PHP function efficiency improvement: avoid unnecessary copying or calculations; use local variables instead of passing parameters; cache expensive operations. Practical case: String processing function optimization: use string buffer; use preg_replace instead of str_replace; avoid unnecessary string conversion.
PHP function efficiency improvement: principles and applications
Principles of optimizing function calls
1. Avoid unnecessary copying or calculation
Do not repeatedly calculate or copy variable values inside the function. For example:
function calculate($a, $b) { $sum = $a + $b; $product = $a * $b; return $sum + $product; }
Improvement:
function calculate($a, $b) { $sum = $a + $b; return $sum + ($a * $b); }
2. Use local variables instead of passing parameters
When the passed parameters are used inside the function, PHP will copy them . Therefore, declare frequently accessed parameters as local variables to avoid extra copies:
function myFunction($input) { $result = ''; for ($i = 0; $i < count($input); $i++) { $result .= $input[$i]; } return $result; }
Improvement:
function myFunction($input) { $count = count($input); $result = ''; for ($i = 0; $i < $count; $i++) { $result .= $input[$i]; } return $result; }
3. Cache expensive operations
if function Perform expensive operations, such as database queries or complex calculations, and cache the results to avoid repeating those operations.
function getFromDB($id) { static $cache = []; if (!isset($cache[$id])) { $cache[$id] = queryDB($id); } return $cache[$id]; }
Practical case: Improving the efficiency of string processing functions
