How to create robust Java functions to handle various error scenarios?
在 Java 中创建健壮函数处理错误场景的方法包括:使用 try-catch 块隔离错误代码并执行特定异常处理。抛出异常让调用方决定如何处理错误。创建自定义异常类提供更多错误详细信息。
如何创建健壮的 Java 函数来处理各种错误场景
在现实世界的应用程序中,处理错误场景至关重要,以确保应用程序的稳定性和可靠性。在 Java 中,有几种方法可以创建健壮的函数来应对各种错误场景。
1. 使用 try-catch 块
try-catch 块是一种常见的错误处理机制,它允许您指定需要尝试的代码块,以及在出现异常时要执行的代码块。例如:
try { // 尝试执行的代码 } catch (Exception e) { // 出现异常时执行的代码 }
2. 抛出异常
在某些情况下,您可能希望将错误作为异常抛出,从而让调用方决定如何处理错误。例如:
public void someMethod() throws Exception { // ... // 如果出现错误,抛出异常 // ... }
3. 使用自定义异常类
您可以创建自己的自定义异常类,从而提供更多错误详细信息。例如:
public class MyException extends Exception { private String message; public MyException(String message) { this.message = message; } public String getMessage() { return message; } }
实战案例
假设我们有一个函数需要根据给定的文件路径读取文件内容。如果文件不存在或无法读取,我们希望抛出异常。
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ReadFile { public static String readFile(String filePath) throws FileNotFoundException { File file = new File(filePath); // 检查文件是否存在 if (!file.exists()) { throw new FileNotFoundException("File not found: " + filePath); } // 尝试读取文件内容 try (Scanner scanner = new Scanner(file)) { return scanner.useDelimiter("\\Z").next(); } } public static void main(String[] args) { try { String contents = readFile("path/to/file.txt"); // 使用文件内容 } catch (FileNotFoundException e) { // 处理文件不存在或无法读取的情况 } } }
优势
创建健壮的 Java 函数来处理各种错误场景有很多优势,包括:
- 应用程序稳定性:它可以防止应用程序在遇到错误时崩溃或产生不可预期的行为。
- 可维护性:它使应用程序易于维护,因为错误处理逻辑与业务逻辑分离。
- 可扩展性:它允许您轻松适应未来的错误场景。
The above is the detailed content of How to create robust Java functions to handle various error scenarios?. For more information, please follow other related articles on the PHP Chinese website!
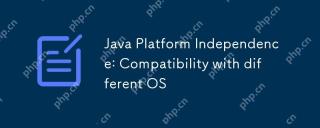
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
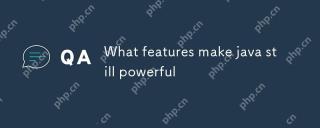
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
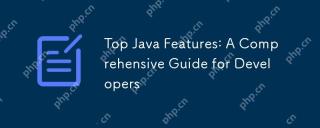
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
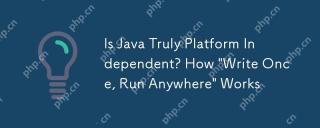
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
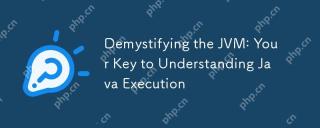
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
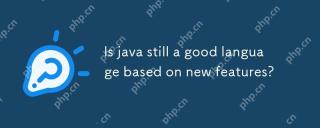
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
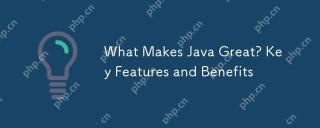
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
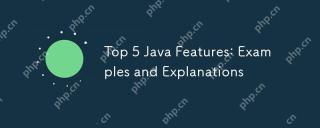
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
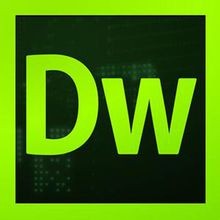
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
