What are some performance optimization tips for using Java functions?
Key techniques to improve Java function performance include: hot spot analysis, data structure selection, algorithm optimization, reducing function calls, concurrent programming and code reuse. By leveraging these techniques, such as using more efficient algorithms or inlining frequently called functions, you can significantly improve the efficiency of your Java functions.
Tips for improving performance with Java functions
Optimizing the performance of Java functions is critical to ensuring the smooth running of your application. The following are some tips to improve the efficiency of Java functions:
1. Hotspot analysis:
-
Use tools such as Java Profiler to identify areas that consume a lot of CPU time or Function part of memory.
import java.util.Arrays; public class Hotspots { public static void main(String[] args) { // 数组填充 int[] arr = new int[100000]; Arrays.fill(arr, 1); // 冒泡排序 long startTime = System.nanoTime(); for (int i = 0; i < arr.length - 1; i++) { for (int j = 0; j < arr.length - i - 1; j++) { if (arr[j] > arr[j + 1]) { int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } long endTime = System.nanoTime(); // 打印排序后的数组 for (int i : arr) { System.out.println(i); } // 打印执行时间 System.out.println("Execution time: " + (endTime - startTime) + " ns"); } }
2. Data structure selection:
- Choose appropriate collection and mapping data structures to optimize lookup and insertion operations.
- For example, for scenarios where elements need to be inserted and deleted quickly, you can consider using a linked list or a hash table.
3. Algorithm optimization:
- Use more effective algorithms to solve problems.
- For example, use binary search instead of linear search to quickly locate elements in an array.
- In the sorting algorithm, you can use divide-and-conquer algorithms such as quick sort or merge sort to improve efficiency.
4. Reduce function calls:
- Consider inlining frequently called functions to reduce the overhead of function calls.
- For example, if a function needs to generate a string multiple times, the operation can be inlined into the function body instead of calling the string generation function repeatedly.
5. Concurrent programming:
- Use multi-threading or asynchronous programming technology to execute tasks in parallel.
- For example, you can use the Fork/Join framework to parallelize computationally intensive tasks.
6. Code reuse:
- Write reusable modular code to avoid writing the same functionality repeatedly.
- For example, you can create utility classes that contain common utility functions.
Practical case:
Consider the following Java function that uses bubble sort to sort a large array:
public static void bubbleSort(int[] arr) { // 冒泡排序 for (int i = 0; i < arr.length - 1; i++) { for (int j = 0; j < arr.length - i - 1; j++) { if (arr[j] > arr[j + 1]) { int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } }
We can Use the above tips to optimize this function:
- Choose a better sorting algorithm: Bubble sort is inefficient, we can use quick sort or merge sort.
- Reduce function calls: Inline element swap logic to avoid function calls.
The optimized code is as follows:
public static void optimizedBubbleSort(int[] arr) { // 优化后的冒泡排序 for (int i = 0; i < arr.length - 1; i++) { for (int j = 0; j < arr.length - i - 1; j++) { if (arr[j] > arr[j + 1]) { arr[j] ^= arr[j + 1]; arr[j + 1] ^= arr[j]; arr[j] ^= arr[j + 1]; } } } }
The above is the detailed content of What are some performance optimization tips for using Java functions?. For more information, please follow other related articles on the PHP Chinese website!
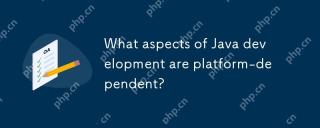
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
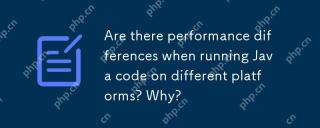
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
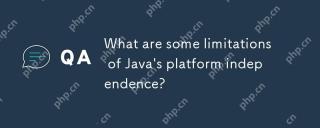
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
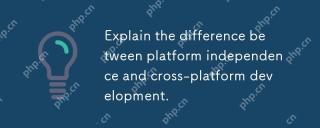
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
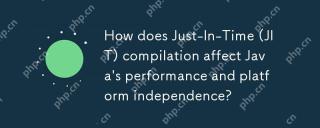
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
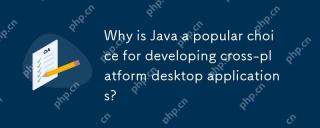
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
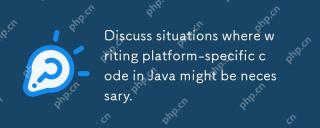
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
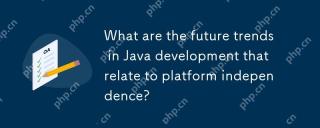
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
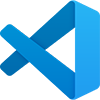
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
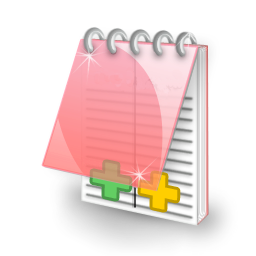
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
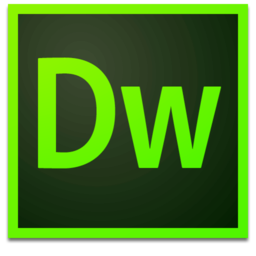
Dreamweaver Mac version
Visual web development tools
