


How do C++ functions handle timeouts and exceptions in network programming?
C In network programming, use the chrono library to set the timeout when processing timeouts, such as setting a 10-second timeout: std::chrono::seconds timeout = 10s;. Use try-catch statements to handle exceptions, such as: try { ... } catch (const std::exception& e) { ... }.
How C functions handle timeouts and exceptions in network programming
In network programming, timeouts and exceptions are common challenges . C provides powerful functions for handling these situations, and this article will explore how to use them effectively.
Handling timeouts
C provides the chrono
library to manage time. To set a timeout, you can use the following function:
#include <chrono> using namespace std::chrono_literals; std::chrono::seconds timeout = 10s; // 设置 10 秒的超时
Practical case: Use the select()
function to implement the timeout
select( )
Function waits for the readability of one or more file descriptors for a specific period of time. It can be used with timeouts:
#include <sys/select.h> int main() { // 设置文件描述符集合 fd_set fds; FD_ZERO(&fds); FD_SET(socket_fd, &fds); // 设置超时 struct timeval timeout; timeout.tv_sec = 10; timeout.tv_usec = 0; // 等待可读性或超时 int result = select(socket_fd + 1, &fds, NULL, NULL, &timeout); if (result == 0) { // 超时 std::cout << "Operation timed out." << std::endl; } else if (result > 0) { // 文件描述符可读 // ... } else { // 错误 std::cout << "An error occurred." << std::endl; } return 0; }
Handling Exceptions
C Use exceptions to handle exceptional situations. When an exception is thrown, it causes the immediate termination of the current function and transfers control to its caller. To catch exceptions, you can use try-catch
statements around the code block:
#include <stdexcept> try { // ... } catch (const std::exception& e) { // 异常处理 std::cout << "An exception occurred: " << e.what() << std::endl; }
Practical example: Handling std::runtime_error
exceptions in a network connection
std::runtime_error
is a commonly used exception used to represent runtime errors. It can throw when a network connection fails:
#include <iostream> using namespace std; int main() { try { // 建立网络连接 // ... } catch (const std::runtime_error& e) { // 连接失败 cout << "Connection failed: " << e.what() << endl; } return 0; }
Efficient handling of timeouts and exceptions is critical for robust and reliable network applications. C provides powerful functions that allow you to easily manage these situations and ensure that your code still works properly when unforeseen problems arise.
The above is the detailed content of How do C++ functions handle timeouts and exceptions in network programming?. For more information, please follow other related articles on the PHP Chinese website!
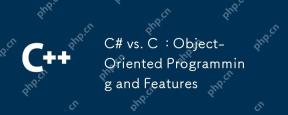
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
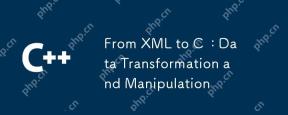
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
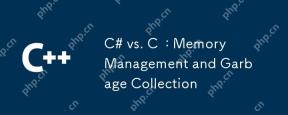
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
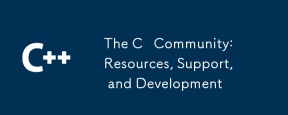
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
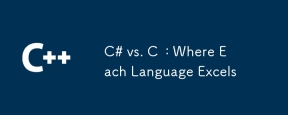
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
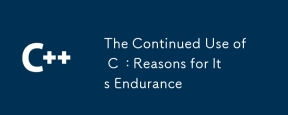
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
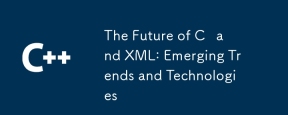
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool