This tutorial describes how to use Sentry to set up a Java function error monitoring and alerting system: create a Sentry account and integrate the Sentry SDK. Initialize Sentry and trap errors into Sentry. Set up alerts to monitor error count, error rate, and specific impact. An example of how to monitor database connection errors and set up alerts to receive notifications.
Settings for Java function error monitoring and alarm system
Introduction
Error Monitoring is critical to identifying and resolving issues in your application. This tutorial will guide you on how to set up a Java function error monitoring and alerting system to automatically detect and notify you of errors in your application.
Monitor Errors with Sentry
Sentry is a popular open source error monitoring service that provides a wide range of features, including automatic error capture, error grouping, alerts, and dashboards.
Set up Sentry
- Create a Sentry account: [https://sentry.io/](https://sentry.io/)
- Create a new project
- Go to your project settings and select the "Integrations" tab
- Select "Java" and follow the instructions to add the Sentry SDK
Integrate Sentry SDK
To integrate Sentry SDK in your Java function, you need to add the following dependencies:
<dependency> <groupId>io.sentry</groupId> <artifactId>sentry</artifactId> <version>5.1.2</version> </dependency>
Then, in your function class Initialize Sentry:
import io.sentry.Sentry; public class MyFunction { public static void main(String[] args) { // 初始化 Sentry Sentry.init("SENTRY_DSN"); // ... 你的函数逻辑 ... } }
Set Alerts
In the Sentry dashboard, go to the "Alerts" tab and create a new alert. You can set alert conditions, for example:
- Number of errors exceeds threshold
