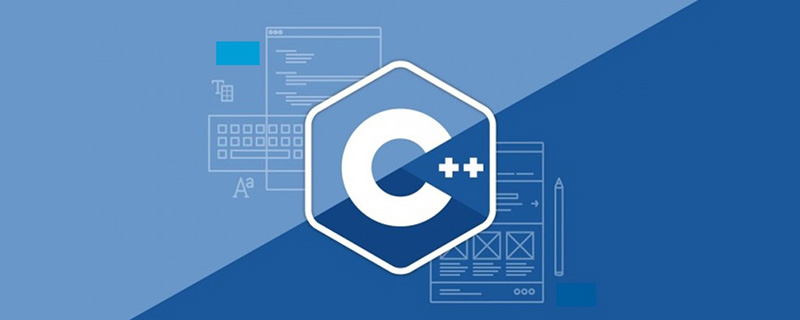
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
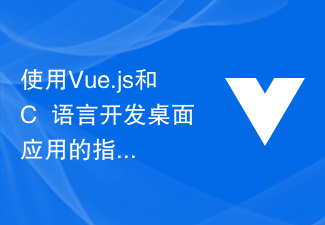
使用Vue.js和C++语言开发桌面应用的指南随着互联网的发展,前端技术也在不断更新和进步。而Vue.js作为一种轻量级、高效、易用的前端框架,在开发Web应用方面具有很大的优势。然而,在一些特定的场景中,我们可能需要开发一些更加复杂的桌面应用程序,这时候就需要结合C++语言来实现一些底层功能。本文将会介绍如何使用Vue.js和C++语言开发桌面应用,并提供
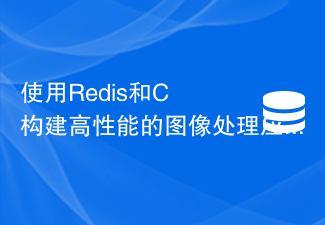
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同
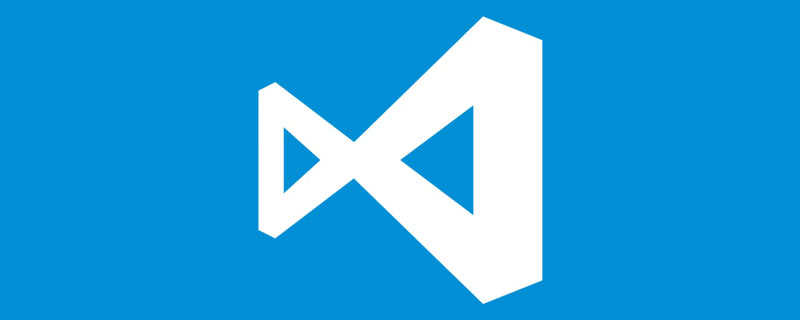
VSCode历史版本的下载安装 VSCode安装 下载 安装 参考资料 VSCode安装 Windows版本:Windows10 VSCode版本:VScode1.65.0(64位User版本) 本文


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
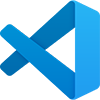
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
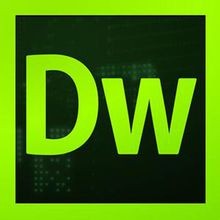
Dreamweaver CS6
Visual web development tools
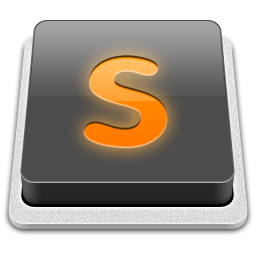
SublimeText3 Mac version
God-level code editing software (SublimeText3)