Code refactoring can be achieved in Go by using custom functions. These functions allow us to define specific functionality and reuse them multiple times. Custom functions improve readability, reduce duplication, and improve maintainability and modularity.
Use custom Go functions to implement code refactoring
Introduction
Code Refactoring is a software engineering practice that improves the structure of code and makes it easier to understand and maintain. In Go, we can use custom functions to simplify repetitive code and improve readability and maintainability.
Custom Functions
Custom functions allow us to define our own specific functionality in code and reuse them as many times as needed. This eliminates the need to copy and paste identical blocks of code, thereby reducing maintenance overhead.
To create a custom function, use the following syntax:
func myFunction(parameters) returnType { // 函数体 }
Practical example
Consider the following example code:
fmt.Println("Hello, world!") fmt.Println("Welcome to Go!") fmt.Println("Let's learn together!")
This code prints three messages repeatedly. We can use custom functions to simplify this process:
package main import ( "fmt" ) // 自定义函数用于打印消息 func PrintMessage(message string) { fmt.Println(message) } func main() { // 调用自定义函数多次 PrintMessage("Hello, world!") PrintMessage("Welcome to Go!") PrintMessage("Let's learn together!") }
By creating the PrintMessage() function, we effectively encapsulate the repetitive task into a reusable unit. This makes the code cleaner and easier to read and understand.
Advantages
Using custom functions for code refactoring has the following advantages:
- Improving readability:By encapsulating repeated code blocks into functions, we can improve the readability of the code.
