How to avoid stack overflow from recursive calls in Java functions?
How to avoid stack overflow caused by recursive calls in Java functions? Use loops instead of recursion. Avoid deep recursion. Use tail recursion. Set stack size limit.
Avoid stack overflow of recursive calls in Java functions
Recursive functions are very useful in Java, but if used improperly, they may will cause a stack overflow error. A stack overflow occurs when the number of function calls becomes too large, exhausting available memory.
How stack overflow occurs
When a function recurses, it creates new stack frames. Each stack frame contains the function's local variables and return address. If a function recurses too many times, the number of stack frames exceeds available memory, causing a stack overflow.
Tips to avoid stack overflow
Here are some tips to avoid stack overflow in recursive calls in Java functions:
- Use loops instead of recursion: When possible, consider using loops instead of recursion. The loop does not create a new stack frame and therefore cannot cause a stack overflow.
- Avoid deep recursion: Limit the depth of the recursive call stack. If you can, break the recursive function into smaller, more manageable parts.
- Use tail recursion: Tail recursion means that the last step of the recursive function is to call itself. The Java compiler can optimize tail recursion to avoid creating new stack frames.
- Set stack size limit: You can limit the stack size of the Java Virtual Machine (JVM) by setting the -Xss option. This prevents exhausting available memory before the stack overflows.
Practical case
Consider the following recursive function for calculating Fibonacci numbers:
public static int fib(int n) { if (n <= 1) { return n; } else { return fib(n - 1) + fib(n - 2); } }
This function is too recursive, for For larger n values, it causes stack overflow. To avoid this, we can use a loop instead of recursion:
public static int fib(int n) { int a = 0; int b = 1; for (int i = 0; i < n; i++) { int temp = a; a = b; b = temp + b; } return a; }
This loop version does not create a new stack frame, so it will not cause a stack overflow.
The above is the detailed content of How to avoid stack overflow from recursive calls in Java functions?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
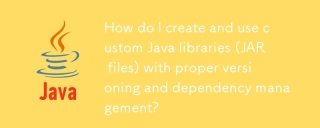
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
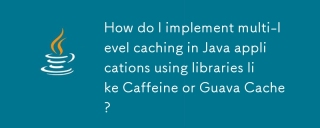
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
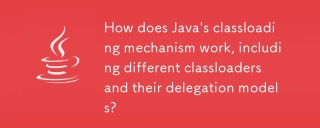
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
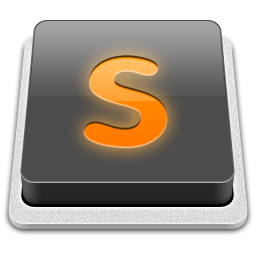
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.