In multi-threading, Java exception handling is crucial and can be implemented in the following ways: Thread exception handling: Each thread has an independent exception handler. When an uncaught exception occurs, the thread is terminated and the exception is propagated to the calling thread. Exception propagation: The function declares the exceptions that may be thrown through throws, forcing the caller to handle them. Uncaught exceptions are propagated upwards. Thread pool exception handling: The thread pool prints exceptions and closes the thread by default. ExecutorService: Provides more fine-grained control, allowing you to specify the processing logic when thread exceptions are not caught.
Concurrency and multi-threaded exception handling of Java functions
In a multi-threaded environment, exception handling is a key aspect. It is related to the stability and reliability of the program. Java provides several mechanisms to handle exceptions in multiple threads.
Thread exception handling
Each thread has its own exception handler. When an uncaught exception occurs, the thread terminates and the exception is propagated to the calling thread as a Throwable
object.
Exception propagation
Declaring that a function may throw an exception using the throws
keyword will force the caller of the function to handle the exception. If uncaught, the exception will propagate up the call stack.
Thread pool exception handling
The thread pool provides a default mechanism for handling thread exceptions. When an uncaught exception occurs, the thread pool prints the exception and closes the thread.
ExecutorService
java.util.concurrent.ExecutorService
interface provides more fine-grained exception handling control. It allows you to specify a Thread.UncaughtExceptionHandler
that is called when an uncaught exception occurs.
Practical case
Consider the following multi-threaded function:
public static void processList(List<String> list) { for (String item : list) { try { // 处理项,可能抛出异常 } catch (Exception e) { // 处理异常 } } }
This is a simple example where each thread processes one item in the list , and handle exceptions through try-catch blocks.
ExecutorService Using
Using ExecutorService
provides more control over exception handling. Here's how to use Thread.UncaughtExceptionHandler
:
ExecutorService executor = Executors.newFixedThreadPool(5); executor.setUncaughtExceptionHandler(new Thread.UncaughtExceptionHandler() { @Override public void uncaughtException(Thread t, Throwable e) { // 自定义异常处理逻辑 } });
In this example, Thread.UncaughtExceptionHandler
can execute custom logic when an uncaught exception occurs.
By following these best practices, you can ensure that exceptions are handled effectively in a multi-threaded environment, improving the robustness and reliability of your code.
The above is the detailed content of How does Java function concurrency and multi-threading handle exceptions?. For more information, please follow other related articles on the PHP Chinese website!
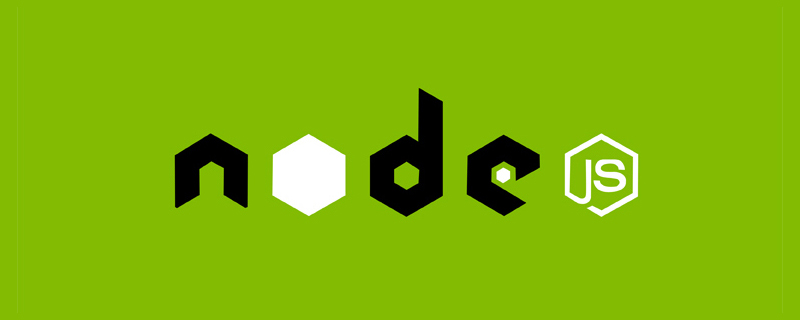
大家都知道 Node.js 是单线程的,却不知它也提供了多进(线)程模块来加速处理一些特殊任务,本文便带领大家了解下 Node.js 的多进(线)程,希望对大家有所帮助!
