C supports distributed concurrent programming and provides the following functions: Parallel computing library: std::thread, std::mutex and std::condition_variable, used to create and manage threads, synchronize access to shared resources and wait for conditions. Function templates: allow generic programming and reusable code to handle different types of objects or data structures, facilitating data synchronization and distributed computing in distributed systems.
#How do C functions support distributed concurrent programming?
In distributed systems, concurrent programming is critical to achieving high performance and scalability. The C language provides powerful features that make it ideal for distributed concurrent programming.
C Functions in parallel computing
C provides parallel computing libraries, such as std::thread
, std::mutex
and std::condition_variable
, for concurrent execution of tasks on multi-core systems. These functions allow us to create and manage threads, synchronize access to shared resources, and wait conditions.
Function Template
C Function template allows generic programming to reuse code to handle different types of objects or data structures. This is useful for synchronizing data in distributed systems and distributing computations to multiple nodes.
Practice case: Using C to implement a distributed task queue
The following code shows how to use C functions to implement a distributed task queue, in which different threads process different Task:
#include <iostream> #include <thread> #include <queue> #include <mutex> #include <condition_variable> std::queue<int> task_queue; std::mutex task_queue_mutex; std::condition_variable task_queue_cv; void worker_thread() { while (true) { std::unique_lock<std::mutex> lock(task_queue_mutex); while (task_queue.empty()) { task_queue_cv.wait(lock); } int task = task_queue.front(); task_queue.pop(); // 执行任务 std::cout << "Worker