


What is the type deduction mechanism for lambda expressions in C++ functions?
When a lambda expression captures a variable from an enclosing scope, the return type is deduced to the type of the captured variable. If multiple variables are captured, the return type is jointly deduced from their types. This mechanism allows the return type of a lambda expression to be deduced and automatically handles different types of containers when needed.
Type derivation mechanism of lambda expression in C function
Lambda expression is a convenient way to define anonymous functions in C method. This expression allows type deduction within a function for its return type.
Type deduction mechanism
When a lambda expression captures a variable from its enclosing scope, the deduced return type will be the same as the type of the captured variable. For example:
int main() { int x = 10; auto lambda = [x] { return x; }; int result = lambda(); }
In this example, the lambda expression captures the variable x
, so its return type is deduced to be int
, and it can be stored in int
variable.
If multiple variables are captured
If a lambda expression captures multiple variables, its return type will be jointly deduced from the types of the captured variables. For example:
struct Point { int x; int y; }; int main() { Point point = {1, 2}; auto lambda = [point] { return point.x + point.y; }; int result = lambda(); }
In this example, the lambda expression captures an instance point
of the structure Point
, so its return type is deduced to be int
and can be stored in a int
variable.
Practical Case
The following is a good practical case showing the type derivation of lambda expressions:
#include <iostream> #include <vector> template <typename T> void print_vector(const std::vector<T>& v) { for (auto& element : v) { std::cout << element << " "; } std::cout << std::endl; } int main() { std::vector<int> v1 = {1, 2, 3}; std::vector<double> v2 = {1.5, 2.5, 3.5}; print_vector(v1); print_vector(v2); }
In this example, # The ##print_vector function uses lambda expressions to deduce the
T type and automatically handles different types of containers. This function will type infer the correct return type and allow printing out the contents of the container of different types.
The above is the detailed content of What is the type deduction mechanism for lambda expressions in C++ functions?. For more information, please follow other related articles on the PHP Chinese website!
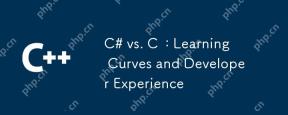
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
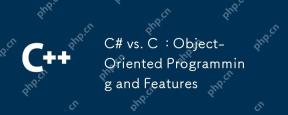
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
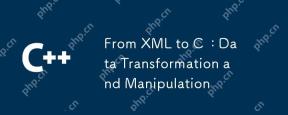
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
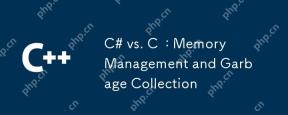
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
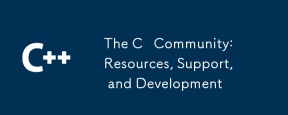
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
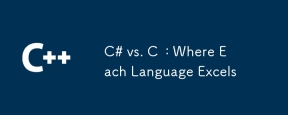
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
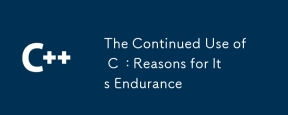
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
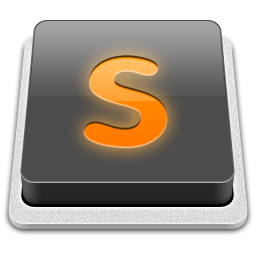
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use