1. Using string buffer
PHP’s string buffer Provides faster string processing than string concatenation. The following is an example of using a string buffer:
$string = 'Hello'; $string .= ' World'; // 字符串拼接 $buffer = new StringWriter(); $buffer->write('Hello'); $buffer->write(' World'); // 字符串缓冲区 $string = $buffer->toString();
2. Use preg_replace
instead of str_replace
preg_replace
Faster than str_replace
for more complex replacements. The following is an example of preg_replace
:
$string = preg_replace('/<br>/', "\n", $string); // `preg_replace` $string = str_replace('<br>', "\n", $string); // `str_replace`
3. Avoid unnecessary string conversions
Use numbers or booleans directly as strings instead Convert it to a string first:
echo 'Value: ' . 123; // 直接使用数字 echo 'Value: ' . (string) 123; // 转换为字符串
The above is the detailed content of Improving PHP function efficiency: from principle to application. For more information, please follow other related articles on the PHP Chinese website!
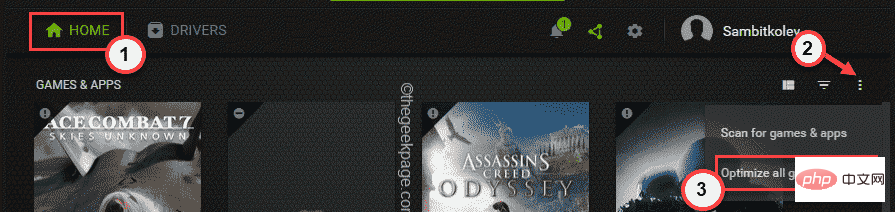
GeforceExperience不仅为您下载最新版本的游戏驱动程序,它还提供更多!最酷的事情之一是它可以根据您的系统规格优化您安装的所有游戏,为您提供最佳的游戏体验。但是一些游戏玩家报告了一个问题,即GeForceExperience没有优化他们系统上的游戏。只需执行这些简单的步骤即可在您的系统上解决此问题。修复1–为所有游戏使用最佳设置您可以设置为所有游戏使用最佳设置。1.在您的系统上打开GeForceExperience应用程序。2.GeForceExperience面
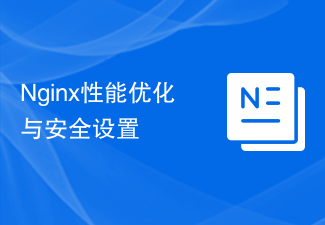
Nginx是一种常用的Web服务器,代理服务器和负载均衡器,性能优越,安全可靠,可以用于高负载的Web应用程序。在本文中,我们将探讨Nginx的性能优化和安全设置。一、性能优化调整worker_processes参数worker_processes是Nginx的一个重要参数。它指定了可以使用的worker进程数。这个值需要根据服务器硬件、网络带宽、负载类型等
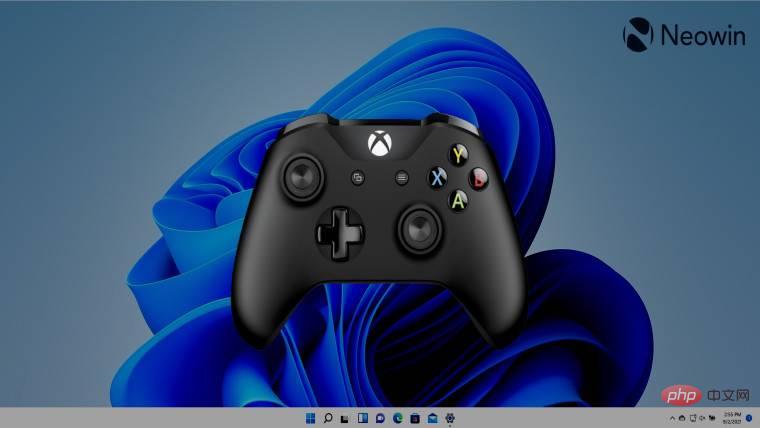
