Golang Functional Programming In distributed systems, functional programming is widely used in the development of scalable and maintainable high-performance systems. Golang supports features such as anonymous functions, closures, and higher-order functions, making functional programming possible. For example, in a distributed task processing system, Golang functional programming can be used to create closures to process tasks and execute tasks in parallel in a pool of workers, improving efficiency and scalability.
Application of Golang functions in distributed systems
Preface
In Functional programming is becoming increasingly important in modern distributed systems. Functional programming provides a set of tools for designing and developing scalable, maintainable, and performant systems. Golang is ideal for implementing functional programming as it provides powerful functional features and built-in support for concurrency.
Functional Programming Basics
Functional programming focuses on building software by breaking down problems into smaller, composable functions. These functions follow the following principles:
- Pure function: A function cannot modify its passed parameters or external state.
- No side effects: Functions should not produce any visible side effects, such as printing logs or creating files.
- First class citizen: Functions can be passed as parameters to other functions and can be returned as return values.
Functional Programming in Golang
Golang provides several features that make functional programming possible:
- Anonymous functions: Allows the creation of functions at runtime.
- Closure: Enables a function to access external variables when the function was created.
- Higher-order functions: Functions can be passed as parameters to other functions.
Practical Case: Distributed Task Processing
Let us consider an example of distributed task processing. We have a system that receives tasks and assigns them to a pool of distributed workers. To improve efficiency, we want to process tasks in parallel.
We can use Golang functional programming to implement this task processing system:
