By using caching technology, Java function performance can be effectively improved: caching technology reduces calls to underlying storage by storing recently accessed data. Caching libraries available in Java include Caffeine, Guava, and Ehcache. Caffeine is suitable for high-concurrency applications, Guava provides easy cache creation, and Ehcache is suitable for large applications that require scalable cache. Using Caffeine caching, you can significantly improve application performance by reducing the number of database accesses.
How to use caching technology to improve the performance of Java functions
Caching technology is an effective strategy that can be used to store recently accessed data to improve the performance of Java functions, thereby reducing access to the underlying storage. In Java, various caching libraries are available such as Caffeine, Guava, and Ehcache.
Caffeine
Caffeine is a high-performance, thread-safe cache library ideal for applications requiring high concurrency. It provides various caching strategies, such as:
- Strong reference cache: always stores the value, even if it is not accessed.
- Soft reference cache: Values can be cleared when the JVM encounters memory limits.
- Weak reference cache: The value will be cleared at the next GC.
Caffeine<String, String> cache = Caffeine.newBuilder() .expireAfterWrite(10, TimeUnit.MINUTES) .build(); String value = cache.getIfPresent("key"); if (value == null) { // 从数据库获取值 value = loadFromDB("key"); cache.put("key", value); }
Guava
Guava is also a popular caching library that provides an easy way to create caches. Guava has fewer caching features than Caffeine, but it's easier to use.
