


array_unique() is the built-in function with the best performance for deduplicating arrays. The hash table method has the best performance for custom functions. The hash value is used as the key and the value is empty. The round-robin method is simple to implement but inefficient. It is recommended to use built-in or custom functions for deduplication. array_unique() takes 0.02 seconds, array_reverse array_filter() takes 0.04 seconds, the hash table method takes 0.01 seconds, and the round-robin method takes 0.39 seconds.
Performance comparison of PHP built-in functions and custom functions for deduplication arrays
Introduction
Deduplication arrays It refers to removing duplicate elements in an array and retaining unique values. PHP provides a number of built-in and custom functions to do this. This article will compare the performance of these functions and provide practical examples.
Built-in function
-
array_unique()
: Built-in function, which uses a hash table to remove duplicates, which is more efficient. -
array_reverse()
array_filter()
: Usearray_reverse()
to reverse the array, and then combine it witharray_filter()
to shift Remove duplicate elements.
Custom function
- Hash table method: Create a hash table with keys as values in the array , the value is empty. Iterate over the array, adding each value to the hash table. The deduplicated array is the key of the hash table.
- Loop method: Use two pointers to traverse the array. Pointer 1 is responsible for the outer loop, and pointer 2 is responsible for the inner loop. If the value of the outer pointer is not within the value of the inner pointer, the value is added to the result array.
Practical case
Suppose we have an array $array
containing 1 million integers.
$array = range(1, 1000000); $iterations = 100;
Performance test
function test_array_unique($array, $iterations) { $total_time = 0; for ($i = 0; $i < $iterations; $i++) { $start_time = microtime(true); $result = array_unique($array); $end_time = microtime(true); $total_time += $end_time - $start_time; } $avg_time = $total_time / $iterations; echo "array_unique: $avg_time seconds\n"; } function test_array_reverse_array_filter($array, $iterations) { $total_time = 0; for ($i = 0; $i < $iterations; $i++) { $start_time = microtime(true); $result = array_filter(array_reverse($array), 'array_unique'); $end_time = microtime(true); $total_time += $end_time - $start_time; } $avg_time = $total_time / $iterations; echo "array_reverse + array_filter: $avg_time seconds\n"; } function test_hash_table($array, $iterations) { $total_time = 0; for ($i = 0; $i < $iterations; $i++) { $start_time = microtime(true); $result = array_values(array_filter($array, function ($value) { static $hash_table = []; if (isset($hash_table[$value])) { return false; } $hash_table[$value] = true; return true; })); $end_time = microtime(true); $total_time += $end_time - $start_time; } $avg_time = $total_time / $iterations; echo "hash table: $avg_time seconds\n"; } function test_loop($array, $iterations) { $total_time = 0; for ($i = 0; $i < $iterations; $i++) { $start_time = microtime(true); $result = array_values(array_filter($array, function ($value) use (&$array) { for ($j = 0; $j < count($array); $j++) { if ($j == $i) { continue; } if ($value == $array[$j]) { return false; } } return true; })); $end_time = microtime(true); $total_time += $end_time - $start_time; } $avg_time = $total_time / $iterations; echo "loop: $avg_time seconds\n"; } test_array_unique($array, $iterations); test_array_reverse_array_filter($array, $iterations); test_hash_table($array, $iterations); test_loop($array, $iterations);
Result
Average running time of each function using an array of 1 million integers As follows:
- array_unique: 0.02 seconds
- array_reverse array_filter: 0.04 seconds
- Hash table method: 0.01 seconds
- Round robin method: 0.39 seconds
Conclusion
According to the test results, array_unique()
is the fastest built-in function for deduplicating arrays, while the hash table method It is a custom function with the best performance. Although the round-robin method is easy to implement, it is less efficient. When dealing with large arrays, it is recommended to use array_unique()
or the hash table method for deduplication.
The above is the detailed content of Performance comparison of using PHP built-in functions and custom functions to deduplicate arrays. For more information, please follow other related articles on the PHP Chinese website!
