In order to automate unit testing of Java functions, you need to write test cases using a testing framework (such as JUnit) and leverage assertions and simulations (such as Mockito) to verify the results. Specific steps include: Set up JUnit dependencies Create a dedicated test class and extend TestCase Use the @Test annotation to identify test methods Use assertions to verify test results Use mocks to avoid using actual dependencies
How to Automate Unit Testing of Java Functions
Automated unit testing is a fast and reliable way to verify how your code works. Automated unit testing of Java functions can be easily done by using the right frameworks and technologies.
Requirements
- Java Development Kit (JDK)
- Testing framework (such as JUnit)
- To run tests IDE (such as IntelliJ IDEA or Eclipse)
JUnit settings
JUnit is a popular Java unit testing framework. It provides a simple API for creating and running tests. To use JUnit, add the following dependencies to your project:
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency>
Writing Test Cases
Creating a test case involves writing a specialized class for the function to be tested. This class can extend JUnit's TestCase
class and use the @Test
annotation to identify the test method:
import org.junit.Test; public class MyFunctionTest { @Test public void testMyFunction() { // ... } }
Assertion and mocking
Use assertions to verify whether the test results meet expectations. JUnit provides a set of built-in assertion methods, such as assertEquals
, assertTrue
and assertFalse
.
Mocking allows the creation of fake objects in tests to avoid using real dependencies. Mockito is a popular Java mocking library that allows easy creation and verification of mock objects:
import org.mockito.Mockito; @Test public void testMyFunctionWithMock() { // 创建依赖项的模拟 MyDependency mockDependency = Mockito.mock(MyDependency.class); // 使用模拟的依赖项调用函数 myFunction(mockDependency); // 验证模拟的依赖项被正确调用 Mockito.verify(mockDependency).doSomething(); }
Practical Case
Suppose we have a function that calculates the sum of two numbers Function calculateSum
:
public class MathUtils { public static int calculateSum(int a, int b) { return a + b; } }
Let’s write a unit test to verify this function:
import org.junit.Test; public class MathUtilsTest { @Test public void testCalculateSum() { // 计算预期结果 int expectedSum = 10; // 调用函数 int actualSum = MathUtils.calculateSum(5, 5); // 验证结果 assertEquals(expectedSum, actualSum); } }
