


Function pointers allow storing function addresses in C, but lack type safety. To enhance safety, C 11 introduced typed callbacks, allowing the signature of a function pointer to be specified. Additionally, type-unsafe function pointer conversions can lead to undefined behavior. Programmers can balance convenience with safety by using type-safe callbacks and careful function pointer conversions.
C Function Pointers and Type Safety: An In-depth Exploration of the Delicate Balance
Introduction
In C, a function pointer is a A concise and efficient way to store function addresses in variables and call functions. However, function pointers introduce type safety hazards because they allow function pointers to be used incorrectly for unintended types.この记事 will delve into the balance between function pointers and type safety, and provide practical examples to illustrate this concept.
Function pointer
C A function pointer is a pointer pointing to a function address. They are declared with type T*
, where T
is the signature of the function. For example, a pointer to a function that accepts int
arguments and returns an int
value is declared int (*)(int)
.
int add(int a, int b) { return a + b; } int (*fp)(int, int) = &add; // 函数指针指向 add 函数
Type Safety Hazards
Function pointers lack type safety because they allow conversion between different types. For example, we can cast a pointer of type int (*)(int, int)
to type double (*)(double, double)
even though this may result in undefined Behavior.
double (*dp)(double, double) = (double (*)(double, double))fp; double result = dp(1.5, 2.3); // 可能导致未定义行为
Type safety enhancements
To enhance the type safety of function pointers, C 11 introduces typed callbacks, which allow programmers to specify the signature of function pointers. Typed callbacks are declared with the auto
keyword and the function signature is defined using the ->
operator.
auto fp = [](int a, int b) -> int { return a + b; }; // 类型化回调 // ...调用 fp ...
Typed callbacks ensure that function pointers are only used for their expected types, thus improving type safety.
Practical case
Type-safe callback
The following example shows how to use function pointers in type-safe callbacks:
struct Rectangle { int width, height; int area() { return width * height; } }; void printArea(auto fn) { std::cout << "Area: " << fn() << std::endl; } int main() { Rectangle rect{5, 3}; auto rectArea = [](Rectangle& r) -> int { return r.area(); }; // 类型安全回调 printArea(rectArea); // 输出: Area: 15 }
Type-unsafe function pointer conversion
The following example demonstrates the potential harm of type-unsafe function pointer conversions:
int sum(int a, int b) { return a + b; } double subtract(double a, double b) { return a - b; } int (*fp)(int, int) = ∑ fp(1, 2); // 正确执行 double (*dp)(int, int) = (double (*)(int, int))fp; // 类型不安全的转换 dp(1, 2); // 导致未定义行为
Conclusion
Function pointers provide flexibility in C, but they can also bring to type safety hazards. The introduction of typed callbacks enhances type safety, allowing the programmer to specify the expected type of a function pointer. By carefully considering the use of function pointers and taking advantage of type safety measures, programmers can balance the convenience and safety of function pointers.
The above is the detailed content of C++ Function Pointers and Type Safety: A Deeper Exploration of the Balance. For more information, please follow other related articles on the PHP Chinese website!