thread processing task: " << task << std::endl; } } int main() { // 创建工作线程 std::vector<std::thread> worker_threads; for (int i = 0; i < 10; i++) { worker_threads.push_back(std::thread(worker_thread)); } // 向队列中添加任务 for (int i = 0; i < 100; i++) { std::unique_lock<std::mutex> lock(task_queue_mutex); task_queue.push(i); task_queue_cv.notify_one(); } // 等待任务完成 for (auto& worker : worker_threads) { worker.join(); } return 0; }
Conclusion
C functions provide a wide range of capabilities to support distributed parallel programming. With its powerful and scalable features, C can efficiently create and synchronize concurrent tasks to implement distributed system requirements.
The above is the detailed content of How do C++ functions support distributed concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!
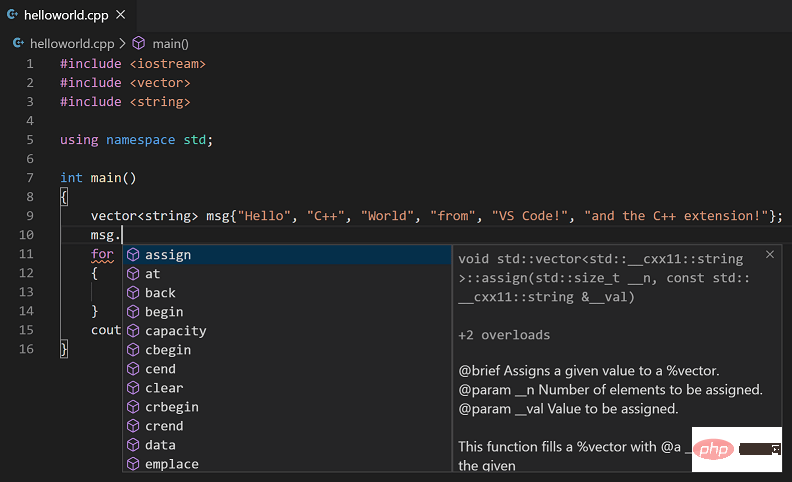
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
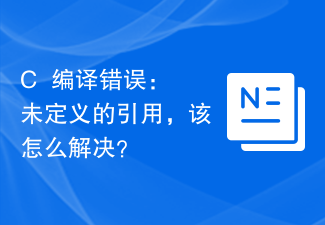
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
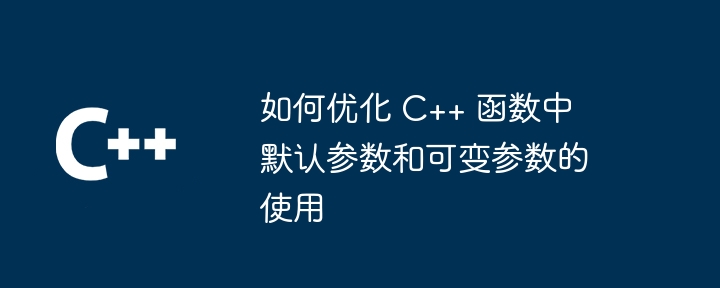
优化C++默认和可变参数函数:默认参数:允许函数使用默认值,减少冗余。将默认参数放在最后以提高可读性。使用constexpr默认参数以减少开销。使用结构化绑定以提高复杂默认参数的可读性。可变参数:允许函数接受数量不定的参数。尽量避免使用可变参数,并在必要时使用。使用std::initializer_list优化可变参数函数以提高性能。
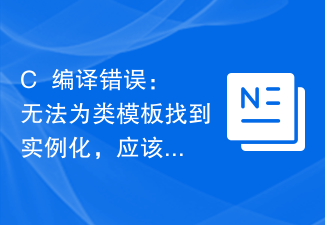
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
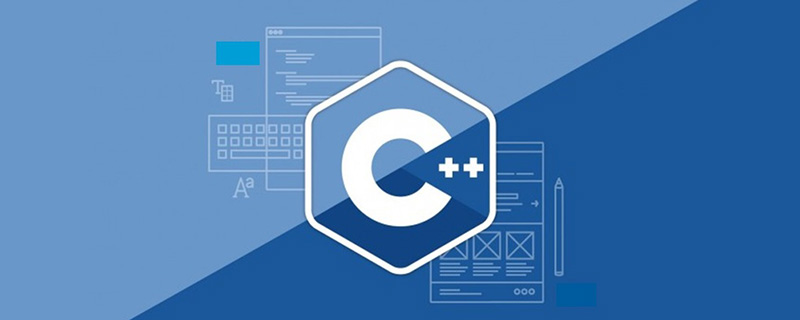
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
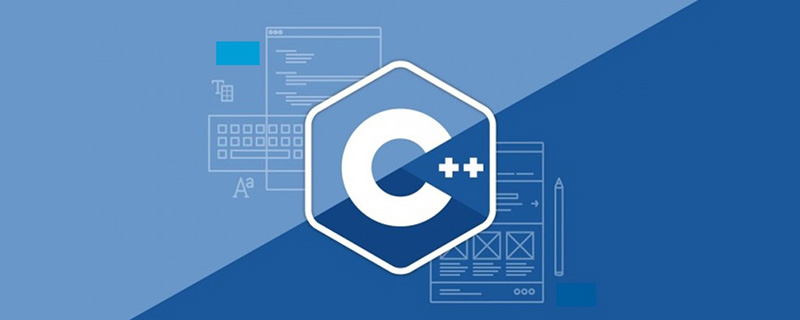
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
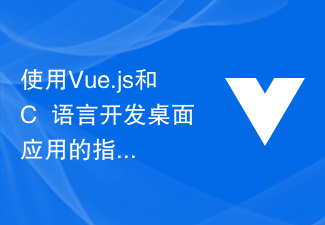
使用Vue.js和C++语言开发桌面应用的指南随着互联网的发展,前端技术也在不断更新和进步。而Vue.js作为一种轻量级、高效、易用的前端框架,在开发Web应用方面具有很大的优势。然而,在一些特定的场景中,我们可能需要开发一些更加复杂的桌面应用程序,这时候就需要结合C++语言来实现一些底层功能。本文将会介绍如何使用Vue.js和C++语言开发桌面应用,并提供
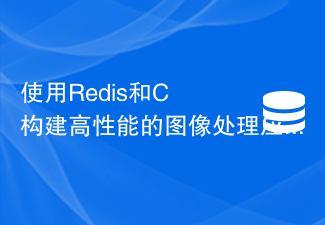
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
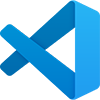
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