如果您在Windows机器上玩旧版游戏,您会很高兴知道Microsoft为它们计划了某些优化,特别是如果您在窗口模式下运行它们。该公司宣布,最近开发频道版本的内部人员现在可以利用这些功能。本质上,许多旧游戏使用“legacy-blt”演示模型在您的显示器上渲染帧。尽管DirectX12(DX12)已经利用了一种称为“翻转模型”的新演示模式,但Microsoft现在也正在向DX10和DX11游戏推出这一增强功能。迁移将改善延迟,还将为自动HDR和可变刷新率(VRR)等进一步增强打
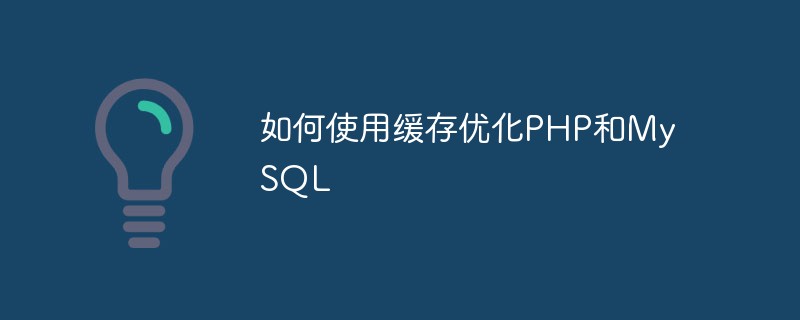
随着互联网的不断发展和应用的扩展,越来越多的网站和应用需要处理海量的数据和实现高流量的访问。在这种背景下,对于PHP和MySQL这样的常用技术,缓存优化成为了非常必要的优化手段。本文将在介绍缓存的概念及作用的基础上,从两个方面的PHP和MySQL进行缓存优化的实现,希望能够为广大开发者提供一些帮助。一、缓存的概念及作用缓存是指将计算结果或读取数据的结果缓存到
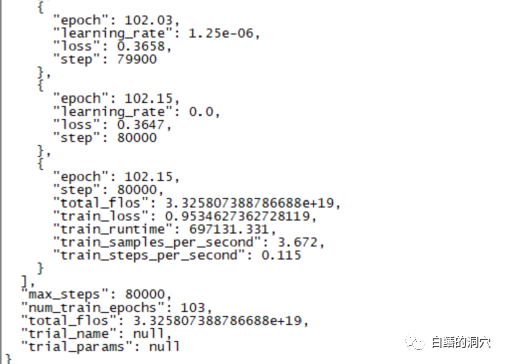
昨天一个跑了220个小时的微调训练完成了,主要任务是想在CHATGLM-6B上微调出一个能够较为精确的诊断数据库错误信息的对话模型来。不过这个等了将近十天的训练最后的结果令人失望,比起我之前做的一个样本覆盖更小的训练来,差的还是挺大的。这样的结果还是有点令人失望的,这个模型基本上是没有实用价值的。看样子需要重新调整参数与训练集,再做一次训练。大语言模型的训练是一场军备竞赛,没有好的装备是玩不起来的。看样子我们也必须要升级一下实验室的装备了,否则没有几个十天可以浪费。从最近的几次失败的微调训练来看
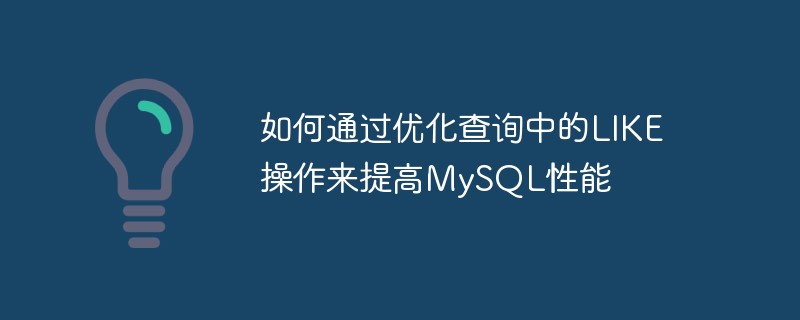
MySQL是目前最流行的关系型数据库之一,但是在处理大量数据时,MySQL的性能可能会受到影响。其中,一种常见的性能瓶颈是查询中的LIKE操作。在MySQL中,LIKE操作是用来模糊匹配字符串的,它可以在查询数据表时用来查找包含指定字符或者模式的数据记录。但是,在大型数据表中,如果使用LIKE操作,它会对数据库的性能造成影响。为了解决这个问题,我们可
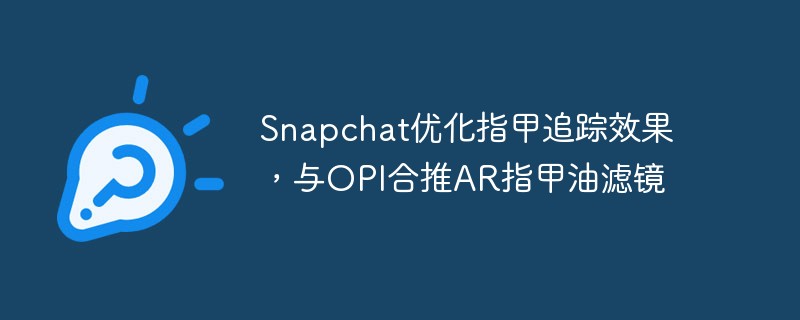
5月26日消息,SnapchatAR试穿滤镜技术升级,并与OPI品牌合作,推出指甲油AR试用滤镜。据悉,为了优化AR滤镜对手指甲的追踪定位,Snap在LensStudio中推出手部和指甲分割功能,允许开发者将AR图像叠加在指甲这种细节部分。据青亭网了解,指甲分割功能在识别到人手后,会给手部和指甲分别设置掩膜,用于渲染2D纹理。此外,还会识别用户个人指甲的底色,来模拟指甲油真实上手的效果。从演示效果来看,新的AR指甲油滤镜可以很好的模拟浅蓝磨砂质地。实际上,此前Snapchat曾推出AR指甲油试用
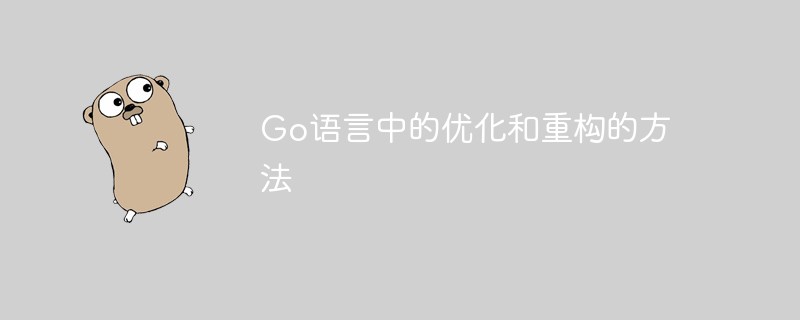
Go语言是一门相对年轻的编程语言,虽然从语言本身的设计来看,其已经考虑到了很多优化点,使得其具备高效的性能和良好的可维护性,但是这并不代表着我们在开发Go应用时不需要优化和重构,特别是在长期的代码积累过程中,原来的代码架构可能已经开始失去优势,需要通过优化和重构来提高系统的性能和可维护性。本文将分享一些在Go语言中优化和重构的方法,希望能够对Go开发者有所帮


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
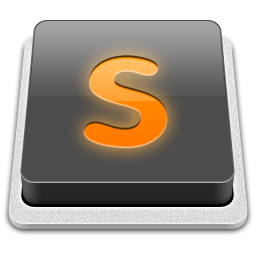
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
