Enhancing an existing system with GoLang functions can significantly increase its functionality and customization. The specific steps are as follows: Create a GoLang function, write custom function code and build it into a module. Write integration code to load and call custom functions in existing systems. Practical scenario: Integrate custom calculation functions into back-end applications, such as calculating shipping costs. Note: Make sure that the function name matches the one specified and that the integrated system is fully tested for correctness and stability.
Integrating custom GoLang functions into existing systems
Custom GoLang functions can significantly improve the functionality of existing systems and customizability. This tutorial will guide you step-by-step through the integration process and provide a practical example.
Steps:
-
Create GoLang function:
Create a new file in a text editor and add Custom function code, for example:func Square(n int) int { return n * n }
-
Build the GoLang module:
Use the following command to build the GoLand module containing the custom function:go build -buildmode=plugin -o square.so square.go
-
Write integration code:
In an existing system, write code to load and call custom functions. In GoLang, you can use theplugin
package, for example:package main import ( "fmt" "plugin" ) func main() { p, err := plugin.Open("square.so") if err != nil { fmt.Println(err) return } squareFn, err := p.Lookup("Square") if err != nil { fmt.Println(err) return } v, err := squareFn.(func(int) int)(5) if err != nil { fmt.Println(err) return } fmt.Println(v) // 输出:25 }
Practical case:
