There are three ways to get the current date and time in JavaScript: use the Date object to provide date and time properties, such as date.getDate(), date.getMonth(), etc. Using the Date.now() method, returns the number of milliseconds since the epoch. Uses an ISO standard format string containing information such as year, month, date, hours, minutes, seconds, and millisecond time zone offset.
How to get the current date and time in JavaScript
In JavaScript, there are the following methods to get the current date and time:
1. Use Date object
Date
object represents a date and time. A new Date object can be created in the following way:
const date = new Date();
This object contains information about the current date and time, which can be accessed through the following properties:
-
date.getDate( )
: Returns the date of the current date (1-31) -
date.getMonth()
: Returns the month of the current date (0-11) -
date.getFullYear()
: Returns the year of the current date -
date.getHours()
: Returns the hour of the current time -
date. getMinutes()
: Returns the minutes of the current time -
date.getSeconds()
: Returns the seconds of the current time -
date.getMilliseconds()
: Return the milliseconds of the current time
2. Use the Date.now() method
Date.now()
method to return the elapsed time since the epoch Number of milliseconds. It can be used in the following ways:
const msSinceEpoch = Date.now();
3. Using ISO standard format strings
Date
objects in JavaScript can be converted to ISO 8601 standard format strings. The string contains the following information:
- Year
- Month
- Date
- Hour
- Minute
- Seconds
- Milliseconds
- Time zone offset
The ISO format string can be obtained using the following syntax:
const isoString = date.toISOString();
Example
Sample code to get the current date and time:
const date = new Date(); const fullDate = `${date.getDate()}/${date.getMonth() + 1}/${date.getFullYear()}`; const fullTime = `${date.getHours()}:${date.getMinutes()}:${date.getSeconds()}`; console.log(`Current date: ${fullDate}`); console.log(`Current time: ${fullTime}`);
Output:
<code>Current date: 31/12/2023 Current time: 12:00:00</code>
The above is the detailed content of How to get the current date and time in js. For more information, please follow other related articles on the PHP Chinese website!
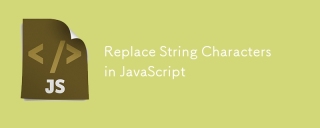
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
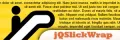
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
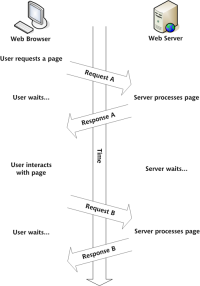
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
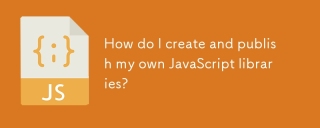
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
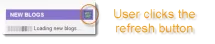
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
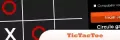
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
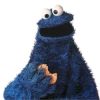
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session
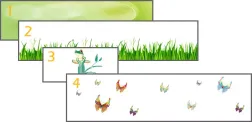
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
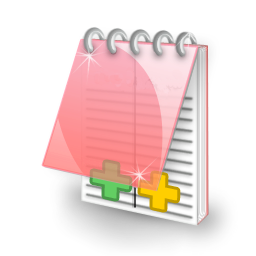
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
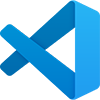
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
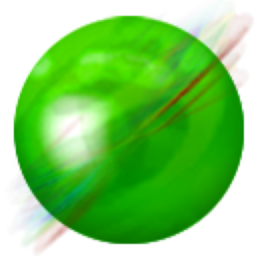
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
