There are two ways to retain two decimal places in output in C: 1. Use std::fixed and std::setprecision to control the output stream formatting, such as: cout
How to retain two decimal places in C output?
There are two ways to retain two decimal places in output in C:
1. Use std::fixed and std::setprecision
This method is implemented by controlling the formatting of the output stream.
#include <iostream> #include <iomanip> using namespace std; int main() { double value = 123.456789; // 设置保留两位小数 cout << fixed << setprecision(2) << value << endl; return 0; }
Output:
<code>123.46</code>
2. Using std::to_string and std::substr
This method involves converting a number to a string, Then extract the required number of decimal places.
#include <iostream> #include <string> using namespace std; int main() { double value = 123.456789; // 转换为字符串 string str = to_string(value); // 提取两位小数 string result = str.substr(0, str.find('.') + 3); cout << result << endl; return 0; }
Output:
<code>123.46</code>
The above is the detailed content of How to retain 2 decimal places for output in c++. For more information, please follow other related articles on the PHP Chinese website!
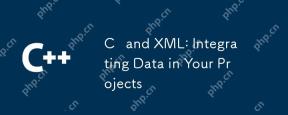
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
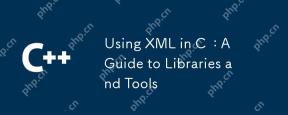
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
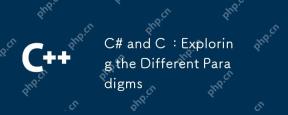
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
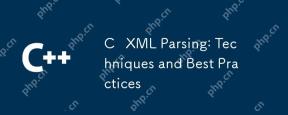
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
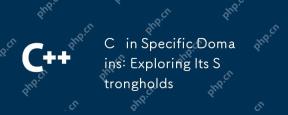
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
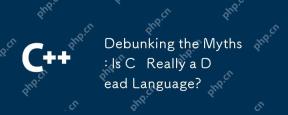
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
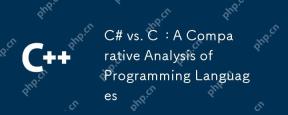
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
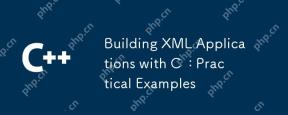
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
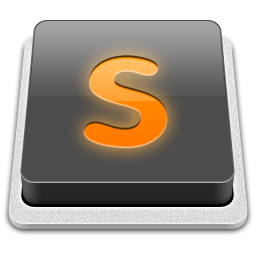
SublimeText3 Mac version
God-level code editing software (SublimeText3)
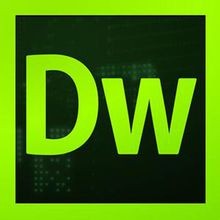
Dreamweaver CS6
Visual web development tools
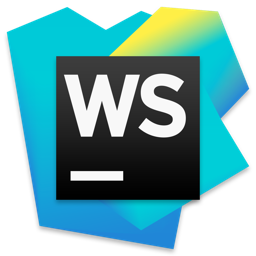
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
