How do C++ function overloading and virtual functions work together?
Function overloading in C allows defining different implementations for functions of the same name with different parameters, while virtual functions allow overriding base class functions in derived classes to achieve polymorphism. Function overloading and virtual functions can work together. By designing a virtual overloaded function in the base class, the derived class can only overload versions of specific parameter combinations, thereby providing more flexible polymorphism, such as calculating different types in practical cases The distance of the shape from its origin.
Cooperation of function overloading and virtual functions in C
Introduction
C language provides two mechanisms to achieve polymorphism: function overloading and virtual functions. Function overloading allows defining multiple functions with the same name but different behavior based on parameter types. Virtual functions allow functions in a base class to be overridden in a derived class, thus supporting polymorphism in inheritance.
Function Overloading
Function overloading allows defining different implementations for multiple functions with the same name but different parameter lists. The compiler will choose the correct function based on the argument types when called. For example:
int add(int a, int b) { return a + b; } double add(double a, double b) { return a + b; }
When used:
int sum1 = add(1, 2); // 呼叫整數版本 double sum2 = add(1.5, 2.3); // 呼叫浮點版本
Virtual function
Virtual function allows a derived class to override a function in a base class. When a virtual function is called through a base class pointer, the overridden version in the derived class is executed. For example:
class Shape { public: virtual double getArea() const = 0; }; class Circle : public Shape { public: double getArea() const override { return 3.14 * radius * radius; } private: double radius; };
When used:
Shape* shape = new Circle(5.0); double area = shape->getArea(); // 會呼叫 Circle::getArea()
Collaboration of function overloading and virtual functions
Function overloading and virtual functions can work together to Provides more flexible polymorphism. By designing a virtual overloaded function in the base class, a derived class can overload only versions with specific parameter combinations. For example:
class Shape { public: virtual double getArea(bool isFilled) const { return 0.0; } }; class Circle : public Shape { public: double getArea(bool isFilled) const override { if (isFilled) { return 3.14 * radius * radius; } else { return 0.0; } } };
When used:
Shape* shape = new Circle(5.0); double filledArea = shape->getArea(true); // 呼叫 Circle::getArea(bool) double unfilledArea = shape->getArea(false); // 呼叫 Shape::getArea(bool)
Practical case
The following is an example of using function overloading and virtual function collaboration in computational geometry Practical case:
class Shape { public: virtual double distanceToOrigin() const = 0; }; class Point : public Shape { public: double distanceToOrigin() const override { return 0.0; } }; class Circle : public Shape { public: double distanceToOrigin() const override { return radius; } }; class Rectangle : public Shape { public: double distanceToOrigin() const override { return min(x, y); } }; int main() { Shape* shapes[] = {new Point(), new Circle(5.0), new Rectangle(3.0, 4.0)}; for (Shape* shape : shapes) { cout << "距離原點: " << shape->distanceToOrigin() << endl; } }
This code demonstrates the use of function overloading and virtual functions to calculate the distance from the origin of different types of shapes.
The above is the detailed content of How do C++ function overloading and virtual functions work together?. For more information, please follow other related articles on the PHP Chinese website!
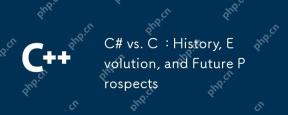
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
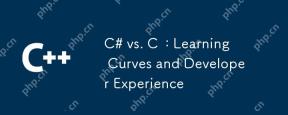
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
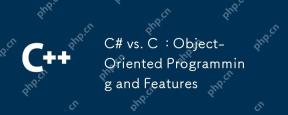
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
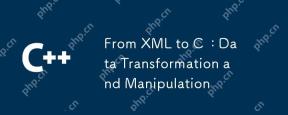
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
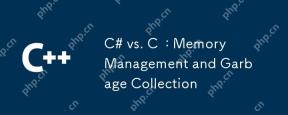
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
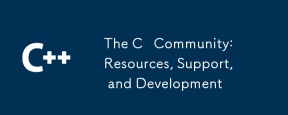
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
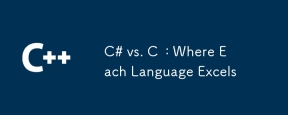
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
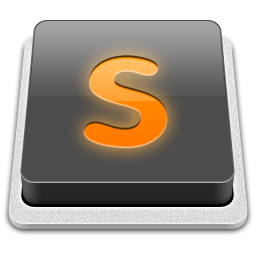
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.