Cin in C can be combined with the database through a database interface library (such as MySQL Connector/C or ODBC). Specific steps include: installing the database interface library; establishing a database connection; creating a query statement; binding the cin input to the query parameters; executing the query; and obtaining the query results.
The combination of cin and database in C
Use cin in C to read user input from the command line. And database is used to store and manage data. To combine cin with a database, you need to use a database interface library (such as MySQL Connector/C or ODBC).
Using MySQL Connector/C
- Install the MySQL Connector/C library.
-
Include necessary header files in your C code.
#include <iostream> #include <mysqlx/xdevapi.h>
-
Establish a database connection.
mysqlx::Session session("host", "port", "user", "password", "database");
-
Create query statement.
std::string query = "SELECT * FROM table_name WHERE column_name = ?";
-
Bind cin input to query parameters.
mysqlx::PreparedStatement stmt = session.prepare(query); std::string input; std::cin >> input; stmt.bind("column_name", input);
-
Execute the query.
mysqlx::Result res = stmt.execute();
-
Get query results.
for (auto row : res.fetchAll()) { std::cout << row[0].get<std::string>() << std::endl; }
Using ODBC
-
Include the necessary ODBC header files.
#include <iostream> #include <sql.h> #include <sqlext.h>
-
Establish a database connection.
SQLHENV henv; SQLHDBC hdbc; SQLAllocEnv(&henv); SQLAllocConnect(henv, &hdbc); SQLDriverConnect(hdbc, nullptr, "DSN", SQL_NTS, nullptr, 0, nullptr, SQL_DRIVER_NOPROMPT);
-
Create a SQL statement handle.
SQLHSTMT hstmt; SQLAllocStmt(hdbc, &hstmt);
-
Set SQL statement.
std::string sql = "SELECT * FROM table_name WHERE column_name = ?"; SQLBindParameter(hstmt, 1, SQL_PARAM_INPUT, SQL_C_CHAR, SQL_CHAR, 0, 0, nullptr, 0, nullptr);
-
Bind cin input to a SQL statement.
std::string input; std::cin >> input; SQLSetParam(hstmt, 1, SQL_C_CHAR, input.c_str(), input.length(), nullptr);
-
Execute SQL statement.
SQLExecute(hstmt);
-
Get query results.
SQLBindCol(hstmt, 1, SQL_C_CHAR, nullptr, 0, nullptr); while (SQLFetch(hstmt) == SQL_SUCCESS) { char buffer[1024]; SQLGetData(hstmt, 1, SQL_C_CHAR, buffer, sizeof(buffer), nullptr); std::cout << buffer << std::endl; }
The above is the detailed content of How to combine cin and database in c++. For more information, please follow other related articles on the PHP Chinese website!
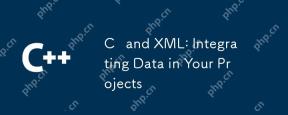
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
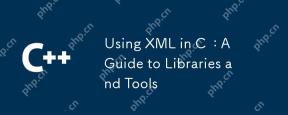
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
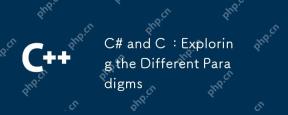
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
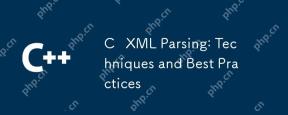
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
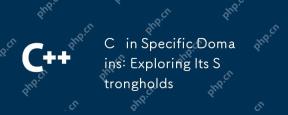
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
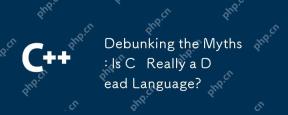
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
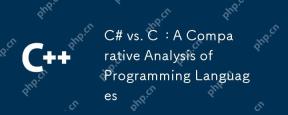
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
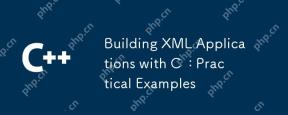
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
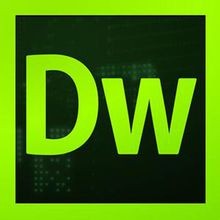
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
