


Handling edge cases of recursion in C++: Understanding recursion termination conditions
Handling edge cases in recursion is crucial. The following are the steps: Determine the basic situation: the conditions under which the recursion terminates and the result is returned. Return in base case: When the base case is met, the function returns the result immediately. Calling itself in recursive situations: When the base case is not satisfied, the function calls itself and keeps approaching the base case.
Boundary Case Handling of Recursion in C: Understanding Recursion Termination Conditions
Recursion is a programming technique that enables functions to Call itself. If edge cases are not handled appropriately, recursion can lead to stack overflow, where the program attempts to allocate more memory than is available. Edge cases are situations where a recursive function terminates and returns a result instead of continuing to call itself.
Understanding edge cases is crucial to writing effective recursive functions. Here are the general steps for handling edge cases:
- Determine the base case: Determine when the function should stop recursion and return a result. This is usually a simple condition, such as reaching a specific value or processing the last element in a data structure.
- Return in base case: When the base case is met, the function should return the result immediately. This will prevent it from continuing to recurse.
- Calling itself in recursive cases: When the base case is not satisfied, the function should call itself and provide parameters that continuously approximate the base case.
Practical case: Calculating factorial
The factorial is the cumulative product of positive integers until 1. For example, the factorial of 5 (noted 5!) is 120, calculated as: 5! = 5 × 4 × 3 × 2 × 1 = 120.
We can use a recursive function to calculate the factorial:
int factorial(int n) { // 基本情况:当 n 为 0 或 1 时返回 1 if (n == 0 || n == 1) { return 1; } // 递归情况:调用自身并传入减小的参数 else { return n * factorial(n - 1); } }
In this example, the base case is that when n
is 0 or 1, the function returns 1. For all other values, the function calls itself with decreasing arguments, continually approaching the base case, eventually causing the recursion to terminate.
The above is the detailed content of Handling edge cases of recursion in C++: Understanding recursion termination conditions. For more information, please follow other related articles on the PHP Chinese website!
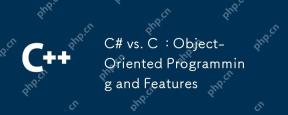
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
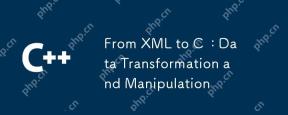
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
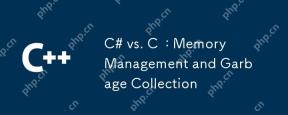
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
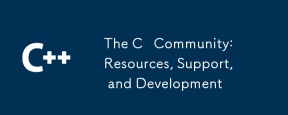
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
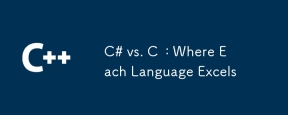
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
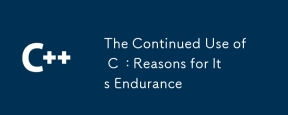
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
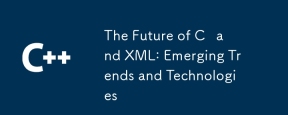
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
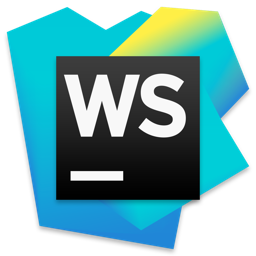
WebStorm Mac version
Useful JavaScript development tools
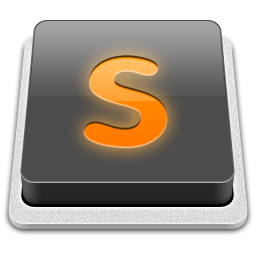
SublimeText3 Mac version
God-level code editing software (SublimeText3)