CacheBuilder<String, String> cache = CacheBuilder.newBuilder() .expireAfterWrite(10, TimeUnit.MINUTES) .build(); String value = cache.getIfPresent("key"); if (value == null) { // 从数据库获取值 value = loadFromDB("key"); cache.put("key", value); }
Ehcache
Ehcache is an enterprise-level caching library that provides various features such as persistence, distributed support, and off-heap memory. It is suitable for large applications that require a scalable caching solution.
CacheManager cacheManager = new CacheManager(); Cache cache = cacheManager.getCache("myCache"); String value = cache.get("key"); if (value == null) { // 从数据库获取值 value = loadFromDB("key"); cache.put("key", value); }
Practical case
The following is a simple Java function that uses Caffeine cache to improve performance:
import com.github.benmanes.caffeine.cache.Caffeine; public class CachingJavaFunction { private static Caffeine<String, String> cache = Caffeine.newBuilder() .expireAfterWrite(10, TimeUnit.MINUTES) .build(); public static String getCachedValue(String key) { String value = cache.getIfPresent(key); if (value == null) { // 从数据库获取值 value = loadFromDB(key); cache.put(key, value); } return value; } }
Using this function, you can Database accesses are reduced to a minimum, significantly improving application performance.
The above is the detailed content of How to use caching technology to improve the performance of Java functions?. For more information, please follow other related articles on the PHP Chinese website!
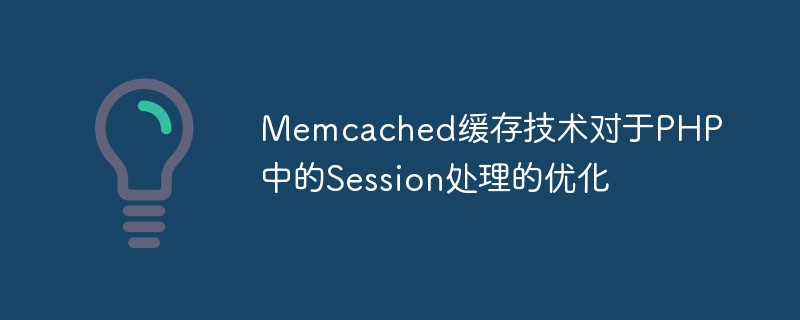
Memcached是一种常用的缓存技术,它可以使Web应用程序的性能得到很大的提升。在PHP中,常用的Session处理方式是将Session文件存放在服务器的硬盘上。但是,这种方式并不是最优的,因为服务器的硬盘会成为性能瓶颈之一。而使用Memcached缓存技术可以对PHP中的Session处理进行优化,提高Web应用程序的性能。PHP中的Session处
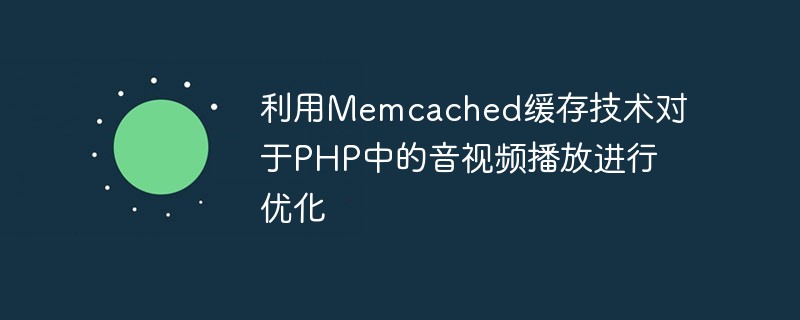
