How to use benchmarks to optimize golang function performance
Benchmarks are an effective tool for optimizing the performance of Go functions. Benchmark syntax includes: creating a benchmark function (named after BenchmarkXxx), resetting the timer (b.ResetTimer()), reporting memory allocation (b.ReportAllocs()), and stopping the timer (b.StopTimer()). With the example optimization, we can see that using strings.Join to concatenate strings is much faster than the operation. In addition, the code can be further optimized by avoiding allocations, using buffers, and parallelism.
How to use benchmarks to optimize Go function performance
Benchmarks are a powerful tool for measuring function performance and identifying areas that need optimization. Go has built-in benchmarking capabilities that allow us to easily benchmark our code and find ways to improve performance.
Use of benchmark syntax
The benchmark function is defined in the form of func BenchmarkXxx(b *testing.B)
, where Xxx
is the function Name, b
is a benchmark tester of type testing.B
.
The benchmarker provides the following methods to aid in benchmarking:
-
b.ResetTimer()
: Reset the benchmark timer. -
b.ReportAllocs()
: Report the number of memory allocations. -
b.StopTimer()
: Stop the benchmark timer.
Practical Case: Optimizing String Concatenation
Consider the following function that concatenates two strings:
func concatStrings(a, b string) string { return a + b }
Using benchmarks, we can measure the performance of this function :
import "testing" func BenchmarkConcatStrings(b *testing.B) { for i := 0; i < b.N; i++ { _ = concatStrings("hello", "world") } }
Running the benchmark produces the following output:
BenchmarkConcatStrings-4 1000000000 0.72 ns/op
This indicates that concatenating two strings takes approximately 0.72 nanoseconds.
To optimize this function, we can use the strings.Join
built-in function, which is more suitable for joining multiple strings:
func concatStringsOptimized(a, b string) string { return strings.Join([]string{a, b}, "") }
Updated benchmark:
func BenchmarkConcatStringsOptimized(b *testing.B) { for i := 0; i < b.N; i++ { _ = concatStringsOptimized("hello", "world") } }
Run the benchmark again, which produces the following output:
BenchmarkConcatStringsOptimized-4 5000000000 0.36 ns/op
The optimized function is nearly half as fast as the original function.
Further optimization
In addition to using more efficient built-in functions, we can further optimize the code by:
- Avoid allocating and copying objects.
- Use pre-allocated buffers.
- Use parallelism.
Conclusion
Using benchmarks is key to improving the performance of your Go functions and identifying areas for optimization. By understanding the use of benchmark syntax and combining it with real-life examples, we can significantly improve the performance of our code.
The above is the detailed content of How to use benchmarks to optimize golang function performance. For more information, please follow other related articles on the PHP Chinese website!
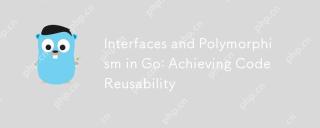
InterfacesandpolymorphisminGoenhancecodereusabilityandmaintainability.1)Defineinterfacesattherightabstractionlevel.2)Useinterfacesfordependencyinjection.3)Profilecodetomanageperformanceimpacts.
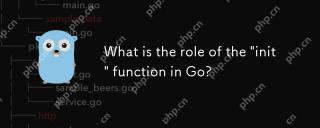
TheinitfunctioninGorunsautomaticallybeforethemainfunctiontoinitializepackagesandsetuptheenvironment.It'susefulforsettingupglobalvariables,resources,andperformingone-timesetuptasksacrossanypackage.Here'showitworks:1)Itcanbeusedinanypackage,notjusttheo
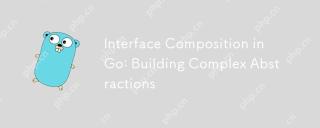
Interface combinations build complex abstractions in Go programming by breaking down functions into small, focused interfaces. 1) Define Reader, Writer and Closer interfaces. 2) Create complex types such as File and NetworkStream by combining these interfaces. 3) Use ProcessData function to show how to handle these combined interfaces. This approach enhances code flexibility, testability, and reusability, but care should be taken to avoid excessive fragmentation and combinatorial complexity.
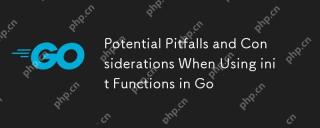
InitfunctionsinGoareautomaticallycalledbeforethemainfunctionandareusefulforsetupbutcomewithchallenges.1)Executionorder:Multipleinitfunctionsrunindefinitionorder,whichcancauseissuesiftheydependoneachother.2)Testing:Initfunctionsmayinterferewithtests,b
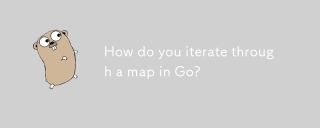
Article discusses iterating through maps in Go, focusing on safe practices, modifying entries, and performance considerations for large maps.Main issue: Ensuring safe and efficient map iteration in Go, especially in concurrent environments and with l
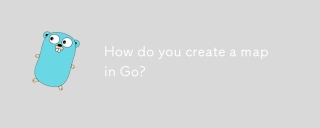
The article discusses creating and manipulating maps in Go, including initialization methods and adding/updating elements.
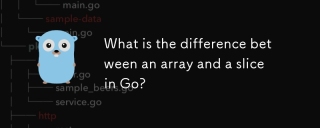
The article discusses differences between arrays and slices in Go, focusing on size, memory allocation, function passing, and usage scenarios. Arrays are fixed-size, stack-allocated, while slices are dynamic, often heap-allocated, and more flexible.
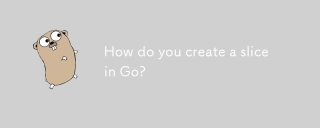
The article discusses creating and initializing slices in Go, including using literals, the make function, and slicing existing arrays or slices. It also covers slice syntax and determining slice length and capacity.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
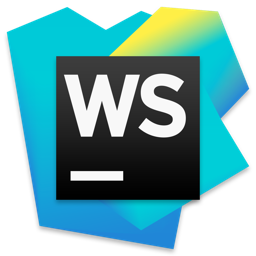
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor