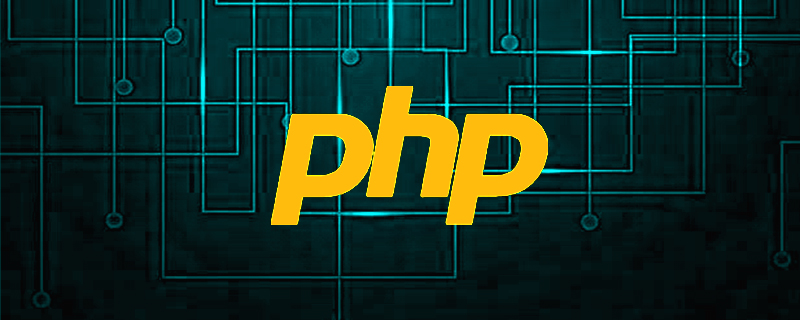
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
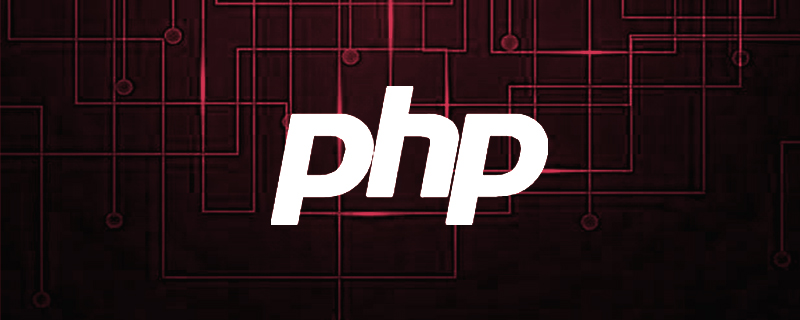
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
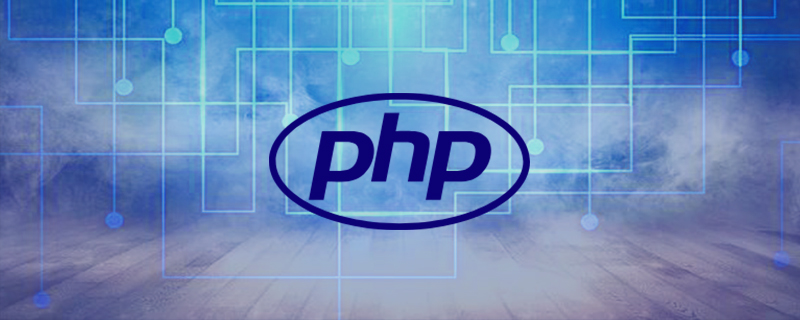
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
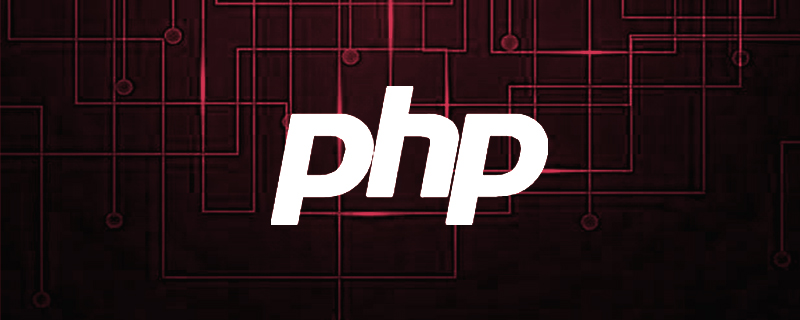
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
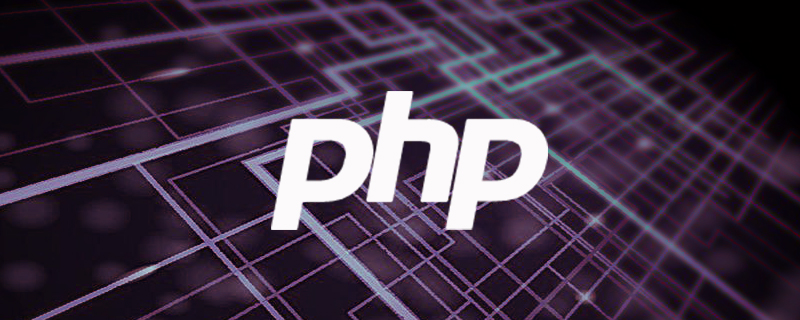
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
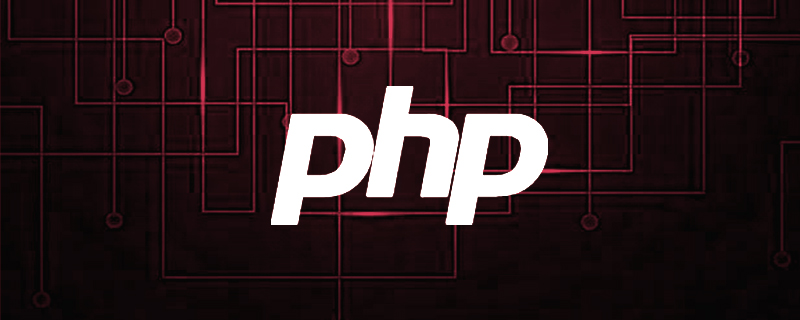
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
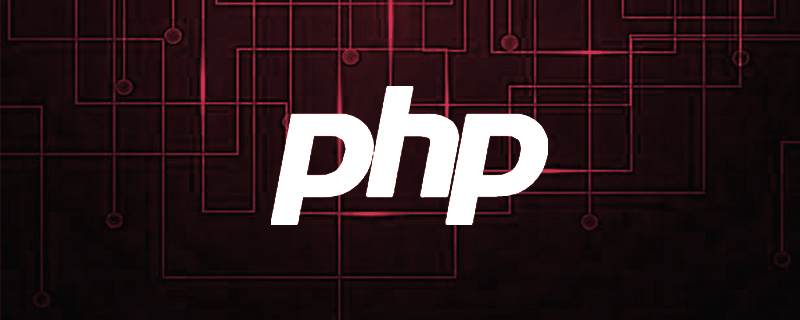
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
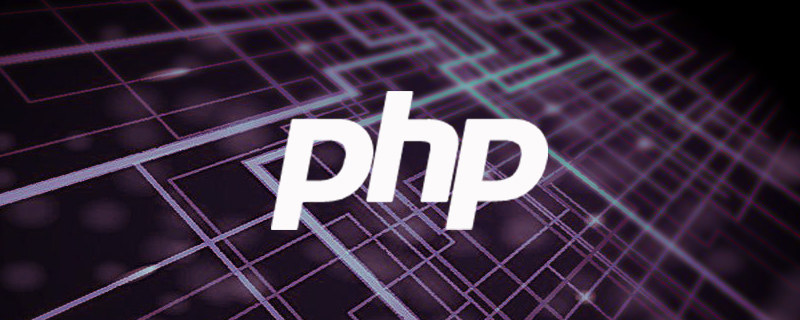
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
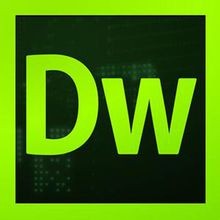
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