- Error rate exceeds expectations
- Affects specific environments or features
Using practical cases
Example: Monitoring database connection errors
In your Java function, you can use Sentry to capture database connection errors:
import io.sentry.Sentry; public class MyFunction { // ... public void connectToDatabase() { try { // ... 连接到数据库 ... } catch (Exception e) { // 捕获并记录数据库连接错误 Sentry.captureException(e); throw e; } } }
By setting up Sentry alerts, you will be notified when errors occur. This will enable you to quickly identify and resolve issues, thereby increasing the reliability and stability of your application.
The above is the detailed content of Setup of Java function error monitoring and alerting system. For more information, please follow other related articles on the PHP Chinese website!
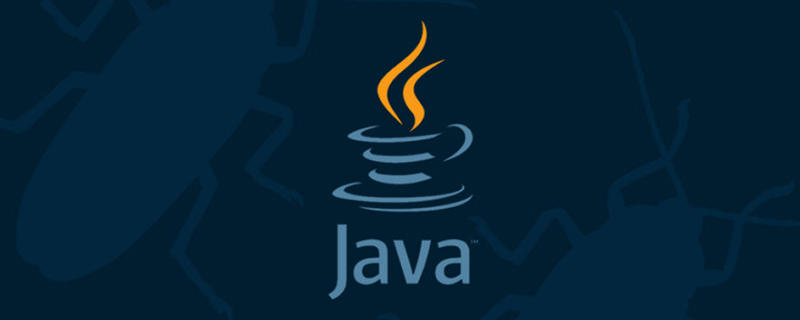
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
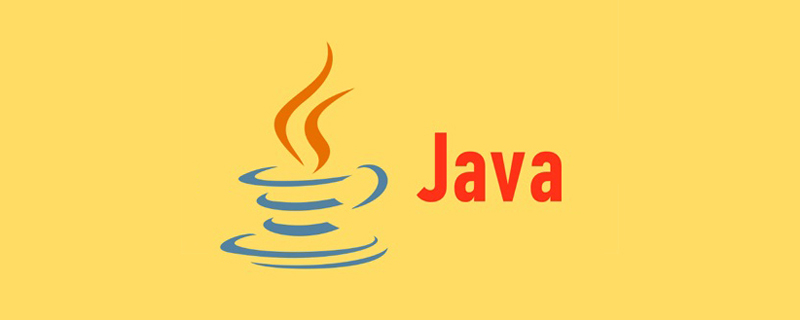
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
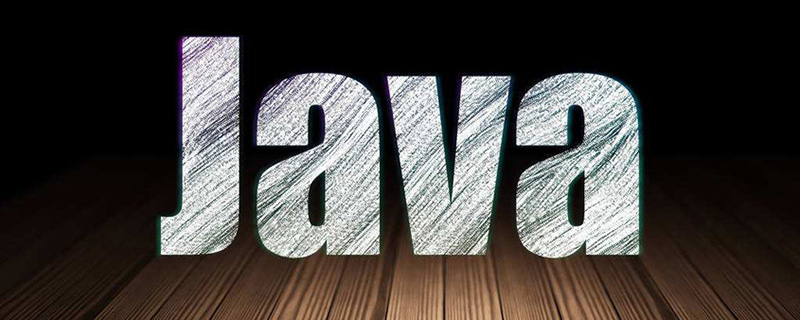
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
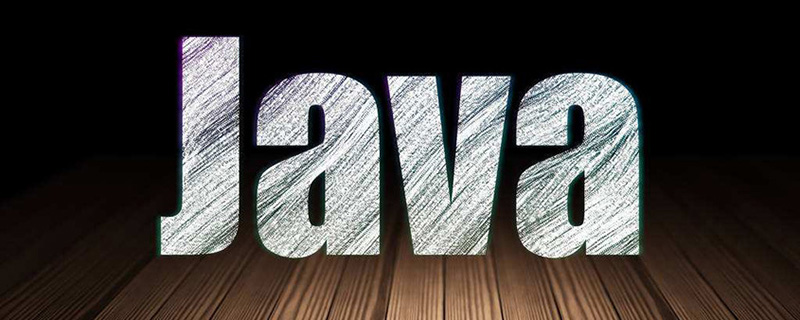
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
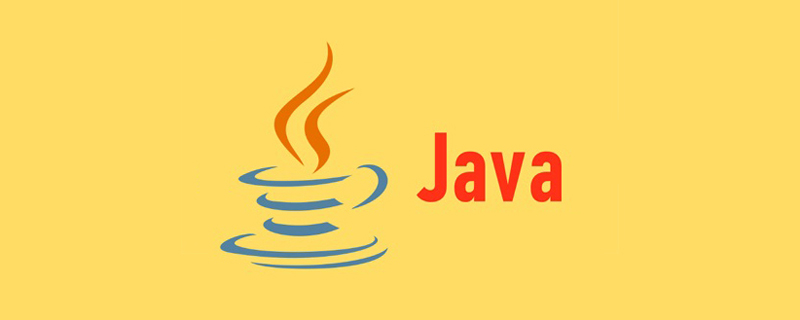
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
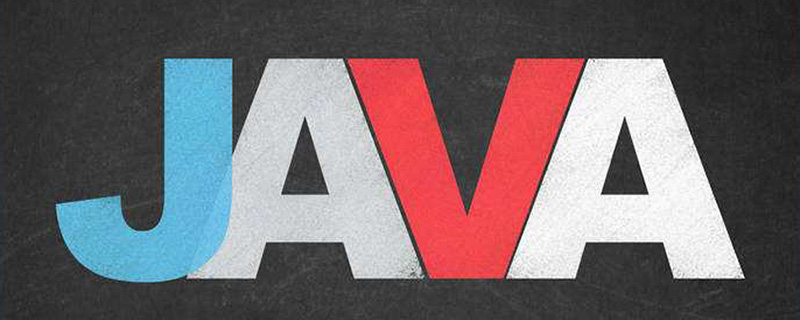
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
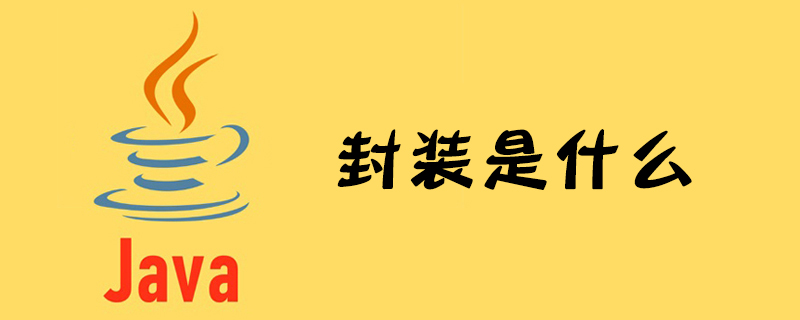
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
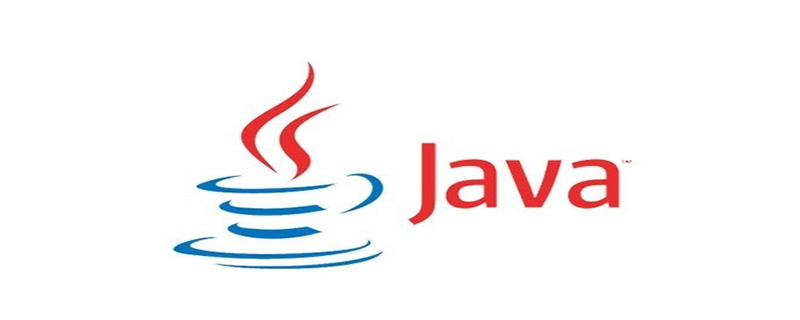
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
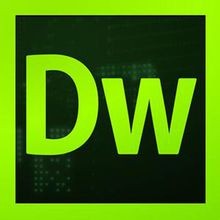
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
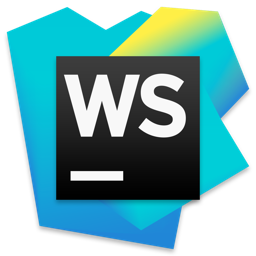
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