Run the test
in the IDE or use the mvn test
command to run the test. Tests that run successfully print nothing, while tests that fail print an error message.
The above is the detailed content of How to automate unit testing of Java functions?. For more information, please follow other related articles on the PHP Chinese website!
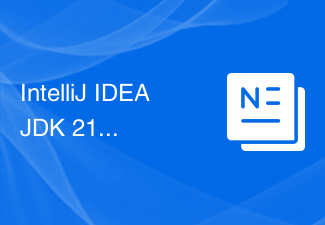
当我尝试使用java.util.concurrent中的timeunit类时遇到问题。它发生在oraclejdk21.0.1(配置如下)+intellijidea2023.1.5(社区版)-当前最新更新(更新:正如我一开始所想的,因为我运行了更新-请参阅下面的解决方案)。我的计算机上的配置(通过控制台中的java-version获取):javaversion"21.0.1"2023-10-17ltsjava(tm)seruntimeenvironme
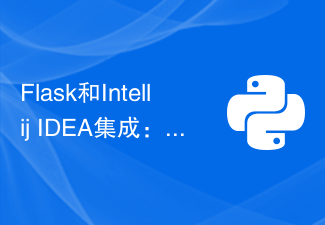
在第一部分介绍了基本的Flask和IntellijIDEA集成、项目和虚拟环境的设置、依赖安装等方面的内容。接下来我们将继续探讨更多的Pythonweb应用程序开发技巧,构建更高效的工作环境:使用FlaskBlueprintsFlaskBlueprints允许您组织应用程序代码以便于管理和维护。Blueprint是一个Python模块,能够包
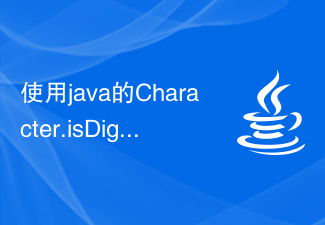
使用Java的Character.isDigit()函数判断字符是否为数字字符在计算机内部以ASCII码的形式表示,每个字符都有一个对应的ASCII码。其中,数字字符0到9分别对应的ASCII码值为48到57。要判断一个字符是否为数字,可以使用Java中的Character类提供的isDigit()方法进行判断。isDigit()方法是Character类的
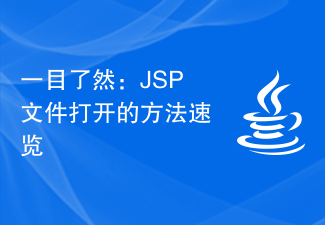
JSP文件打开方式JSP(JavaServerPages)是一种动态网页技术,它允许程序员在HTML页面中嵌入Java代码。JSP文件是文本文件,其中包含HTML代码、XML标记和Java代码。当JSP文件被请求时,它会被编译成JavaServlet,然后由Web服务器执行。打开JSP文件的方法有几种方法可以打开JSP文件。最简单的方法是使用文本编辑器,
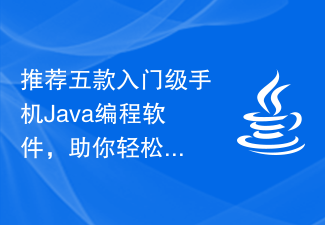
手机Java编程软件推荐:让你轻松入门的五款工具在这个数字化时代,手机已经成为了我们生活中不可或缺的一部分。无论是工作、学习还是娱乐,手机几乎可以满足我们所有的需求。而对于编程爱好者来说,手机也可以成为一个非常实用的工具,帮助他们随时随地进行代码编写与学习。本文将向大家推荐五款手机Java编程软件,让你轻松入门,享受编程的乐趣。AIDEAIDE是一款功能强大
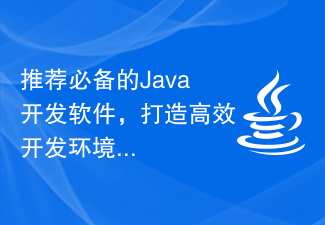
在今天的软件开发领域,Java作为一种广泛应用的编程语言,有着很高的开发效率和便捷性。为了提高开发效率,拥有一个优秀的Java编程环境是至关重要的。本文将为大家推荐几款必备的Java编程软件,帮助打造一个高效的开发环境。EclipseEclipse是一款功能强大且广泛使用的Java集成开发环境(IDE)。它提供了丰富的功能和插件,支持Java项目的开发、调试
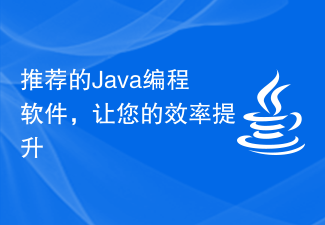
随着现代工作和生活的步伐不断加快,高效工作成为每个人追求的目标。而在信息技术领域,编程成为了提升效率的重要工具之一。在众多的编程语言中,Java作为一种广泛应用的高级编程语言,其编程软件更是成为提升效率的神器。本文就为大家推荐几款优秀的Java编程软件。首先要推荐的是Eclipse。作为Java开发者最常用的集成开发环境(IDE),Eclipse具备了强大的
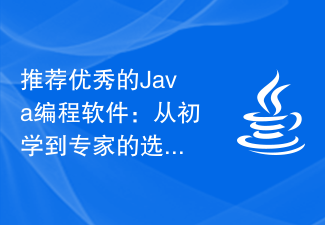
Java编程语言被广泛应用于各种软件开发领域,因其跨平台性能和可靠性而备受开发者青睐。然而,要进行Java编程,我们需要选择一款优秀的Java编程软件。本文就从入门到精通,推荐几款优秀的Java编程软件,帮助读者选择适合自己的工具。Eclipse(EclipseIDE)Eclipse是一个非常流行的开源集成开发环境(IDE)。它提供了强大的编辑器、调试器


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
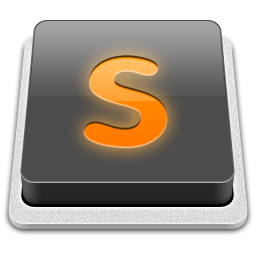
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
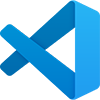
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