// Task represents a unit of work to be processed. type Task struct { // Input data for the task. Data []byte } // TaskProcessor represents a function that processes a task. type TaskProcessor func(t Task) error // TaskQueue is a queue of tasks to be processed. type TaskQueue chan Task // CreateTaskProcessor creates a task processor function. func CreateTaskProcessor(workerPoolSize int) TaskProcessor { // Create a pool of workers. workers := make([]worker, workerPoolSize) for i := 0; i < workerPoolSize; i++ { workers[i] = worker{ taskQueue: make(TaskQueue), } } // Start the workers. for _, w := range workers { go w.run() } // Return the task processor function. return func(t Task) error { // Send the task to a random worker. workers[rand.Intn(len(workers))].taskQueue <- t return nil } } // Worker represents a task processing worker. type worker struct { taskQueue TaskQueue } // run starts the worker and processes tasks. func (w *worker) run() { for t := range w.taskQueue { // Process the task. if err := processTask(t); err != nil { // Handle error. } } }
In the above example, we created the following functional component:
-
CreateTaskProcessor
Creates a closure that returns a task processing function. -
worker
is a worker that receives tasks and processes them.
By combining these functional components, we build a distributed task processing system that can process tasks in parallel, thereby improving efficiency and scalability.
The above is the detailed content of Application of golang functions in distributed systems. For more information, please follow other related articles on the PHP Chinese website!
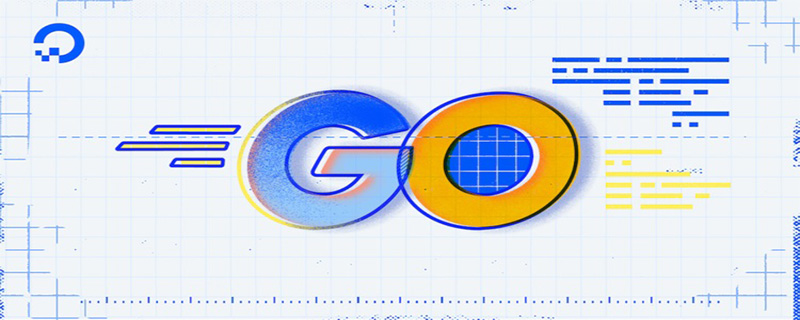
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
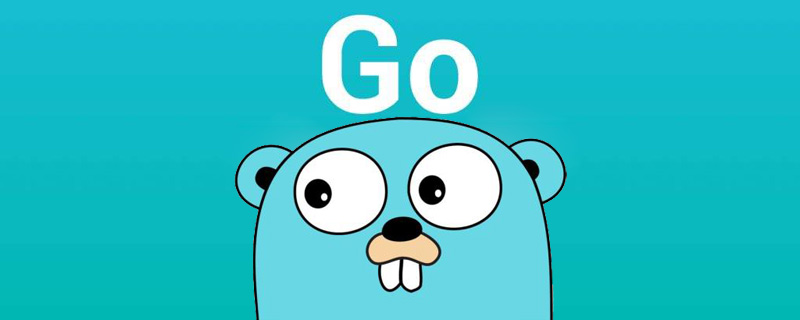
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
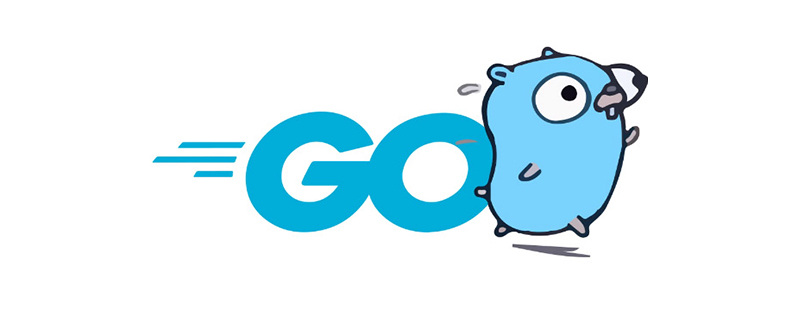
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
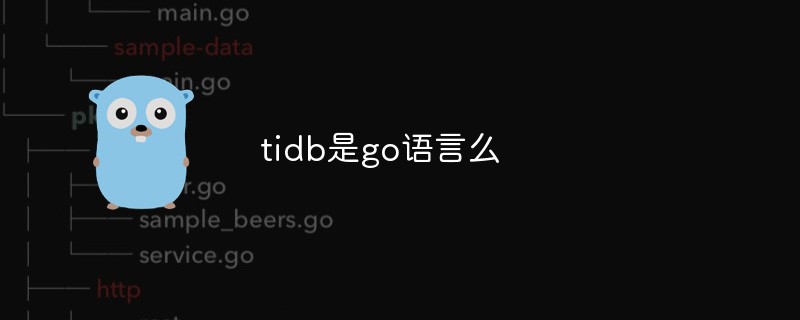
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
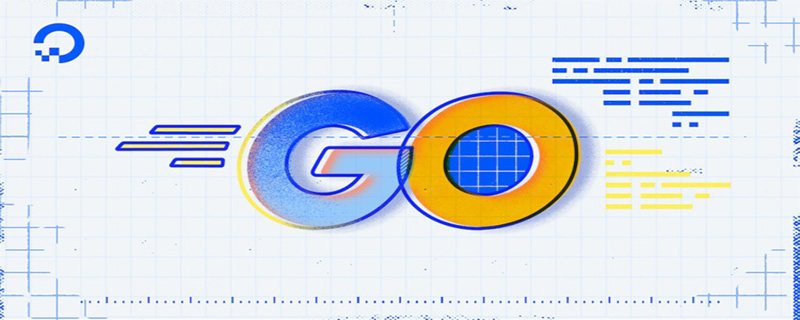
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
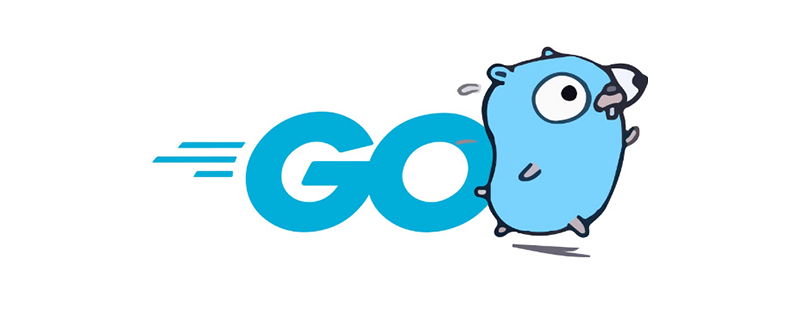
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
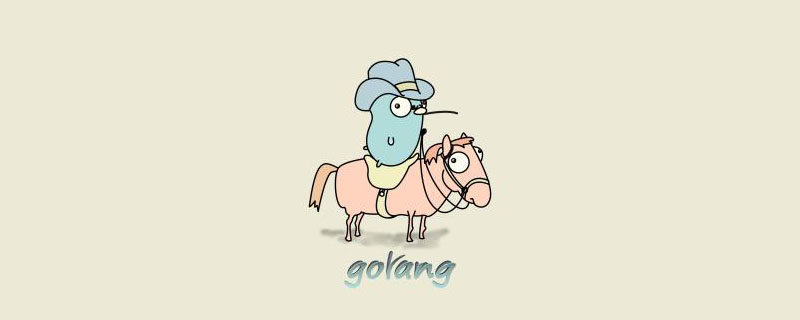
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
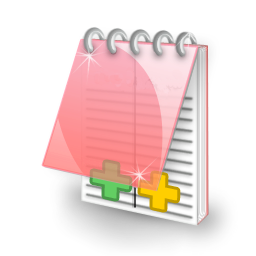
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
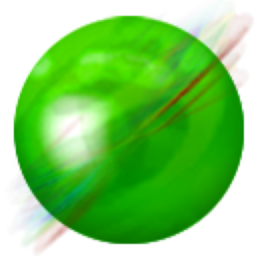
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