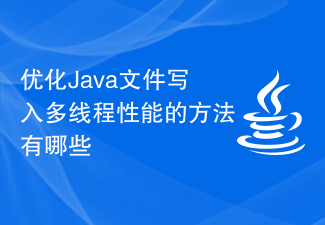
Java开发中如何优化文件写入多线程并发性能在大规模数据处理的场景中,文件的读写操作是不可避免的,而且在多线程并发的情况下,如何优化文件的写入性能变得尤为重要。本文将介绍一些在Java开发中优化文件写入多线程并发性能的方法。合理使用缓冲区在文件写入过程中,使用缓冲区可以大大提高写入性能。Java提供了多种缓冲区实现,如ByteBuffer、CharBuffe
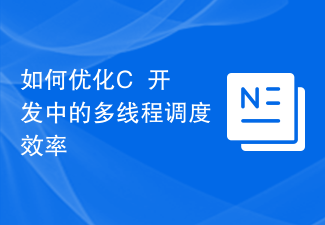
在当今的软件开发领域中,多线程编程已经成为了一种常见的开发模式。而在C++开发中,多线程调度的效率优化是开发者需要关注和解决的一个重要问题。本文将围绕如何优化C++开发中的多线程调度效率展开讨论。多线程编程的目的是为了充分利用计算机的多核处理能力,提高程序运行效率和响应速度。然而,在并行执行的同时,多线程之间的竞争条件和互斥操作可能导致线程调度的效率下降。为
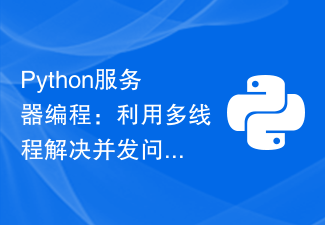
随着互联网的发展,越来越多的应用程序被开发出来,它们需要处理并发请求。例如,Web服务器需要处理多个客户端请求。在处理并发请求时,服务器需要同时处理多个请求。这时候,Python中的多线程技术就可以派上用场了。本文将介绍如何使用Python多线程技术解决并发问题。首先,我们将了解什么是多线程。然后,我们将讨论使用多线程的优点和缺点。最后,我们将演示一个实例,
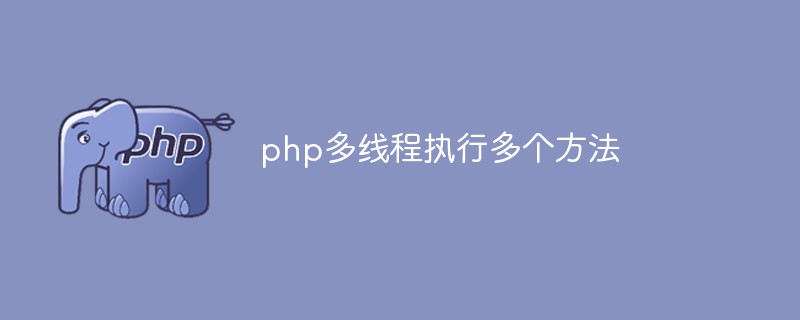
在PHP开发中,经常会遇到需要同时执行多个操作的情况。想要在一个进程中同时执行多个耗时操作,就需要使用PHP的多线程技术来实现。本文将介绍如何使用PHP多线程执行多个方法,提高程序的并发性能。
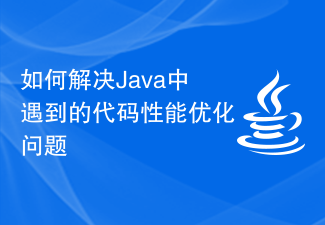
如何解决Java中遇到的代码性能优化问题随着现代软件应用的复杂性和数据量的增加,对于代码性能的需求也变得越来越高。在Java开发中,我们经常会遇到一些性能瓶颈,如何解决这些问题成为了开发者们关注的焦点。本文将介绍一些常见的Java代码性能优化问题,并提供一些解决方案。一、避免过多的对象创建和销毁在Java中,对象的创建和销毁是需要耗费资源的。因此,当一个方法
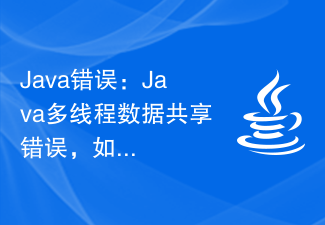
随着社会的发展和科技的进步,计算机程序已经渐渐成为我们生活中不可或缺的一部分。而Java作为一种流行的编程语言,以其可移植性、高效性和面向对象特性等而备受推崇。然而,Java程序开发过程中可能会出现一些错误,如Java多线程数据共享错误,这对于程序员们来说并不陌生。在Java程序中,多线程是非常常见的,开发者通常会使用多线程来优化程序的性能。多线程能够同时处
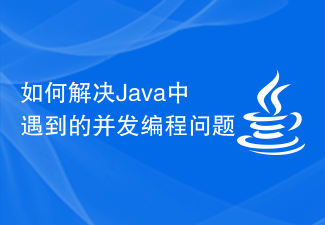
如何解决Java中遇到的并发编程问题随着计算机技术的发展和应用场景的扩大,多线程编程在软件开发中变得越来越重要。而Java作为一种常用的编程语言,也提供了强大的支持来进行并发编程。然而,并发编程也带来了一些挑战,如数据竞争、死锁、活锁等问题。本文将探讨在Java中如何解决这些并发编程的问题。数据竞争数据竞争是指当多个线程同时访问和修改共享数据时,由于执行顺序


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
