


Practical application of C++ virtual functions: code examples and troubleshooting
Virtual functions allow overriding base class functions in derived classes and calling appropriate functions at runtime based on the object type: Create virtual functions: Use the virtual keyword in the base class to declare functions. Override virtual functions: Use the override keyword in derived classes to override base class virtual functions. Actual call: When a derived class object is pointed to through a base class pointer or reference, the overridden version will be called. Uncovered virtual function: If not overridden, the base class original implementation will be called. Call using pointers or references: Implement polymorphism, allowing a pointer or reference from a base class to point to a derived class object, and call the appropriate overriding function at runtime.
Practical application of C virtual functions: code examples and troubleshooting
Introduction to virtual functions
Virtual functions are a type of polymorphism in C mechanism. It allows functions in the base class to be overridden in the derived class, allowing the appropriate function to be called at runtime based on the actual type of the object.
Create a virtual function
To create a virtual function, you need to declare the function in the base class using the virtual
keyword, as follows:
class Base { public: virtual void print() { cout << "Base class" << endl; } };
Override virtual functions
In derived classes, you can use the override
keyword to override virtual functions in the base class, as shown below:
class Derived : public Base { public: override void print() { cout << "Derived class" << endl; } };
Practical case
Consider the following simple example:
#include <iostream> using namespace std; class Animal { public: virtual void speak() { cout << "Animal speaks" << endl; } }; class Dog : public Animal { public: override void speak() { cout << "Dog barks" << endl; } }; int main() { Animal* a = new Dog(); a->speak(); // 输出:Dog barks return 0; }
In this example, the base class Animal
defines the speak()
virtual function, which is used in Dog
Overridden in derived classes. When we call the speak()
function via the Animal*
pointer, the overridden version is called, outputting "Dog barks".
Question-solving
Q: Why do I need to use pointers or references to call virtual functions?
Answer: To achieve polymorphism, a pointer or reference from a base class can point to an object of a derived class, allowing the appropriate overriding function to be called at runtime.
Q: What happens if the virtual function is not overridden in the derived class?
Answer: In this case, the original implementation in the base class will be called.
The above is the detailed content of Practical application of C++ virtual functions: code examples and troubleshooting. For more information, please follow other related articles on the PHP Chinese website!
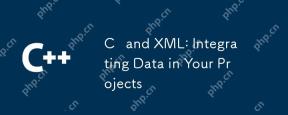
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
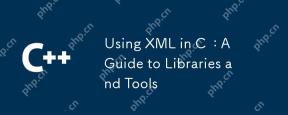
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
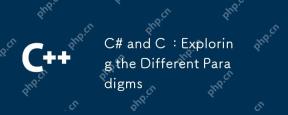
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
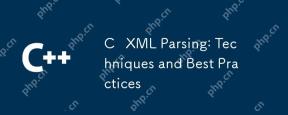
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
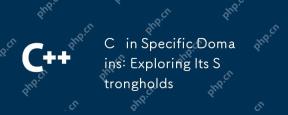
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
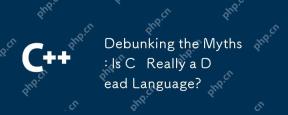
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
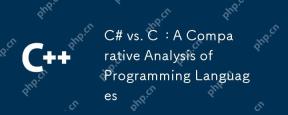
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
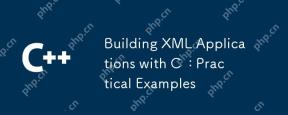
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
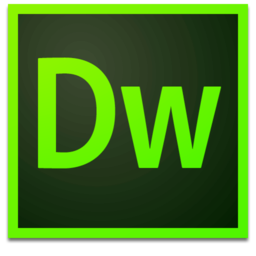
Dreamweaver Mac version
Visual web development tools
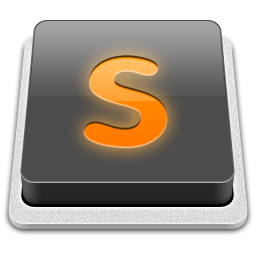
SublimeText3 Mac version
God-level code editing software (SublimeText3)
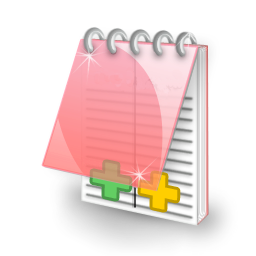
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
