


C Indefinite parameter passing: implemented through the ... operator, which accepts any number of additional parameters. The advantages include flexibility, scalability, and simplified code. The disadvantages include performance overhead, debugging difficulties, and type safety. Common practical examples include printf() and std::cout, which use va_list to handle a variable number of arguments.
C Detailed explanation of function parameters: the implementation and advantages and disadvantages of indefinite parameter passing
Indefinite parameter passing allows the function to accept an unknown number of parameter. It provides a flexible way to handle input lists without pre-defining parameter lists. In C, you can use the ...
syntax to implement variable parameter passing.
Implementation method
In C, you can use the ...
operator to implement indefinite parameter transfer. This operator is placed at the end of the argument list, indicating that the function can accept any number of additional arguments. These additional parameters are stored in std::initializer_list
.
The following code demonstrates how to use the ...
operator:
#include <initializer_list> void print_args(std::initializer_list<int> args) { for (int arg : args) { std::cout << arg << " "; } std::cout << std::endl; } int main() { print_args({}); // 空参数列表 print_args({1, 2, 3}); // 三个 int 值 print_args({1, 2.5, 3}); // 混合数据类型 return 0; }
Output:
(nothing) 1 2 3 1 2.5 3
Advantages
Indefinite parameter passing provides the following advantages:
- Flexibility: It allows the function to handle an unknown number of parameters, thereby improving the function's versatility and reusability.
- Extensibility: Functions can add or remove parameters as needed without having to change the function signature.
- Simplify code: Indefinite parameter passing can simplify the code for repeated tasks, such as traversing a list or array.
Disadvantages
Indefinite parameter passing also has some disadvantages:
- Performance overhead:Indefinite parameter passing Involves additional copying and memory allocation, which may impact performance.
- Debugging Difficulty: Because a varying number of arguments can be passed, it can be difficult to track the behavior of a function when debugging.
- Type safety: Indefinite parameter passing allows different types of data to be passed, which may lead to type-unsafe code.
Practical case
A common practical case of indefinite parameter passing is the function printf()
and std::cout
, they all allow passing an unlimited number of format specifiers and parameters. These functions use va_list