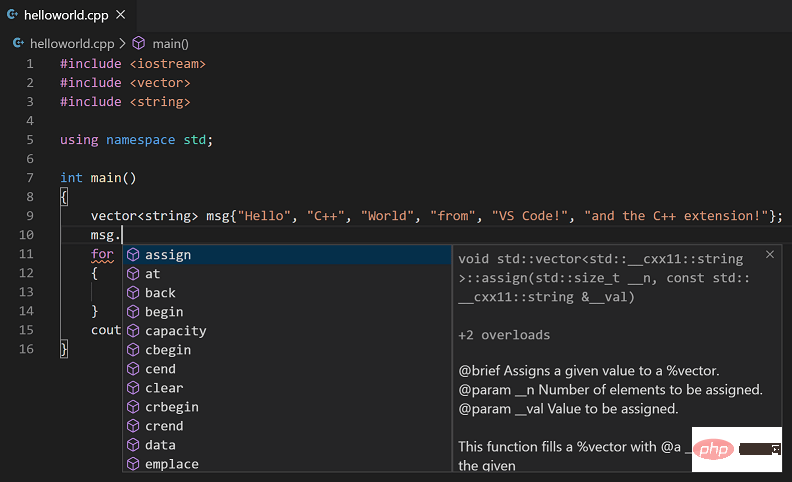
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
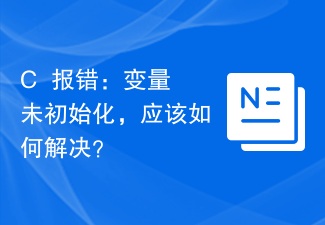
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
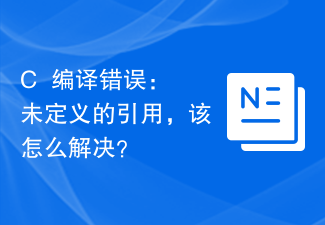
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
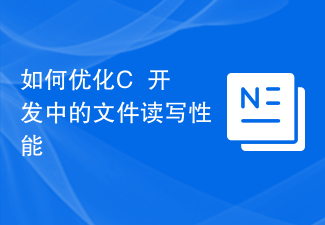
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
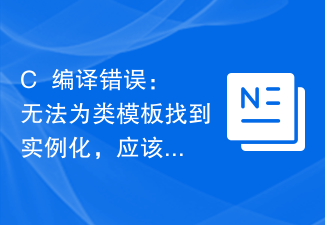
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
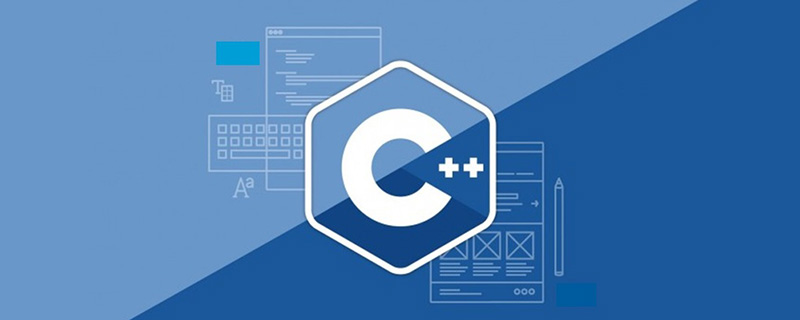
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
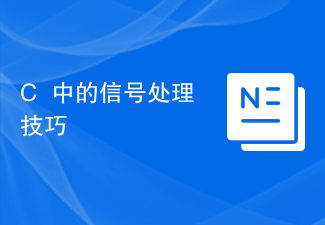
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以
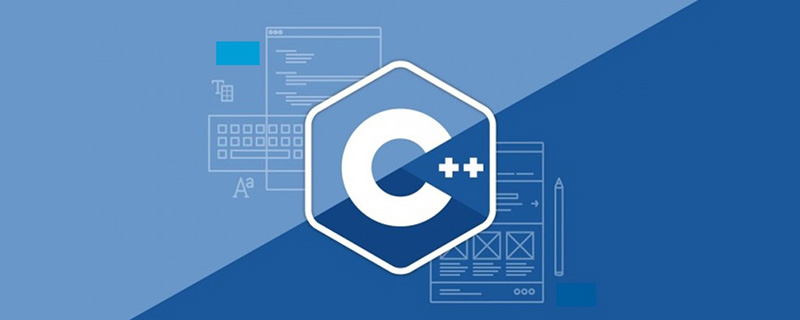
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
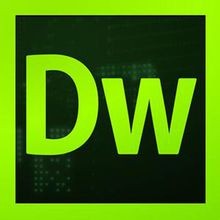
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
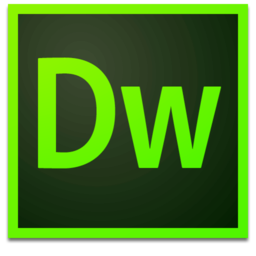
Dreamweaver Mac version
Visual web development tools