Key elements of the C function style guide include: Function signatures: Use descriptive function names, easy-to-understand parameter types, and const references to pass non-modifying values. Function body: Break it into smaller pieces, use indentation and braces to enhance readability, and put variables in scope. Error handling: Use a try-catch block to specify a specific exception type and throw the exception. Memory management: Use smart pointers to avoid manual memory management. Documentation: Include purpose, parameter descriptions, and return values, and use documentation generation tools to automate the documentation process.
Elements of a C Functional Style Guide
Introduction
Normal functional style is essential for maintainability Safety and readability are crucial. This article outlines the key elements of the C functional style guide and provides practical examples to illustrate best practices.
Function signature
- Use descriptive and concise function names.
- Parameter types should be easy to understand and have clear names.
- Use const references to pass non-modifiable values.
- For longer parameter lists, use named parameters or structure parameters.
Practical case:
int calculate_total_cost(const Product& product, int quantity);
Function body
- Decompose the function body into manageable Small pieces.
- Use indentation and whitespace characters to enhance readability.
- Avoid declaring variables within the function body, instead place them within the scope.
- When using
if-else
statements, use indentation and braces.
Practical case:
if (is_valid) { // 执行有效代码 } else { // 执行无效代码 }
Error handling
- Use try-catch block to handle exceptions .
- Specify specific exception types in the catch block.
- Throw an exception when an error occurs instead of returning an error code.
Practical case:
try { // 执行可能抛出异常的操作 } catch (std::runtime_error& ex) { std::cerr << ex.what() << std::endl; }
Memory management
- Use smart pointers (such as std:: unique_ptr, std::shared_ptr) to avoid manual memory management.
- Explicitly release dynamically allocated memory in the function body.
Practical case:
void release_resource() { if (resource != nullptr) { delete resource; resource = nullptr; } }
Documentation
- Add comments to the function, including purpose and parameters Description and return value.
- Automate the documentation process using Doxygen or other documentation generation tools.
Practical case:
// 函数:计算产品总成本 /// /// \param product 要计算成本的产品 /// \param quantity 要购买的产品数量 /// \return 产品的总成本 int calculate_total_cost(const Product& product, int quantity);
Conclusion
Following the elements of these functional style guides can improve the maintainability of C code and readability are crucial. By applying these best practices, you can create high-quality functions that are easy to understand and maintain.
The above is the detailed content of Elements of a C++ Functional Style Guide. For more information, please follow other related articles on the PHP Chinese website!
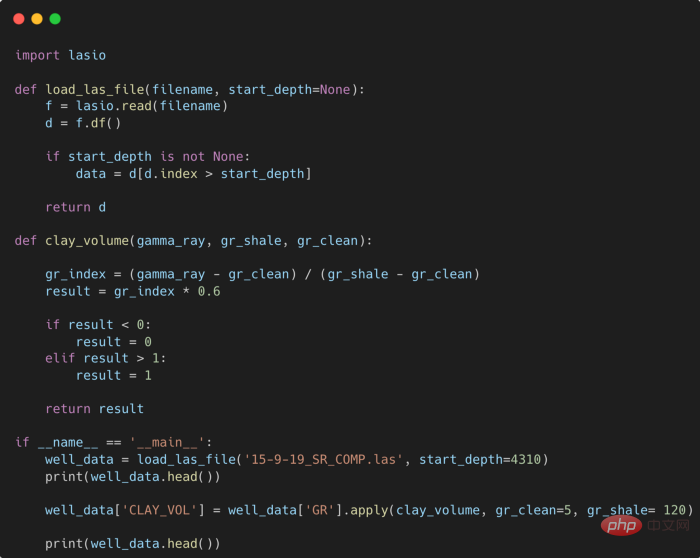
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
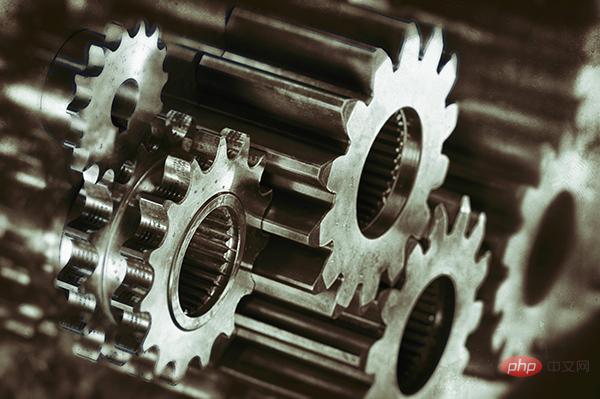