to obtain and process a variable number of arguments.
For example, the following code uses printf()
to print an indefinite number of integers:
#include <stdarg.h> // 头文件包含 va_list void print_ints(int count, ...) { va_list args; va_start(args, count); // 初始化 va_list for (int i = 0; i < count; i++) { int arg = va_arg(args, int); // 获取下一个参数 std::cout << arg << " "; } va_end(args); // 清理 va_list } int main() { print_ints(0); // 无参数 print_ints(3, 1, 2, 3); // 三个整数 return 0; }
Output:
(nothing) 1 2 3
The above is the detailed content of Detailed explanation of C++ function parameters: implementation methods, advantages and disadvantages of indefinite parameter passing. For more information, please follow other related articles on the PHP Chinese website!
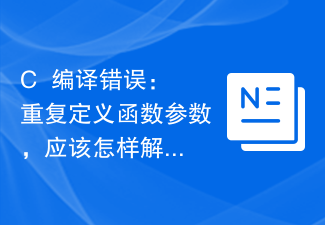
C++作为一种高效的编程语言,因其可靠性被广泛应用于各种各样的领域。但是,在编写代码的过程中,经常会遇到一些编译错误,其中重复定义函数参数就是其中之一。本文将详细介绍重复定义函数参数的原因和解决方案。什么是重复定义函数参数?在C++编程中,函数参数是指在函数定义和声明中出现的变量或表达式,用于接受函数调用时传递的实参。在定义函数的参数列表时,每个参数必须使用
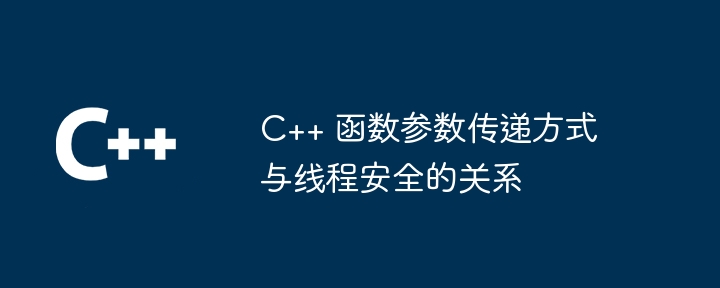
函数参数传递方式与线程安全:值传递:创建参数副本,不影响原始值,通常线程安全。引用传递:传递地址,允许修改原始值,通常不线程安全。指针传递:传递指向地址的指针,类似引用传递,通常不线程安全。在多线程程序中,应慎用引用和指针传递,并采取措施防止数据竞争。
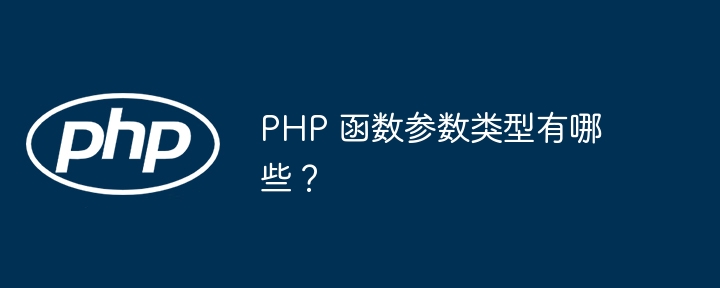
PHP函数参数类型包括标量类型(整数、浮点数、字符串、布尔值、空值)、复合类型(数组、对象)和特殊类型(回调函数、可变参数)。函数可自动转换不同类型参数,但也可通过类型声明强制特定类型,以防止意外转换并确保参数正确性。
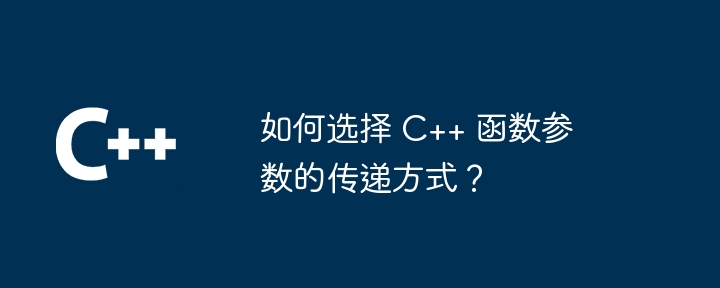
在C++中选择函数参数传递方式时,有四种选择:按值传递、按引用传递、按指针传递和按const引用传递。按值传递创建参数值的副本,不会影响原始参数;按引用传递参数值的引用,可以修改原始参数;按指针传递参数值的指针,允许通过指针修改原始参数值;按const引用传递参数值的const引用,只能访问参数值,不能修改。
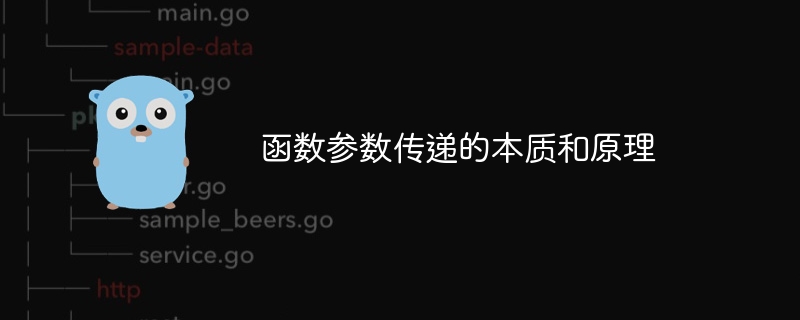
函数参数传递本质上决定了函数获取和修改外部变量的方式。在传值传递下,函数获得传入变量值的副本,对副本的修改不影响外部变量;在传引用传递下,函数直接接收外部变量的引用,对参数的修改也修改外部变量。
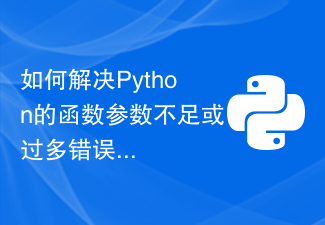
Python是一种高级编程语言,它与其他语言相比具有独特的特点。作为一种面向对象的语言,它能够提供丰富的库和模块,方便用户进行开发和编程。在Python中,函数是编写程序的基本单元,函数的参数可以根据需要进行传递。但是,在编写Python程序时,我们有时会遇到函数参数不足或过多的错误。这些错误可能会导致程序无法运行或结果不正确。为了避免这些错误,我们需要了解
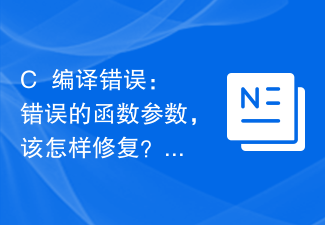
C++是一种流行的编程语言,它被广泛应用于软件开发和系统编程中。C++编译器在解析源代码时,会检查代码的语法和语义,并生成可执行文件或库。当编译器遇到问题时,它会输出一定的错误信息,告诉程序员错误的具体位置和原因。本文将讨论一种常见的C++编译错误--错误的函数参数,并探讨如何修复它。一、错误信息示例下面是一个简单的C++程序,在编译时会出现错误的示例:#i
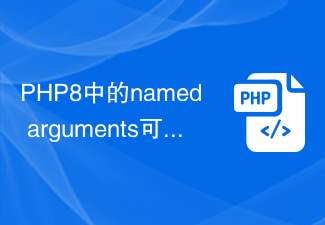
最新发布的PHP8版本带来了一些改进和新特性,其中namedarguments(命名参数)是一个新的功能,它使得函数的参数更加易读。在早期的PHP版本中,使用函数时需要按照定义的参数顺序依次传入每一个参数,这很容易导致混淆和错误。而namedarguments允许开发者为每个参数指定一个名字,然后无需按照顺序传入参数,在使用函数时可以指定参数名并传入相应


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
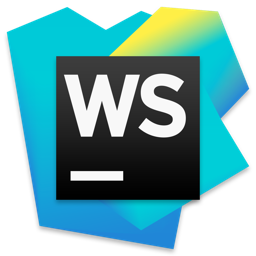
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
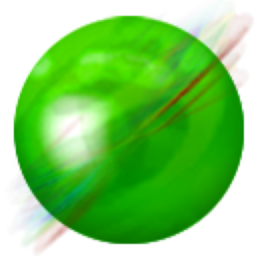
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
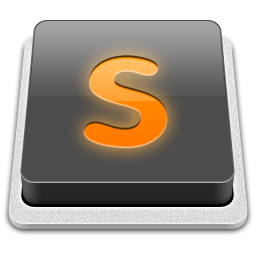
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!