随着互联网技术的不断发展,音视频资源已经成为了互联网上非常重要的一种内容形式,而PHP作为网络开发中使用最广泛的语言之一,也在不断地应用于视频和音频播放领域。然而,随着音视频网站的用户日益增加,许多网站已经发现了一个问题:在高并发的情况下,PHP对于音视频的处理速度明显变缓,会导致无法及时播放或者播放卡顿等问题。为了解决这个问题,Memcached缓存技术应
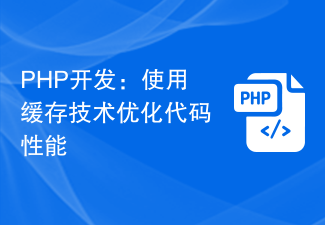
PHP是一种脚本语言,常用于Web应用程序开发。随着互联网的迅速发展,Web应用程序的开发也变得越来越复杂,代码量越来越大。因此,优化代码性能变得尤为重要,在这方面,使用缓存技术是一种行之有效的方式。在本文中,我们将探讨PHP开发中使用缓存技术优化代码性能的方法和技巧。什么是缓存技术?首先,让我们了解一下什么是缓存技术。在计算机中,缓存是一种临时存储数据的技
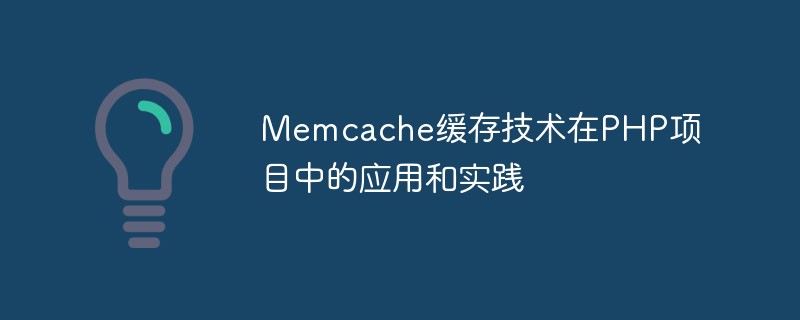
Memcache是一种开源的、分布式的缓存技术。它通过将数据存储在内存中,极大地提高了数据的访问速度,从而提升了网站的性能和响应速度。在PHP项目中,Memcache缓存技术也被广泛应用,并且取得了很好的效果。本篇文章将深入探讨Memcache缓存技术在PHP项目中的应用和实践。一、Memcache的原理和优势Memcache是一种内存缓存技术,它可以将数据
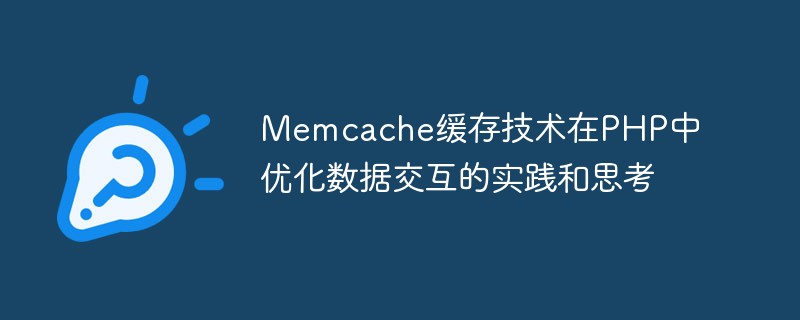
Memcache缓存技术在PHP中优化数据交互的实践和思考在现代的Web应用中,数据交互是一个非常重要的问题,它没有足够的高效性,将会限制Web应用程序的扩展性和性能。为了加快数据交互速度,我们通常的做法是优化数据库的设计、提高硬件的性能和增加服务器容量。但是,这些方法都有一个共同的限制:它们会增加系统的成本。最近几年,Memcache技术在解决这个问题上提
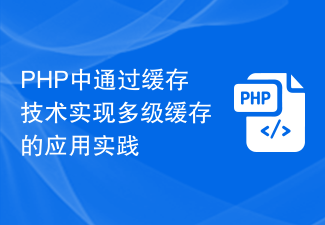
随着互联网的迅速发展,大量应用和网站需要处理大量的数据和请求。为了提高响应速度和减轻服务器负载,使用缓存技术已经成为了常态。而在PHP中,通过缓存技术实现多级缓存已成为一种重要的优化手段。本文将介绍PHP中多级缓存的应用实践,具体包括以下内容:什么是缓存技术缓存技术的三种常见应用场景多级缓存的原理和应用在PHP中使用多级缓存的具体实现总结什么是缓存技术缓存技
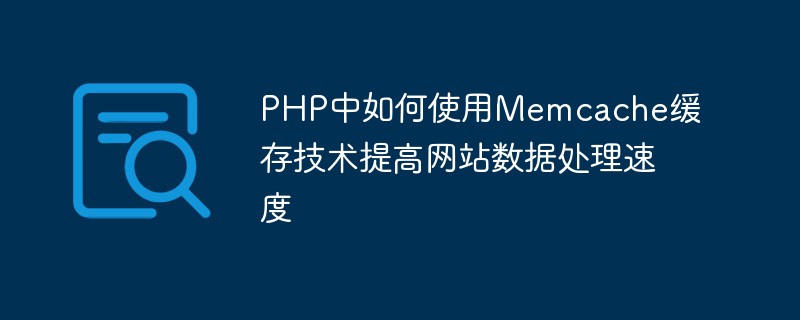
随着互联网用户数量的快速增长,网站数据处理速度愈发成为了一个核心问题。Memcache以其高性能和低延迟的优点成为了网站缓存技术中的佼佼者。今天本文就会带你一步一步了解PHP中如何使用Memcache缓存技术来提高网站数据处理速度。Memcache基础知识Memcache是一个高性能的分布式内存对象缓存系统。它可以减少数据库在处理高并发访问时的压力,提高网站
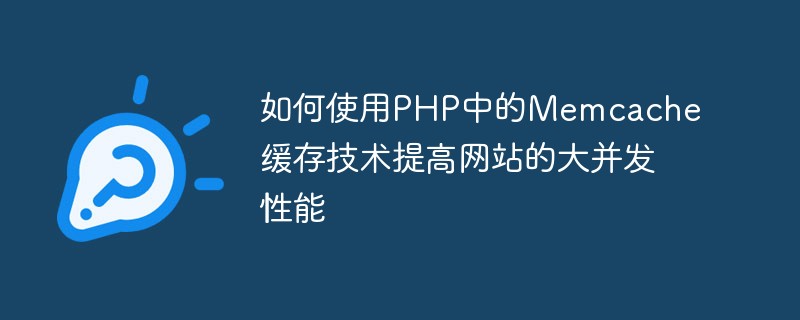
随着互联网技术的不断发展,网站的用户访问量越来越大,带来的并发访问量也越来越高。为了应对这种高并发访问,常用的手段是使用缓存技术。而在PHP语言中,Memcache缓存技术是一种非常有效的解决方案。Memcache是一种分布式缓存系统,能够将大量的数据缓存在内存中,并能够从内存中快速读取,从而提高网站的响应速度和并发能力。在本文中,我们将介绍如何使用PHP中


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
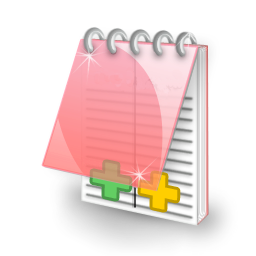
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
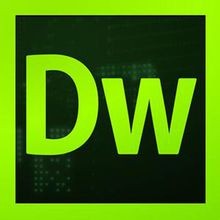
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
