Inline functions are a C feature that replaces function code directly at the call site, thereby optimizing performance. Best practices include using inlining sparingly and only for small, frequently called functions. Avoid recursion and loops as they increase function size and complexity. Keep inline functions small, usually no more than 5-10 lines. Consider inline bloat as it may increase application size. Disable inlining in debug mode to simplify debugging.
Best Practice Tips for C Inline Functions
Introduction
Inside A link function is a small function in C that is recognized by the compiler and directly replaced by the actual function calling code. It inlines function code to the call site, eliminating the overhead of function calls and increasing execution speed. However, misuse of inline functions can also have negative consequences.
Best Practice Tips
-
Use the inline keyword with caution: Only for really tiny, frequently called functions Add inline.
inline int square(int x) { return x * x; }
-
Avoid recursion and loops: Recursion and loops will increase the size and complexity of inline functions.
// 避免 inline int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } }
-
Be careful about function size: Inline functions should be kept small (~5-10 lines). Larger functions will result in code bloat and longer compilation times.
// 超过 10 行 inline int calculate_average(int arr[], int size) { int sum = 0; for (int i = 0; i < size; i++) { sum += arr[i]; } return sum / size; }
- Consider inline bloat: Inline functions lead to code duplication, which may increase application size. In space-constrained environments, this can be a problem.
- Disable inlining for Debug mode: In debug mode, disable inlining for easier debugging as the line numbers will point to the actual function calls.
Practical Case
The following is an example of using inline functions in a real application:
#include <iostream> // 计算 x 的平方以内联 inline int square(int x) { return x * x; } // 使用内联函数优化绘图循环 void draw_circle(int cx, int cy, int radius) { for (int x = cx - radius; x <= cx + radius; x++) { for (int y = cy - radius; y <= cy + radius; y++) { if (square(x - cx) + square(y - cy) <= square(radius)) { // 绘制圆的当前像素 } } } } int main() { // 调用内联函数计算平方 std::cout << square(5) << std::endl; // 使用内联函数优化绘图循环 draw_circle(200, 200, 50); }
Conclusion
Following these best practice tips can help you make effective use of inline functions in C, thereby improving performance and optimizing your code. It is important to use caution and weigh the pros and cons on a case-by-case basis.
The above is the detailed content of Sharing of best practice tips for C++ inline functions. For more information, please follow other related articles on the PHP Chinese website!
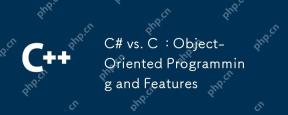
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
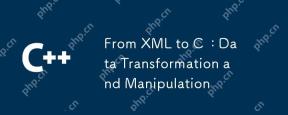
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
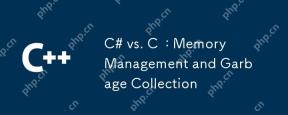
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
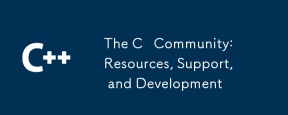
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
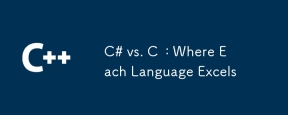
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
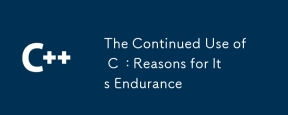
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
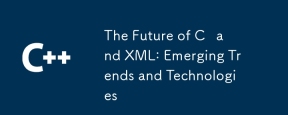
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
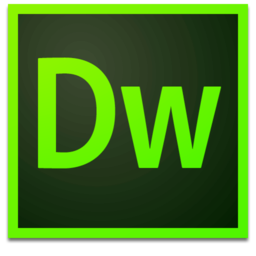
Dreamweaver Mac version
Visual web development tools
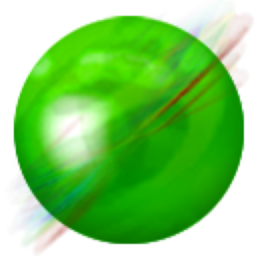
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment