


Concurrency control is crucial in distributed systems to ensure data consistency. Go provides a variety of concurrency control technologies, including: Goroutine: lightweight thread that allows concurrent execution of functions. Channel: A synchronization mechanism used for communication between coroutines. Mutex: A lock used to protect shared data from concurrent access. Condition variable: A synchronization mechanism used to wait for specific conditions to be met.
#The correlation between Go function concurrency control and distributed systems
In distributed systems, concurrency control is crucial to ensure data consistency. In the Go language, various techniques can be used to manage function concurrency, which is crucial for the efficient operation of distributed systems.
Concurrency control in Go
Go provides several primitives to manage concurrency, including:
- Coroutine (goroutine) : Lightweight threads that allow concurrent execution of functions.
- Channel (channel): Synchronization mechanism for communication between coroutines.
- Mutex lock (mutex): A lock used to protect shared data from concurrent access.
- Condition variable (condition variable): Synchronization mechanism used to wait for specific conditions to be met.
Distributed Systems and Concurrency Control
In distributed systems, concurrency control faces additional challenges, such as:
- Network Latency: Functions across different machines may need to wait for network delays, which affects concurrency.
- Distributed lock: Maintaining shared locks in a distributed system is very difficult.
- Distributed Data Consistency: It is crucial to ensure that data across multiple replicas remains consistent.
Practical Case
Consider the following example in a distributed system:
import ( "sync" "time" ) type Account struct { sync.Mutex balance float64 } func (a *Account) Withdraw(amount float64) { a.Lock() defer a.Unlock() if a.balance >= amount { a.balance -= amount } } func main() { account := &Account{balance: 100} go func() { for { account.Withdraw(50) time.Sleep(time.Millisecond * 50) } }() go func() { for { account.Withdraw(25) time.Sleep(time.Millisecond * 50) } }() <-time.After(time.Second * 5) fmt.Println(account.balance) }
In this example, two concurrent coroutines withdraw funds from the same account. Mutex locks are used to prevent simultaneous access to account balances, ensuring data consistency.
The above is the detailed content of The relationship between golang function concurrency control and distributed systems. For more information, please follow other related articles on the PHP Chinese website!
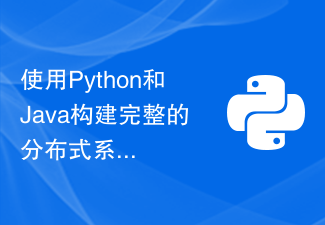
随着云计算和大数据技术的发展,分布式系统的应用越来越广泛,尤其是在企业级应用中。构建分布式系统可以提高系统的可伸缩性和容错性,使得系统更加稳定和可靠。在本文中,我们将介绍如何使用Python和Java构建一个完整的分布式系统。分布式系统通常由多个计算节点组成,这些节点可以是不同的计算机或者是运行在不同进程中的程序。这些节点之间通过通信协议进行通信,协同完成任
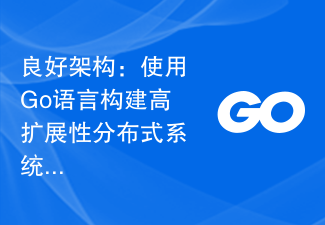
作为一款高性能的编程语言,Go语言在分布式系统的建设中非常流行。它的高速度和极低的延迟时间让开发人员更加容易实现高扩展性的分布式架构。在构建分布式系统前,需考虑的架构问题非常繁琐。如何设计出更加易于维护、可扩展和稳定的架构是所有分布式系统开发者面临的重要问题。使用Go语言来构建分布式系统,可以使这些架构选择变得更加简单和明晰。高效的协程Go语言天生支持协程,
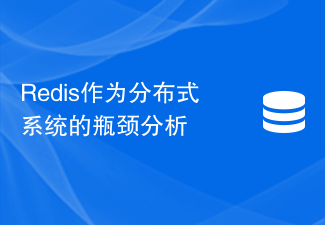
Redis作为一个开源的基于内存的键值存储系统,正被越来越多的企业使用于其分布式系统中,因为其高性能、可靠性和灵活性。但是,在一些情况下,Redis作为分布式系统中的瓶颈,可能会影响系统的整体性能。本文将探讨Redis在分布式系统中的瓶颈原因及其解决方法。Redis中的单线程模型Redis采用的是单线程模型,这意味着一个Redis实例只能够处理一条命令,即使
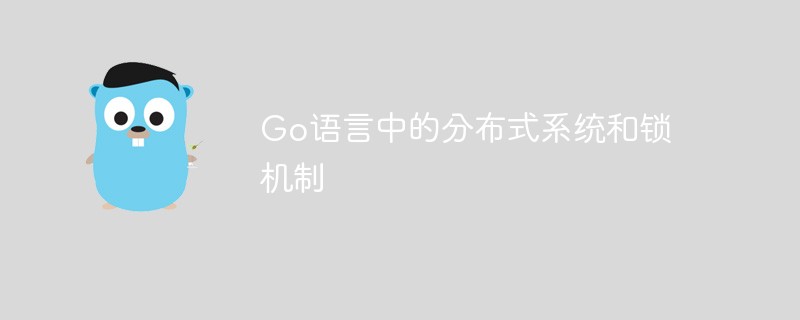
随着互联网的不断发展,分布式系统已经成为了应用领域中的热点话题之一。在分布式系统中,锁机制是一个重要的问题,特别是在涉及到并发的应用场景中,锁机制的效率和正确性越来越受到人们的重视。在这篇文章中,我们将介绍Go语言中的分布式系统和锁机制。分布式系统Go语言是一种开源的、现代的编程语言,具有高效、简洁、易于学习和使用等特点,在工程师团队中已经得到了广泛的应用和
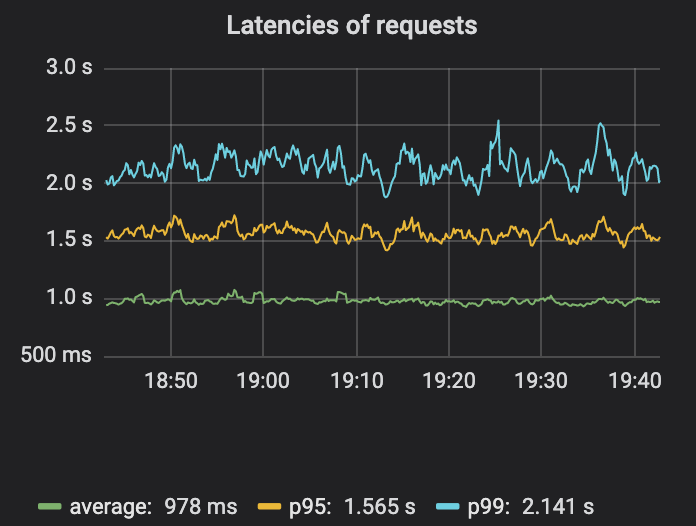
本文是Uber的工程师GergelyOrosz的文章,原文地址在:https://blog.pragmaticengineer.com/operating-a-high-scale-distributed-system/在过去的几年里,我一直在构建和运营一个大型分布式系统:优步的支付系统。在此期间,我学到了很多关于分布式架构概念的知识,并亲眼目睹了高负载和高可用性系统运行的挑战(一个系统远远不是开发完了就完了,线上运行的挑战实际更大)。构建系统本身是一项有趣的工作。规划系统如何处理10x/100
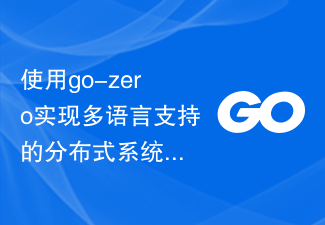
在当今全球化的时代,多语言支持的分布式系统已经成为许多企业的必要需求。为了实现多语言支持,开发人员需要在系统中处理不同的语言翻译和本地化问题。但是,很多人往往会遇到一系列的挑战,如何管理海量的本地化内容,如何快速切换语言、如何高效地管理翻译人员等等。这些问题对于开发系统来说非常具有挑战性和复杂性。在这样的情况下,使用go-zero这个高性能微服务框架来搭建多
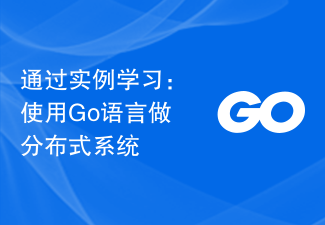
在当今互联网高速发展的背景下,分布式系统已经成为了大型企业和组织中不可或缺的一部分。而作为一门高效、强大且易于理解的编程语言,Go语言已经成为了开发分布式系统的首选语言之一。在本文中,我们将通过实例学习如何使用Go语言开发分布式系统。第一步:理解分布式系统在深入学习Go语言之前,我们需要理解什么是分布式系统。简单来说,分布式系统是由多个独立的计算机节点组成,
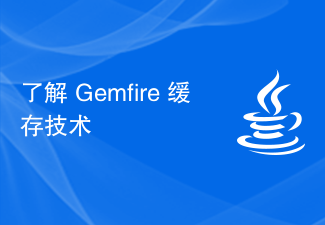
Gemfire是一个高性能的分布式内存数据管理平台,它是一种基于缓存的数据平台,可以提供实时的数据访问和响应,同时还有非常好的可扩展性和容错性。Gemfire提供了一种快速简便的方式从多个数据源获取数据,并将它们保存在一个严密的、可控制的缓存中,以此提高系统性能和可靠性。Gemfire的核心概念:Gemfire是基于一个类似于内存缓存的数据网格的概念


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
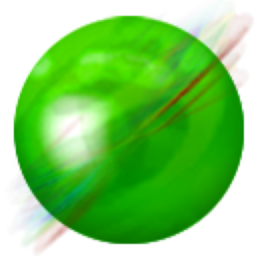
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
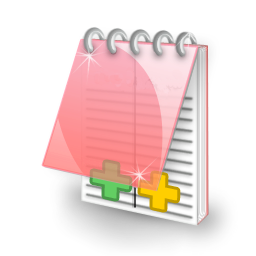
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