- Reduce duplication: Custom functions eliminate the need to copy and paste blocks of code, reducing maintenance overhead.
- Improve maintainability: If we need to update or modify specific functionality, we only need to update the custom function.
- Improve Modularity: Custom functions make the code more modular, allowing us to break it down into smaller units easily.
Conclusion
Using custom functions for code refactoring in Go is a powerful technique that can improve the quality, readability and Maintainability. By encapsulating repetitive tasks, we can create concise and easy-to-understand code, thereby maintaining and enhancing readability.
The above is the detailed content of Code refactoring using custom golang function implementation. For more information, please follow other related articles on the PHP Chinese website!
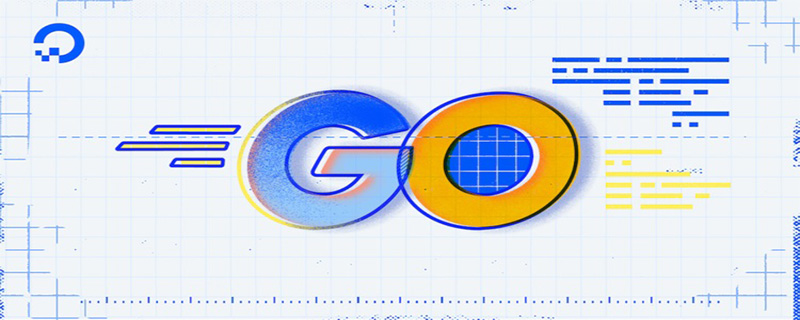
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
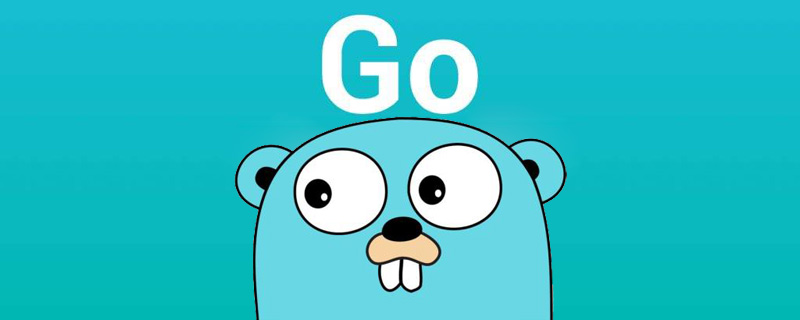
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
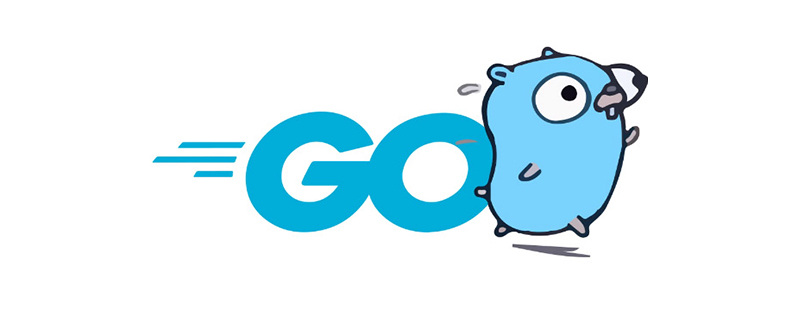
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
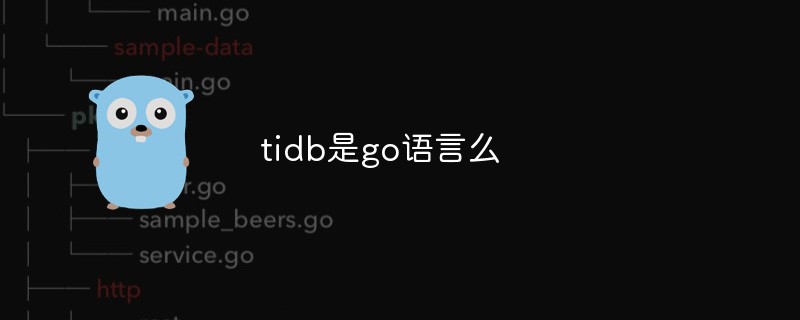
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
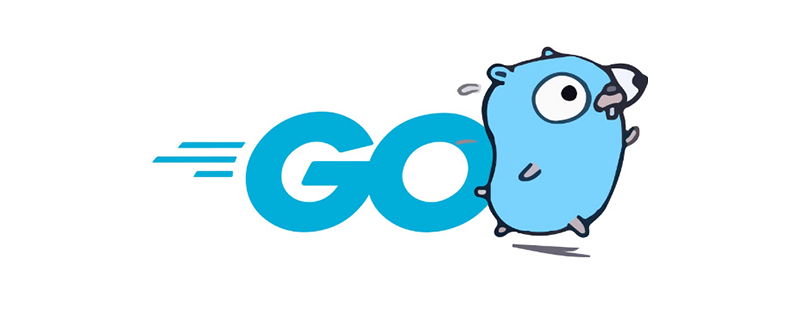
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
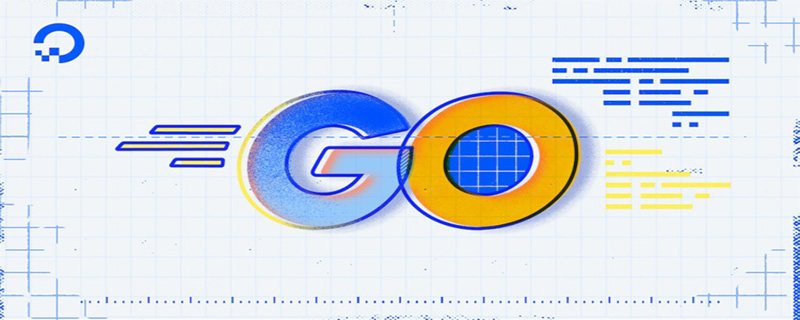
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
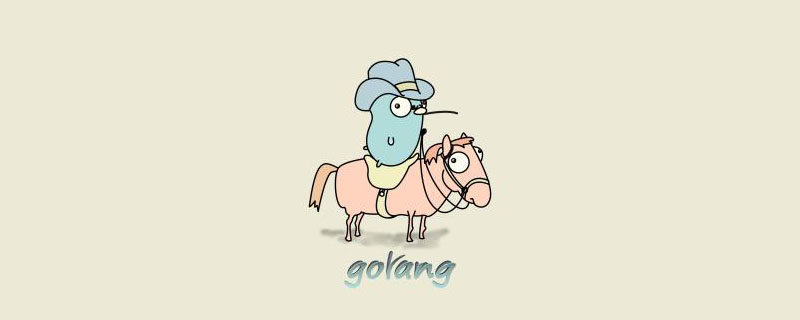
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
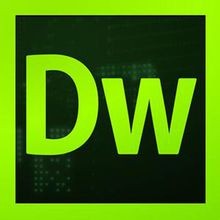
Dreamweaver CS6
Visual web development tools
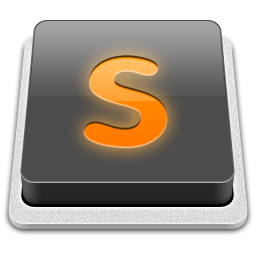
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!
