What are the advantages of closures in object-oriented programming?
Closures provide multiple advantages in object-oriented programming, including: Encapsulation: Encapsulate private variables and methods by accessing and modifying variables in the scope of external functions, improving code security. Data hiding: Hide the internal state of objects to improve code maintainability. Memory management: Helps manage the memory of objects and releases the resources of objects that are no longer needed. Asynchronous programming: Conveniently implement asynchronous programming and handle the response of asynchronous functions.
Advantages of closures in object-oriented programming
Introduction
Oriented Object programming (OOP) is a software development approach that uses classes and objects to organize code. A closure is a function that can access and modify variables in the scope of an outer function. In OOP, closures provide many advantages, including:
Encapsulation
Closures can be used to encapsulate private variables and methods, which helps improve the safety. For example, we can create a function to access an object's private variables without exposing them:
class Person { #name; getName() { return this.#name; } } const person = new Person(); const getName = person.getName; // 闭包 console.log(getName()); // 输出: undefined (无法直接访问私有变量)
Data Hiding
Closures can be used to hide the internals of an object status, which helps improve the maintainability of your code. For example, we can create a closure that calculates the average of an object without exposing the calculation logic to the outside:
class Calculator { #values = []; add(value) { this.#values.push(value); } getAverage() { const average = this.#values.reduce((a, b) => a + b) / this.#values.length; return average; } } const calculator = new Calculator(); calculator.add(1); calculator.add(2); const getAverage = calculator.getAverage; // 闭包 console.log(getAverage()); // 输出: 1.5 ```` **内存管理** 闭包可以帮助管理对象的内存。例如,我们可以创建一个闭包来释放一个对象的资源,当对象不再需要时:
class MyClass {
#resource;
constructor() {
this.#resource = new Resource();
}
close() {
this.#resource.close();
}
}
const myClass = new MyClass();
const close = myClass .close; // Closure
myClass = null; // Release the memory of MyClass
close(); // Release the memory of Resource
**异步编程** 闭包可以方便地实现异步编程。例如,我们可以创建一个闭包来处理异步函数(例如 `fetch`)的响应:
async function fetchUserData() {
const response = await fetch('/user');
const data = await response.json();
return data;
}
const getUserData = fetchUserData (); // Closure
getUserData.then((data) => {
console.log(data);
});
**结论**
The above is the detailed content of What are the advantages of closures in object-oriented programming?. For more information, please follow other related articles on the PHP Chinese website!
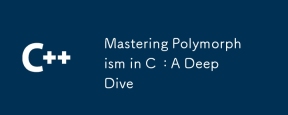
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
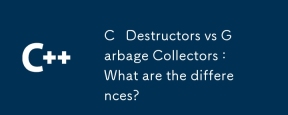
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
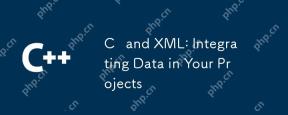
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
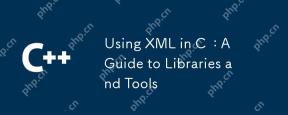
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
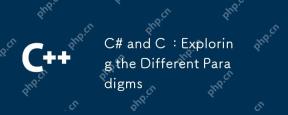
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
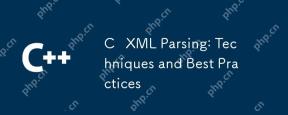
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
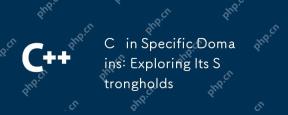
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
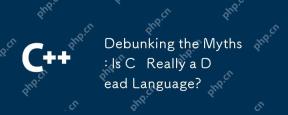
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
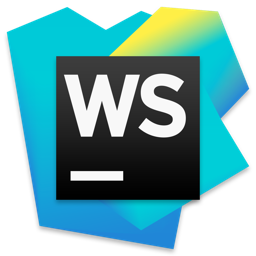
WebStorm Mac version
Useful JavaScript development tools