连续分级概率评分(Continuous Ranked Probability Score, CRPS)或“连续概率排位分数”是一个函数或统计量,可以将分布预测与真实值进行比较。机器学习工作流程的一个重要部分是模型评估。这个过程本身可以被认为是常识:将数据分成训练集和测试集,在训练集上训练模型,并使用评分函数评估其在测试集上的性能。评分函数(或度量)是将真实值及其预测映射到一个单一且可比较的值 [1]。例如,对于连续预测可以使用 RMSE、MAE、MAPE 或 R 平方等评分函数。如果预测不是逐点
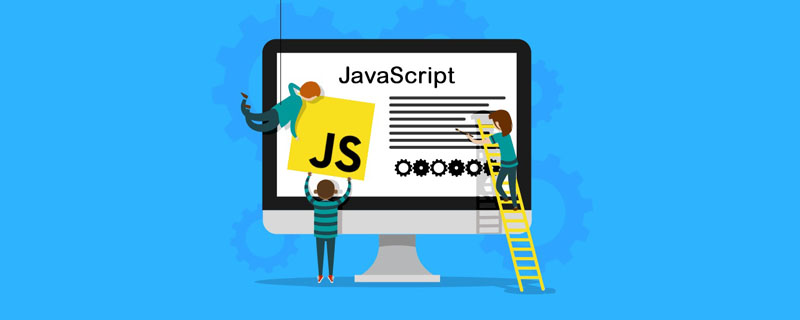
js是弱类型语言,不能像C#那样使用param关键字来声明形参是一个可变参数。那么js中,如何实现这种可变参数呢?下面本篇文章就来聊聊JavaScript函数可变参数的实现方法,希望对大家有所帮助!
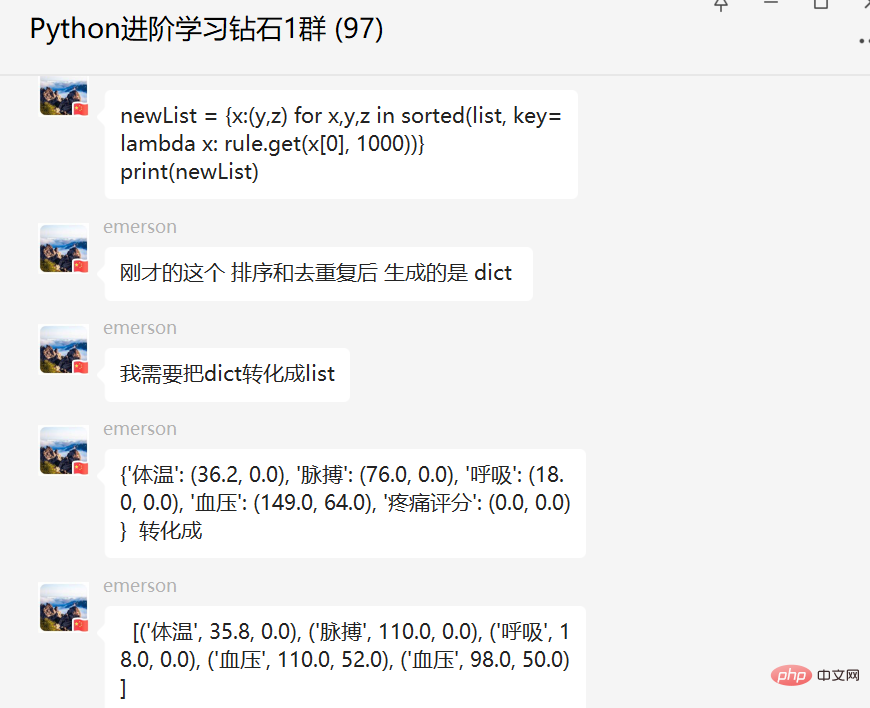
一、前言前几天在Python钻石交流群有个叫【emerson】的粉丝问了一个Python排序的问题,这里拿出来给大家分享下,一起学习下。其实这里【瑜亮老师】、【布达佩斯的永恒】等人讲了很多,只不过对于基础不太好的小伙伴们来说,还是有点难的。不过在实际应用中内置函数sorted()用的还是蛮多的,这里也单独拿出来讲一下,希望下次再有小伙伴遇到的时候,可以不慌。二、基础用法内置函数sorted()可以用来做排序,基础的用法很简单,看个例子,如下所示。lst=[3,28,18,29,2,5,88
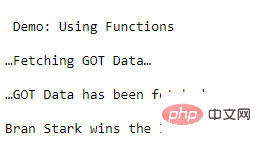
Python 中的 main 函数充当程序的执行点,在 Python 编程中定义 main 函数是启动程序执行的必要条件,不过它仅在程序直接运行时才执行,而在作为模块导入时不会执行。要了解有关 Python main 函数的更多信息,我们将从如下几点逐步学习:什么是 Python 函数Python 中 main 函数的功能是什么一个基本的 Python main() 是怎样的Python 执行模式Let’s get started什么是 Python 函数相信很多小伙伴对函数都不陌生了,函数是可

好嘞,今天我们继续剖析下Python里的类。[[441842]]先前我们定义类的时候,使用到了构造函数,在Python里的构造函数书写比较特殊,他是一个特殊的函数__init__,其实在类里,除了构造函数还有很多其他格式为__XXX__的函数,另外也有一些__xx__的属性。下面我们一一说下:构造函数Python里所有类的构造函数都是__init__,其中根据我们的需求,构造函数又分为有参构造函数和无惨构造函数。如果当前没有定义构造函数,那么系统会自动生成一个无参空的构造函数。例如:在有继承关系
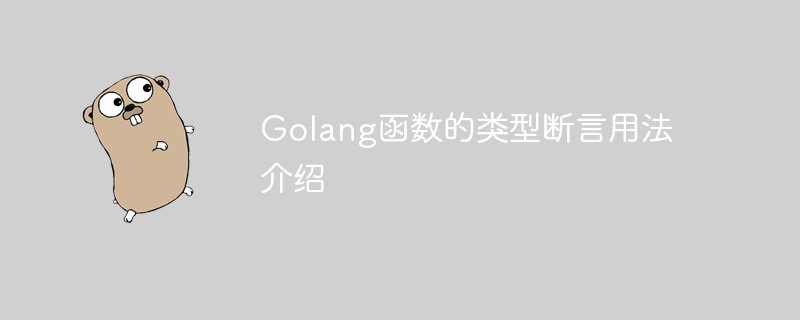
Golang的函数类型断言是一个非常重要的特性,它可以让我们在函数中精细地控制变量的类型,从而更加方便地进行数据处理和转换。本文将介绍Golang函数的类型断言用法,希望能够对大家的学习有所帮助。一、什么是Golang函数的类型断言?Golang函数的类型断言可以理解为函数参数中所声明变量的类型具有多态性,这使得一个函数在不同的参数传递下可以灵活
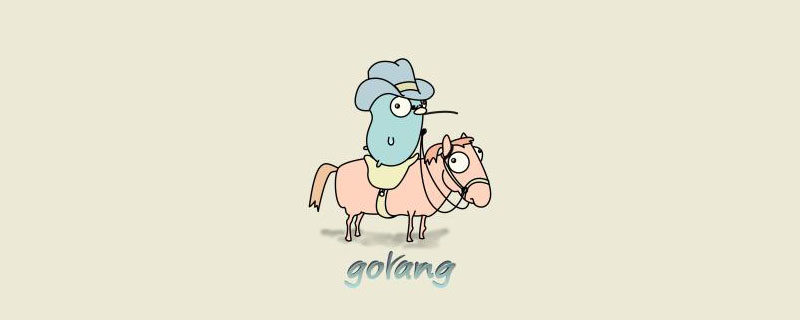
形参变量在未出现函数调用时并不占用内存,只在调用时才占用,调用结束后将释放内存。形参全称“形式参数”,是函数定义时使用的参数;但函数定义时参数是没有任实际何数据的,因而在函数被调用前没有为形参分配内存,其作用是说明自变量的类型和形态以及在过程中的作用。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
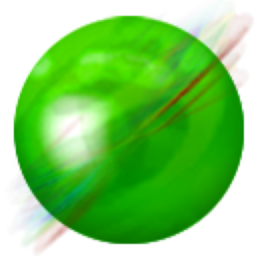
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