A common scenario is to Custom calculation functions are integrated into backend applications. For example, you might need to calculate shipping costs for your online store.
Implementation:
- Create a GoLang function to calculate shipping costs and build it as a module.
- In the backend application, load and call custom functions to calculate shipping costs.
- Integrate shipping amount into order processing logic.
Note:
- Make sure the custom function name matches the name specified in
plugin.Lookup
. - Fully test the integrated system to ensure correctness and stability.
- Consider security management, such as verifying signatures or permissions before loading modules.
The above is the detailed content of Integrate custom golang function implementation into existing systems. For more information, please follow other related articles on the PHP Chinese website!
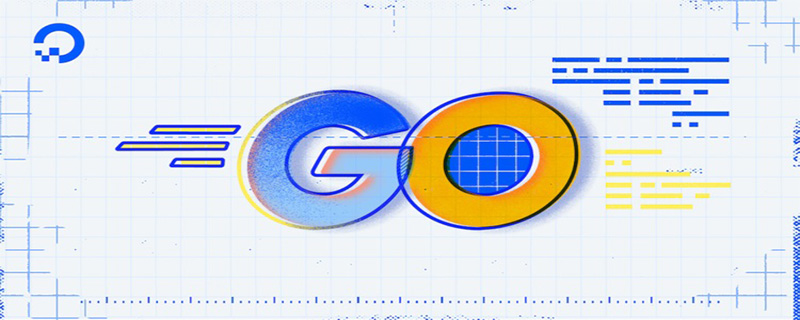
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
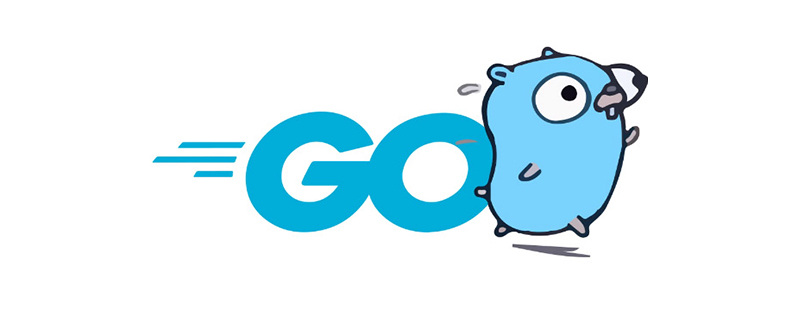
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
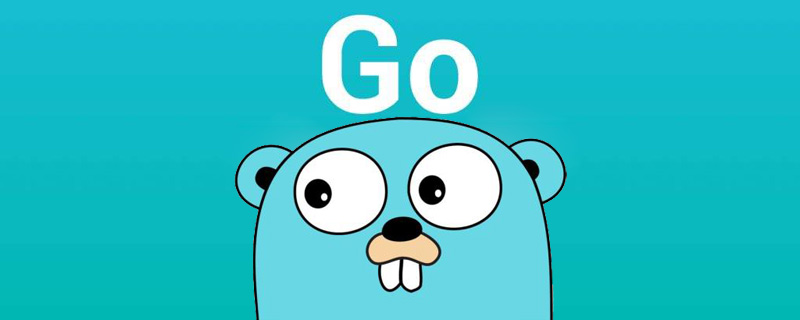
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
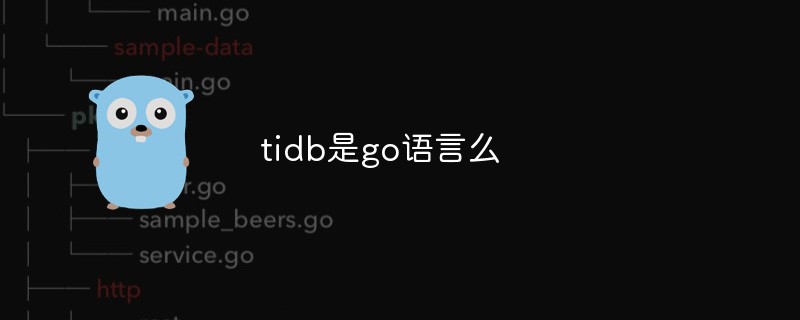
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
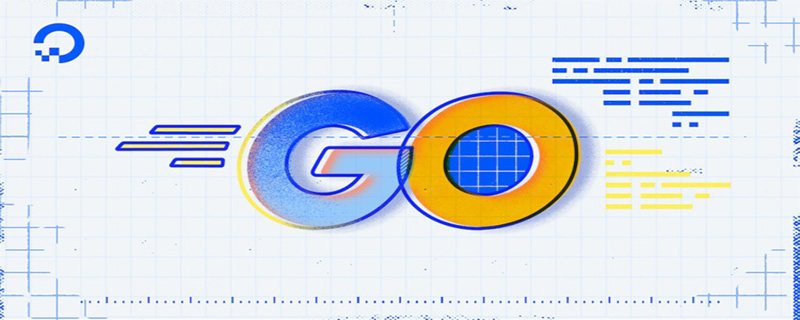
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
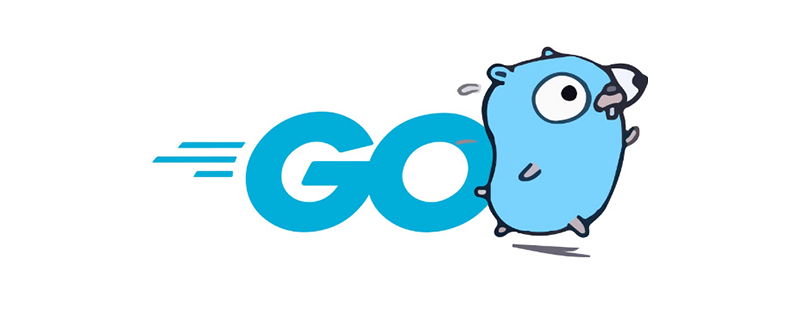
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
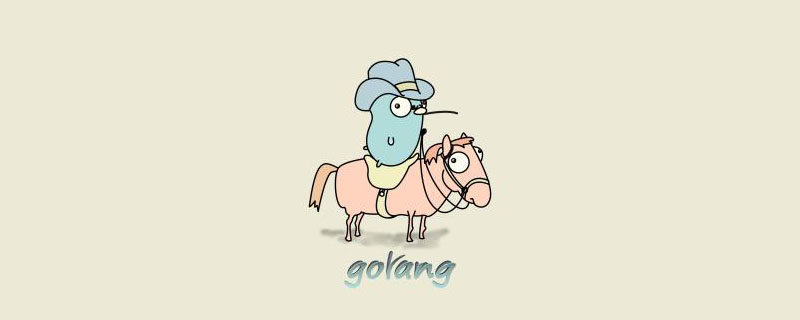
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
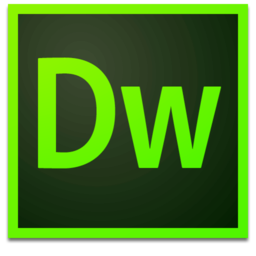
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
