


Detailed explanation of C++ member functions: generic programming and templating of object methods
C Member functions are functions defined within a class and can access data members and variables of the class. Generic programming and templating make code reusable and independent of data types. Generic programming allows writing code that works with different data types, while templating allows creating classes that work with member functions of different types. For programs that need to calculate the areas of different shapes, you can create an AreaCalculator class using templated member functions and provide subclasses that implement the calculateArea member function for each shape type, such as Rectangle and Circle. This class makes it easy to calculate the area of various shapes.
Detailed explanation of C member functions: Generic programming and templating of object methods
In C, member functions are defined in Functions within a class can access the data members and member variables of the class. Generic programming and templating are powerful techniques in C for writing code that is reusable and independent of data types.
Generic Programming
Generic code allows you to write functions that work with different data types. It eliminates the need to write separate functions for each data type.
template<typename T> T max(T a, T b) { return a > b ? a : b; }
The max
function can be used with any data type T
.
Templating
Templating allows you to create classes that can be used with different types of member functions. By using templates, you avoid writing duplicate code for each member function.
template<typename T> class Vector { public: Vector() : x(0), y(0), z(0) {} Vector(T x, T y, T z) : x(x), y(y), z(z) {} T x, y, z; };
This Vector
class can store any type of value.
Practical Case
Suppose you want to create a program that calculates the area of various shapes. You can use templated member functions to create AreaCalculator
classes that can be used for different shape types.
template<typename Shape> class AreaCalculator { public: double area(const Shape& shape) { // 根据形状类型计算面积 return shape.calculateArea(); } };
For each shape type, you need to provide a Shape
subclass that implements the calculateArea
member function.
class Rectangle { public: double width, height; double calculateArea() { return width * height; } }; class Circle { public: double radius; double calculateArea() { return PI * radius * radius; } };
Now you can calculate the area of any shape using AreaCalculator
:
AreaCalculator<Rectangle> rectangleCalculator; double rectangleArea = rectangleCalculator.area(Rectangle{3.0, 4.0}); AreaCalculator<Circle> circleCalculator; double circleArea = circleCalculator.area(Circle{2.0});
The above is the detailed content of Detailed explanation of C++ member functions: generic programming and templating of object methods. For more information, please follow other related articles on the PHP Chinese website!
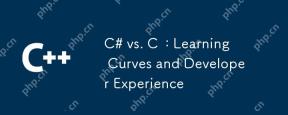
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
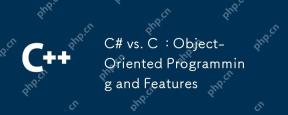
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
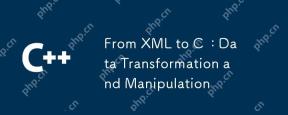
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
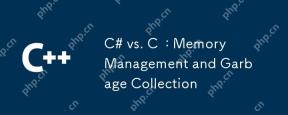
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
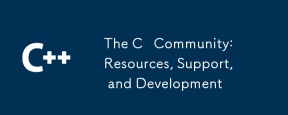
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
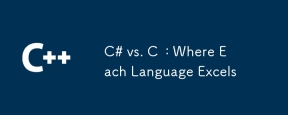
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
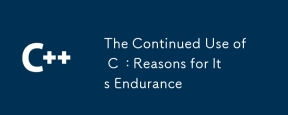
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
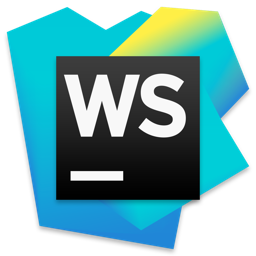
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor